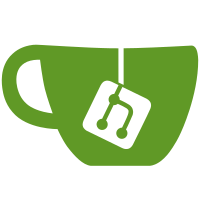
44b64b9 Fix edge case with stale fee estimates (Alex Morcos) 78ae62d Add clarifying comments to fee estimation (Alex Morcos) 5fe0f47 Add extra logging to processBlock in fee estimation. (Alex Morcos) dc008c4 Add IsCurrentForFeeEstimatation (Alex Morcos) ebafdca Pass pointers to existing CTxMemPoolEntries to fee estimation (Alex Morcos) d825838 Always update fee estimates on new blocks. (Alex Morcos) 6f06b26 rename bool to validFeeEstimate (Alex Morcos) 84f7ab0 Remove member variable hadNoDependencies from CTxMemPoolEntry (Alex Morcos) 60ac00d Don't track transactions at all during IBD. (Alex Morcos) 4df4479 Remove extraneous LogPrint from fee estimation (Alex Morcos)
93 lines
2.9 KiB
C++
93 lines
2.9 KiB
C++
// Copyright (c) 2015 The Bitcoin Core developers
|
|
// Copyright (c) 2014-2017 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_TEST_TEST_DASH_H
|
|
#define BITCOIN_TEST_TEST_DASH_H
|
|
|
|
#include "chainparamsbase.h"
|
|
#include "key.h"
|
|
#include "pubkey.h"
|
|
#include "txdb.h"
|
|
#include "txmempool.h"
|
|
|
|
#include <boost/filesystem.hpp>
|
|
#include <boost/thread.hpp>
|
|
|
|
/** Basic testing setup.
|
|
* This just configures logging and chain parameters.
|
|
*/
|
|
struct BasicTestingSetup {
|
|
ECCVerifyHandle globalVerifyHandle;
|
|
|
|
BasicTestingSetup(const std::string& chainName = CBaseChainParams::MAIN);
|
|
~BasicTestingSetup();
|
|
};
|
|
|
|
/** Testing setup that configures a complete environment.
|
|
* Included are data directory, coins database, script check threads setup.
|
|
*/
|
|
class CConnman;
|
|
struct TestingSetup: public BasicTestingSetup {
|
|
CCoinsViewDB *pcoinsdbview;
|
|
boost::filesystem::path pathTemp;
|
|
boost::thread_group threadGroup;
|
|
CConnman* connman;
|
|
|
|
TestingSetup(const std::string& chainName = CBaseChainParams::MAIN);
|
|
~TestingSetup();
|
|
};
|
|
|
|
class CBlock;
|
|
struct CMutableTransaction;
|
|
class CScript;
|
|
|
|
//
|
|
// Testing fixture that pre-creates a
|
|
// 100-block REGTEST-mode block chain
|
|
//
|
|
struct TestChain100Setup : public TestingSetup {
|
|
TestChain100Setup();
|
|
|
|
// Create a new block with just given transactions, coinbase paying to
|
|
// scriptPubKey, and try to add it to the current chain.
|
|
CBlock CreateAndProcessBlock(const std::vector<CMutableTransaction>& txns,
|
|
const CScript& scriptPubKey);
|
|
|
|
~TestChain100Setup();
|
|
|
|
std::vector<CTransaction> coinbaseTxns; // For convenience, coinbase transactions
|
|
CKey coinbaseKey; // private/public key needed to spend coinbase transactions
|
|
};
|
|
|
|
class CTxMemPoolEntry;
|
|
class CTxMemPool;
|
|
|
|
struct TestMemPoolEntryHelper
|
|
{
|
|
// Default values
|
|
CAmount nFee;
|
|
int64_t nTime;
|
|
double dPriority;
|
|
unsigned int nHeight;
|
|
bool spendsCoinbase;
|
|
unsigned int sigOpCount;
|
|
LockPoints lp;
|
|
|
|
TestMemPoolEntryHelper() :
|
|
nFee(0), nTime(0), dPriority(0.0), nHeight(1),
|
|
spendsCoinbase(false), sigOpCount(4) { }
|
|
|
|
CTxMemPoolEntry FromTx(const CMutableTransaction &tx, CTxMemPool *pool = NULL);
|
|
|
|
// Change the default value
|
|
TestMemPoolEntryHelper &Fee(CAmount _fee) { nFee = _fee; return *this; }
|
|
TestMemPoolEntryHelper &Time(int64_t _time) { nTime = _time; return *this; }
|
|
TestMemPoolEntryHelper &Priority(double _priority) { dPriority = _priority; return *this; }
|
|
TestMemPoolEntryHelper &Height(unsigned int _height) { nHeight = _height; return *this; }
|
|
TestMemPoolEntryHelper &SpendsCoinbase(bool _flag) { spendsCoinbase = _flag; return *this; }
|
|
TestMemPoolEntryHelper &SigOps(unsigned int _sigops) { sigOpCount = _sigops; return *this; }
|
|
};
|
|
#endif
|