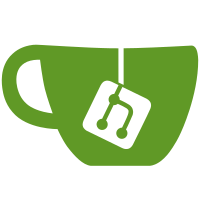
All direct modifications are now done through ModifyCoins, and BatchWrite is used for pushing batches of queued modifications up, so we don't need the low-level SetCoins and SetBestBlock anymore in the top-level CCoinsView class.
66 lines
2.1 KiB
C++
66 lines
2.1 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2013 The Bitcoin developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_TXDB_LEVELDB_H
|
|
#define BITCOIN_TXDB_LEVELDB_H
|
|
|
|
#include "leveldbwrapper.h"
|
|
#include "main.h"
|
|
|
|
#include <map>
|
|
#include <string>
|
|
#include <utility>
|
|
#include <vector>
|
|
|
|
class CCoins;
|
|
class uint256;
|
|
|
|
// -dbcache default (MiB)
|
|
static const int64_t nDefaultDbCache = 100;
|
|
// max. -dbcache in (MiB)
|
|
static const int64_t nMaxDbCache = sizeof(void*) > 4 ? 4096 : 1024;
|
|
// min. -dbcache in (MiB)
|
|
static const int64_t nMinDbCache = 4;
|
|
|
|
/** CCoinsView backed by the LevelDB coin database (chainstate/) */
|
|
class CCoinsViewDB : public CCoinsView
|
|
{
|
|
protected:
|
|
CLevelDBWrapper db;
|
|
public:
|
|
CCoinsViewDB(size_t nCacheSize, bool fMemory = false, bool fWipe = false);
|
|
|
|
bool GetCoins(const uint256 &txid, CCoins &coins) const;
|
|
bool HaveCoins(const uint256 &txid) const;
|
|
uint256 GetBestBlock() const;
|
|
bool BatchWrite(CCoinsMap &mapCoins, const uint256 &hashBlock);
|
|
bool GetStats(CCoinsStats &stats) const;
|
|
};
|
|
|
|
/** Access to the block database (blocks/index/) */
|
|
class CBlockTreeDB : public CLevelDBWrapper
|
|
{
|
|
public:
|
|
CBlockTreeDB(size_t nCacheSize, bool fMemory = false, bool fWipe = false);
|
|
private:
|
|
CBlockTreeDB(const CBlockTreeDB&);
|
|
void operator=(const CBlockTreeDB&);
|
|
public:
|
|
bool WriteBlockIndex(const CDiskBlockIndex& blockindex);
|
|
bool ReadBlockFileInfo(int nFile, CBlockFileInfo &fileinfo);
|
|
bool WriteBlockFileInfo(int nFile, const CBlockFileInfo &fileinfo);
|
|
bool ReadLastBlockFile(int &nFile);
|
|
bool WriteLastBlockFile(int nFile);
|
|
bool WriteReindexing(bool fReindex);
|
|
bool ReadReindexing(bool &fReindex);
|
|
bool ReadTxIndex(const uint256 &txid, CDiskTxPos &pos);
|
|
bool WriteTxIndex(const std::vector<std::pair<uint256, CDiskTxPos> > &list);
|
|
bool WriteFlag(const std::string &name, bool fValue);
|
|
bool ReadFlag(const std::string &name, bool &fValue);
|
|
bool LoadBlockIndexGuts();
|
|
};
|
|
|
|
#endif // BITCOIN_TXDB_LEVELDB_H
|