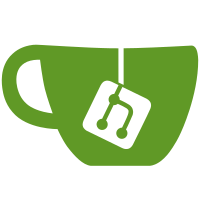
Respend transactions that conflict with transactions already in the wallet are added to it. They are not displayed unless they also involve the wallet, or get into a block. If they do not involve the wallet, they continue not to affect balance. Transactions that involve the wallet, and have conflicting non-equivalent transactions, are highlighted in red. When the conflict first occurs, a modal dialog is thrown. CWallet::SyncMetaData is changed to sync only to equivalent transactions. When a conflict is added to the wallet, counter nConflictsReceived is incremented. This acts like a change in active block height for the purpose of triggering UI updates.
103 lines
2.9 KiB
C++
103 lines
2.9 KiB
C++
// Copyright (c) 2011-2013 The Bitcoin developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include "transactionfilterproxy.h"
|
|
|
|
#include "transactiontablemodel.h"
|
|
#include "transactionrecord.h"
|
|
|
|
#include <cstdlib>
|
|
|
|
#include <QDateTime>
|
|
|
|
// Earliest date that can be represented (far in the past)
|
|
const QDateTime TransactionFilterProxy::MIN_DATE = QDateTime::fromTime_t(0);
|
|
// Last date that can be represented (far in the future)
|
|
const QDateTime TransactionFilterProxy::MAX_DATE = QDateTime::fromTime_t(0xFFFFFFFF);
|
|
|
|
TransactionFilterProxy::TransactionFilterProxy(QObject *parent) :
|
|
QSortFilterProxyModel(parent),
|
|
dateFrom(MIN_DATE),
|
|
dateTo(MAX_DATE),
|
|
addrPrefix(),
|
|
typeFilter(ALL_TYPES),
|
|
minAmount(0),
|
|
limitRows(-1),
|
|
showInactive(false)
|
|
{
|
|
}
|
|
|
|
bool TransactionFilterProxy::filterAcceptsRow(int sourceRow, const QModelIndex &sourceParent) const
|
|
{
|
|
QModelIndex index = sourceModel()->index(sourceRow, 0, sourceParent);
|
|
|
|
int type = index.data(TransactionTableModel::TypeRole).toInt();
|
|
QDateTime datetime = index.data(TransactionTableModel::DateRole).toDateTime();
|
|
QString address = index.data(TransactionTableModel::AddressRole).toString();
|
|
QString label = index.data(TransactionTableModel::LabelRole).toString();
|
|
qint64 amount = llabs(index.data(TransactionTableModel::AmountRole).toLongLong());
|
|
int status = index.data(TransactionTableModel::StatusRole).toInt();
|
|
|
|
if(!showInactive && status == TransactionStatus::Conflicted && type == TransactionRecord::Other)
|
|
return false;
|
|
if(!(TYPE(type) & typeFilter))
|
|
return false;
|
|
if(datetime < dateFrom || datetime > dateTo)
|
|
return false;
|
|
if (!address.contains(addrPrefix, Qt::CaseInsensitive) && !label.contains(addrPrefix, Qt::CaseInsensitive))
|
|
return false;
|
|
if(amount < minAmount)
|
|
return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
void TransactionFilterProxy::setDateRange(const QDateTime &from, const QDateTime &to)
|
|
{
|
|
this->dateFrom = from;
|
|
this->dateTo = to;
|
|
invalidateFilter();
|
|
}
|
|
|
|
void TransactionFilterProxy::setAddressPrefix(const QString &addrPrefix)
|
|
{
|
|
this->addrPrefix = addrPrefix;
|
|
invalidateFilter();
|
|
}
|
|
|
|
void TransactionFilterProxy::setTypeFilter(quint32 modes)
|
|
{
|
|
this->typeFilter = modes;
|
|
invalidateFilter();
|
|
}
|
|
|
|
void TransactionFilterProxy::setMinAmount(qint64 minimum)
|
|
{
|
|
this->minAmount = minimum;
|
|
invalidateFilter();
|
|
}
|
|
|
|
void TransactionFilterProxy::setLimit(int limit)
|
|
{
|
|
this->limitRows = limit;
|
|
}
|
|
|
|
void TransactionFilterProxy::setShowInactive(bool showInactive)
|
|
{
|
|
this->showInactive = showInactive;
|
|
invalidateFilter();
|
|
}
|
|
|
|
int TransactionFilterProxy::rowCount(const QModelIndex &parent) const
|
|
{
|
|
if(limitRows != -1)
|
|
{
|
|
return std::min(QSortFilterProxyModel::rowCount(parent), limitRows);
|
|
}
|
|
else
|
|
{
|
|
return QSortFilterProxyModel::rowCount(parent);
|
|
}
|
|
}
|