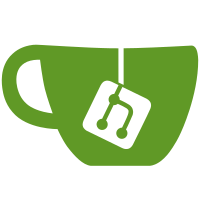
* add locktransaction rpc call * Remove special instantsend fee for simple transactions * Function to check if trx is simple enough to be autolocked * Automatic lock for all received from peers simple trxes If we get a new transaction with CInv message and it is "simple" and is accepted in mempool, we initiate its lock. We don't lock orphan trxes that accepted in mempool after this trx because they are locked by other peers. * Automatically lock simple trxes in wallet * protocol bump for InstantSend without special fee * Add function to detect used mempool share * Mempool threshold for auto IX locks * Add SPORK_16_INSTANTSEND_AUTOLOCKS spork * Make autolocks active only when spork SPORK_16_INSTANTSEND_AUTOLOCKS is active * BIP9 autolocks activation * revert increasing min peer protocol version for mn rank * move IsTrxSimple check to CTxLockRequest class * make MAX_INPUTS_FOR_AUTO_IX private member of CTxLockRequest class * make AUTO_IX_MEMPOOL_THRESHOLD private member of CInstantSend class * remove locktransaction RPC call * tests for automatic IS locks * fix mempool threshod calculation * bump mocktime in activate_autoix_bip9 * set node times * no need to spam the node with gettransaction rpc requests that often * use `spork active` instead of leaking spork logic into tests * codestyle fixes * add test description in comments * fix typo * sync test nodes more often during BIP9 activation * Use 4th bit in BIP9 activation * Fix comments according codestyle guide * Call AcceptLockRequest and Vote at the first node creating autoix lock * fix mempool used memory calculation * rallback not necessary change in CWallet::CreateTransaction * test for stopping autolocks for full mempool * Inject "simple autolockable" txes into txlockrequest logic
98 lines
3.8 KiB
C++
98 lines
3.8 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_CONSENSUS_PARAMS_H
|
|
#define BITCOIN_CONSENSUS_PARAMS_H
|
|
|
|
#include "uint256.h"
|
|
#include <map>
|
|
#include <string>
|
|
|
|
namespace Consensus {
|
|
|
|
enum DeploymentPos
|
|
{
|
|
DEPLOYMENT_TESTDUMMY,
|
|
DEPLOYMENT_CSV, // Deployment of BIP68, BIP112, and BIP113.
|
|
DEPLOYMENT_DIP0001, // Deployment of DIP0001 and lower transaction fees.
|
|
DEPLOYMENT_BIP147, // Deployment of BIP147 (NULLDUMMY)
|
|
DEPLOYMENT_DIP0003, // Deployment of DIP0002 and DIP0003 (txv3 and deterministic MN lists)
|
|
DEPLOYMENT_ISAUTOLOCKS, // Deployment of automatic IS locks for simple transactions
|
|
// NOTE: Also add new deployments to VersionBitsDeploymentInfo in versionbits.cpp
|
|
MAX_VERSION_BITS_DEPLOYMENTS
|
|
};
|
|
|
|
/**
|
|
* Struct for each individual consensus rule change using BIP9.
|
|
*/
|
|
struct BIP9Deployment {
|
|
/** Bit position to select the particular bit in nVersion. */
|
|
int bit;
|
|
/** Start MedianTime for version bits miner confirmation. Can be a date in the past */
|
|
int64_t nStartTime;
|
|
/** Timeout/expiry MedianTime for the deployment attempt. */
|
|
int64_t nTimeout;
|
|
/** The number of past blocks (including the block under consideration) to be taken into account for locking in a fork. */
|
|
int64_t nWindowSize;
|
|
/** A number of blocks, in the range of 1..nWindowSize, which must signal for a fork in order to lock it in. */
|
|
int64_t nThreshold;
|
|
};
|
|
|
|
/**
|
|
* Parameters that influence chain consensus.
|
|
*/
|
|
struct Params {
|
|
uint256 hashGenesisBlock;
|
|
uint256 hashDevnetGenesisBlock;
|
|
int nSubsidyHalvingInterval;
|
|
int nMasternodePaymentsStartBlock;
|
|
int nMasternodePaymentsIncreaseBlock;
|
|
int nMasternodePaymentsIncreasePeriod; // in blocks
|
|
int nInstantSendConfirmationsRequired; // in blocks
|
|
int nInstantSendKeepLock; // in blocks
|
|
int nBudgetPaymentsStartBlock;
|
|
int nBudgetPaymentsCycleBlocks;
|
|
int nBudgetPaymentsWindowBlocks;
|
|
int nSuperblockStartBlock;
|
|
uint256 nSuperblockStartHash;
|
|
int nSuperblockCycle; // in blocks
|
|
int nGovernanceMinQuorum; // Min absolute vote count to trigger an action
|
|
int nGovernanceFilterElements;
|
|
int nMasternodeMinimumConfirmations;
|
|
/** Block height and hash at which BIP34 becomes active */
|
|
int BIP34Height;
|
|
uint256 BIP34Hash;
|
|
/** Block height at which BIP65 becomes active */
|
|
int BIP65Height;
|
|
/** Block height at which BIP66 becomes active */
|
|
int BIP66Height;
|
|
/** Block height at which DIP0001 becomes active */
|
|
int DIP0001Height;
|
|
/**
|
|
* Minimum blocks including miner confirmation of the total of nMinerConfirmationWindow blocks in a retargeting period,
|
|
* (nPowTargetTimespan / nPowTargetSpacing) which is also used for BIP9 deployments.
|
|
* Default BIP9Deployment::nThreshold value for deployments where it's not specified and for unknown deployments.
|
|
* Examples: 1916 for 95%, 1512 for testchains.
|
|
*/
|
|
uint32_t nRuleChangeActivationThreshold;
|
|
// Default BIP9Deployment::nWindowSize value for deployments where it's not specified and for unknown deployments.
|
|
uint32_t nMinerConfirmationWindow;
|
|
BIP9Deployment vDeployments[MAX_VERSION_BITS_DEPLOYMENTS];
|
|
/** Proof of work parameters */
|
|
uint256 powLimit;
|
|
bool fPowAllowMinDifficultyBlocks;
|
|
bool fPowNoRetargeting;
|
|
int64_t nPowTargetSpacing;
|
|
int64_t nPowTargetTimespan;
|
|
int nPowKGWHeight;
|
|
int nPowDGWHeight;
|
|
int64_t DifficultyAdjustmentInterval() const { return nPowTargetTimespan / nPowTargetSpacing; }
|
|
uint256 nMinimumChainWork;
|
|
uint256 defaultAssumeValid;
|
|
};
|
|
} // namespace Consensus
|
|
|
|
#endif // BITCOIN_CONSENSUS_PARAMS_H
|