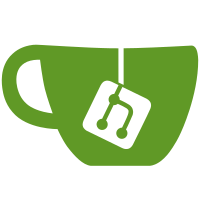
* standart STL containers and Masternode-related classes processing * masternode-related classes * fix st containers processing, use reflection to process simple classes * Content descrioption in README.md * Increase python scripts performance Use gdb objects instead strings and type info for creating Pyton wrappers * fixed simple classes list * addition to README * fix nits * missed `the` Co-Authored-By: gladcow <sergey@dash.org> * fix nits Co-Authored-By: gladcow <sergey@dash.org> * fixed grammatical issues Co-Authored-By: gladcow <sergey@dash.org> * fixed phrase construction Co-Authored-By: gladcow <sergey@dash.org> * missed point Co-Authored-By: gladcow <sergey@dash.org> * fixed grammatical issues Co-Authored-By: gladcow <sergey@dash.org> * remove double space Co-Authored-By: gladcow <sergey@dash.org> * fixed grammatical issues Co-Authored-By: gladcow <sergey@dash.org>
59 lines
2.0 KiB
Python
59 lines
2.0 KiB
Python
#!/usr/bin/python
|
|
#
|
|
|
|
try:
|
|
import gdb
|
|
except ImportError as e:
|
|
raise ImportError("This script must be run in GDB: ", str(e))
|
|
import sys
|
|
import os
|
|
sys.path.append(os.getcwd())
|
|
import common_helpers
|
|
|
|
|
|
simple_types = ["CMasternode", "CMasternodeVerification",
|
|
"CMasternodeBroadcast", "CMasternodePing",
|
|
"CMasternodeMan", "CDarksendQueue", "CDarkSendEntry",
|
|
"CTransaction", "CMutableTransaction", "CPrivateSendBaseSession",
|
|
"CPrivateSendBaseManager", "CPrivateSendClientSession",
|
|
"CPrivateSendClientManager", "CPrivateSendServer", "CMasternodePayments",
|
|
"CMasternodePaymentVote", "CMasternodeBlockPayees",
|
|
"CMasternodePayee", "CInstantSend", "CTxLockRequest",
|
|
"CTxLockVote", "CTxLockCandidate", "COutPoint",
|
|
"COutPointLock", "CSporkManager", "CMasternodeSync",
|
|
"CGovernanceManager", "CRateCheckBuffer", "CGovernanceObject",
|
|
"CGovernanceVote", "CGovernanceObjectVoteFile"]
|
|
|
|
simple_templates = ["CacheMultiMap", "CacheMap"]
|
|
|
|
|
|
class SimpleClassObj:
|
|
|
|
def __init__ (self, gobj):
|
|
self.obj = gobj
|
|
|
|
@classmethod
|
|
def is_this_type(cls, obj_type):
|
|
str_type = str(obj_type)
|
|
if str_type in simple_types:
|
|
return True
|
|
for templ in simple_templates:
|
|
if str_type.find(templ + "<") == 0:
|
|
return True
|
|
return False
|
|
|
|
def get_used_size(self):
|
|
size = 0
|
|
fields = self.obj.type.fields()
|
|
for f in fields:
|
|
# check if it is static field
|
|
if not hasattr(f, "bitpos"):
|
|
continue
|
|
# process base class size
|
|
if f.is_base_class:
|
|
size += common_helpers.get_instance_size(self.obj.cast(f.type.strip_typedefs()))
|
|
continue
|
|
# process simple field
|
|
size += common_helpers.get_instance_size(self.obj[f.name])
|
|
return size
|