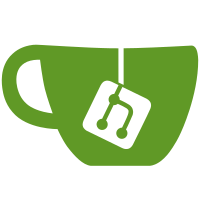
1c860ce Autobackup refactoring and improvements: - make nWalletBackups globally accessable - move autobackup code from init.cpp to walletdb.cpp, see AutoBackupWallet function - refactor autobackup code to warn user if autobackup failed instead of silently ignoring this fact - refactor autobackup code to be able to backup fresh new wallet right after it was created, add this functionality to init sequence - add new cmd-line option "-walletbackupsdir" to specify full path to directory for automatic wallet backups, see GetBackupsDir function 0ba1548 autobackup in PS: - add nKeysLeftSinceAutoBackup to have some idea how many keys in keypool are more or less safe, show it in advanced PS UI mode and in rpc output for privatesend and getwalletinfo commands - add autobackups support in PrivateSend mixing both in daemon and QT mode, warn user if number of keys left since last autobackup is very low or even stop mixing completely if it's too low f3a2494 Warn about a special case - less than 60 seconds between restarts i.e. backup file name is the same as previos one. Continue and do not disable automatic backups in this case . e7b56bd Refactor to address locked wallets issue, replenish keypool and re-initialize autobackup on unlock (only if was disabled due to keypool issue) Adjust few message strings.
81 lines
2.2 KiB
C++
81 lines
2.2 KiB
C++
// Copyright (c) 2011-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_QT_OVERVIEWPAGE_H
|
|
#define BITCOIN_QT_OVERVIEWPAGE_H
|
|
|
|
#include "amount.h"
|
|
|
|
#include <QWidget>
|
|
|
|
class ClientModel;
|
|
class TransactionFilterProxy;
|
|
class TxViewDelegate;
|
|
class PlatformStyle;
|
|
class WalletModel;
|
|
|
|
namespace Ui {
|
|
class OverviewPage;
|
|
}
|
|
|
|
QT_BEGIN_NAMESPACE
|
|
class QModelIndex;
|
|
QT_END_NAMESPACE
|
|
|
|
/** Overview ("home") page widget */
|
|
class OverviewPage : public QWidget
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit OverviewPage(const PlatformStyle *platformStyle, QWidget *parent = 0);
|
|
~OverviewPage();
|
|
|
|
void setClientModel(ClientModel *clientModel);
|
|
void setWalletModel(WalletModel *walletModel);
|
|
void showOutOfSyncWarning(bool fShow);
|
|
|
|
public Q_SLOTS:
|
|
void privateSendStatus();
|
|
void setBalance(const CAmount& balance, const CAmount& unconfirmedBalance, const CAmount& immatureBalance, const CAmount& anonymizedBalance,
|
|
const CAmount& watchOnlyBalance, const CAmount& watchUnconfBalance, const CAmount& watchImmatureBalance);
|
|
|
|
Q_SIGNALS:
|
|
void transactionClicked(const QModelIndex &index);
|
|
|
|
private:
|
|
QTimer *timer;
|
|
Ui::OverviewPage *ui;
|
|
ClientModel *clientModel;
|
|
WalletModel *walletModel;
|
|
CAmount currentBalance;
|
|
CAmount currentUnconfirmedBalance;
|
|
CAmount currentImmatureBalance;
|
|
CAmount currentAnonymizedBalance;
|
|
CAmount currentWatchOnlyBalance;
|
|
CAmount currentWatchUnconfBalance;
|
|
CAmount currentWatchImmatureBalance;
|
|
int nDisplayUnit;
|
|
bool fShowAdvancedPSUI;
|
|
|
|
TxViewDelegate *txdelegate;
|
|
TransactionFilterProxy *filter;
|
|
|
|
void SetupTransactionList(int nNumItems);
|
|
void DisablePrivateSendCompletely();
|
|
|
|
private Q_SLOTS:
|
|
void togglePrivateSend();
|
|
void privateSendAuto();
|
|
void privateSendReset();
|
|
void updateDisplayUnit();
|
|
void updatePrivateSendProgress();
|
|
void updateAdvancedPSUI(bool fShowAdvancedPSUI);
|
|
void handleTransactionClicked(const QModelIndex &index);
|
|
void updateAlerts(const QString &warnings);
|
|
void updateWatchOnlyLabels(bool showWatchOnly);
|
|
};
|
|
|
|
#endif // BITCOIN_QT_OVERVIEWPAGE_H
|