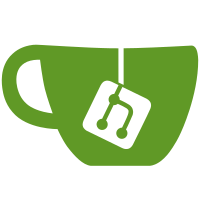
b6b6d6c Added nSuperblockStartBlock, adjusted testnet/regtest params 15a3c64 More for governance block checks, p1 (non-compilable): - add GetPaymentsLimit() and GetPaymentsTotalAmount() - IsValidBlockHeight() should check nSuperblockStartBlock - CSuperblock::IsValid should check payment limit and miner payout - no cs_main - slightly refactored related things e8f9e5d More for governance checks, p2 (compilable): - IsBlockValueValid(), IsBlockPayeeValid() and FillBlockPayee() rewritten, no cs_main for them - CreateNewBlock adjusted, need more work on CBlockTemplate (see TODO) - moved (and simplified) IsBlockPayeeValid() call from CheckBlock() to ConnectBlock() 51434cf Add ability to calculate only superblock part of subsidy in GetBlockSubsidy() aa74200 Fix GetPaymentsLimit() f7b6234 braces and comment ade8f64 more checks for IsValidBlockHeight()
78 lines
2.5 KiB
C++
78 lines
2.5 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_CONSENSUS_PARAMS_H
|
|
#define BITCOIN_CONSENSUS_PARAMS_H
|
|
|
|
#include "uint256.h"
|
|
#include <map>
|
|
#include <string>
|
|
|
|
namespace Consensus {
|
|
|
|
enum DeploymentPos
|
|
{
|
|
DEPLOYMENT_TESTDUMMY,
|
|
DEPLOYMENT_CSV, // Deployment of BIP68, BIP112, and BIP113.
|
|
MAX_VERSION_BITS_DEPLOYMENTS
|
|
};
|
|
|
|
/**
|
|
* Struct for each individual consensus rule change using BIP9.
|
|
*/
|
|
struct BIP9Deployment {
|
|
/** Bit position to select the particular bit in nVersion. */
|
|
int bit;
|
|
/** Start MedianTime for version bits miner confirmation. Can be a date in the past */
|
|
int64_t nStartTime;
|
|
/** Timeout/expiry MedianTime for the deployment attempt. */
|
|
int64_t nTimeout;
|
|
};
|
|
|
|
/**
|
|
* Parameters that influence chain consensus.
|
|
*/
|
|
struct Params {
|
|
uint256 hashGenesisBlock;
|
|
int nSubsidyHalvingInterval;
|
|
int nMasternodePaymentsStartBlock;
|
|
int nMasternodePaymentsIncreaseBlock;
|
|
int nMasternodePaymentsIncreasePeriod; // in blocks
|
|
int nInstantSendKeepLock; // in blocks
|
|
int nInstantSendReprocessBlocks;
|
|
int nBudgetPaymentsStartBlock;
|
|
int nBudgetPaymentsCycleBlocks;
|
|
int nBudgetPaymentsWindowBlocks;
|
|
int nBudgetProposalEstablishingTime; // in seconds
|
|
int nSuperblockStartBlock;
|
|
int nSuperblockCycle; // in blocks
|
|
int nMasternodeMinimumConfirmations;
|
|
/** Used to check majorities for block version upgrade */
|
|
int nMajorityEnforceBlockUpgrade;
|
|
int nMajorityRejectBlockOutdated;
|
|
int nMajorityWindow;
|
|
/** Block height and hash at which BIP34 becomes active */
|
|
int BIP34Height;
|
|
uint256 BIP34Hash;
|
|
/**
|
|
* Minimum blocks including miner confirmation of the total of 2016 blocks in a retargetting period,
|
|
* (nPowTargetTimespan / nPowTargetSpacing) which is also used for BIP9 deployments.
|
|
* Examples: 1916 for 95%, 1512 for testchains.
|
|
*/
|
|
uint32_t nRuleChangeActivationThreshold;
|
|
uint32_t nMinerConfirmationWindow;
|
|
BIP9Deployment vDeployments[MAX_VERSION_BITS_DEPLOYMENTS];
|
|
/** Proof of work parameters */
|
|
uint256 powLimit;
|
|
bool fPowAllowMinDifficultyBlocks;
|
|
bool fPowNoRetargeting;
|
|
int64_t nPowTargetSpacing;
|
|
int64_t nPowTargetTimespan;
|
|
int64_t DifficultyAdjustmentInterval() const { return nPowTargetTimespan / nPowTargetSpacing; }
|
|
};
|
|
} // namespace Consensus
|
|
|
|
#endif // BITCOIN_CONSENSUS_PARAMS_H
|