mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 05:23:01 +01:00
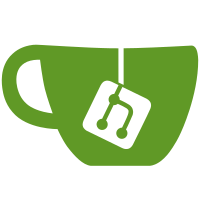
92f1f8b31
Split off key_io_tests from base58_tests (Pieter Wuille)119b0f85e
Split key_io (address/key encodings) off from base58 (Pieter Wuille)ebfe217b1
Stop using CBase58Data for ext keys (Pieter Wuille)32e69fa0d
Replace CBitcoinSecret with {Encode,Decode}Secret (Pieter Wuille) Pull request description: This PR contains some of the changes left as TODO in #11167 (and built on top of that PR). They are not intended for backporting. This removes the `CBase58`, `CBitcoinSecret`, `CBitcoinExtKey`, and `CBitcoinExtPubKey` classes, in favor of simple `Encode`/`Decode` functions. Furthermore, all Bitcoin-specific logic (addresses, WIF, BIP32) is moved to `key_io.{h,cpp}`, leaving `base58.{h,cpp}` as a pure utility that implements the base58 encoding/decoding logic. Tree-SHA512: a5962c0ed27ad53cbe00f22af432cf11aa530e3efc9798e25c004bc9ed1b5673db5df3956e398ee2c085e3a136ac8da69fe7a7d97a05fb2eb3be0b60d0479655 Make linter happy Dashify
67 lines
2.1 KiB
C++
67 lines
2.1 KiB
C++
// Copyright (c) 2014-2020 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <test/data/bip39_vectors.json.h>
|
|
|
|
#include <key.h>
|
|
#include <key_io.h>
|
|
#include <util.h>
|
|
#include <utilstrencodings.h>
|
|
#include <test/test_dash.h>
|
|
#include <bip39.h>
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
#include <univalue.h>
|
|
|
|
// In script_tests.cpp
|
|
extern UniValue read_json(const std::string& jsondata);
|
|
|
|
BOOST_FIXTURE_TEST_SUITE(bip39_tests, BasicTestingSetup)
|
|
|
|
// https://github.com/trezor/python-mnemonic/blob/b502451a33a440783926e04428115e0bed87d01f/vectors.json
|
|
BOOST_AUTO_TEST_CASE(bip39_vectors)
|
|
{
|
|
UniValue tests = read_json(std::string(json_tests::bip39_vectors, json_tests::bip39_vectors + sizeof(json_tests::bip39_vectors)));
|
|
|
|
for (unsigned int i = 0; i < tests.size(); i++) {
|
|
// printf("%d\n", i);
|
|
UniValue test = tests[i];
|
|
std::string strTest = test.write();
|
|
if (test.size() < 4) // Allow for extra stuff (useful for comments)
|
|
{
|
|
BOOST_ERROR("Bad test: " << strTest);
|
|
continue;
|
|
}
|
|
|
|
std::vector<uint8_t> vData = ParseHex(test[0].get_str());
|
|
SecureVector data(vData.begin(), vData.end());
|
|
|
|
SecureString m = CMnemonic::FromData(data, data.size());
|
|
std::string strMnemonic = test[1].get_str();
|
|
SecureString mnemonic(strMnemonic.begin(), strMnemonic.end());
|
|
|
|
// printf("%s\n%s\n", m.c_str(), mnemonic.c_str());
|
|
BOOST_CHECK(m == mnemonic);
|
|
BOOST_CHECK(CMnemonic::Check(mnemonic));
|
|
|
|
SecureVector seed;
|
|
SecureString passphrase("TREZOR");
|
|
CMnemonic::ToSeed(mnemonic, passphrase, seed);
|
|
// printf("seed: %s\n", HexStr(seed).c_str());
|
|
BOOST_CHECK(HexStr(seed) == test[2].get_str());
|
|
|
|
CExtKey key;
|
|
CExtPubKey pubkey;
|
|
|
|
key.SetMaster(seed.data(), 64);
|
|
pubkey = key.Neuter();
|
|
|
|
// printf("CBitcoinExtKey: %s\n", EncodeExtKey(key).c_str());
|
|
BOOST_CHECK(EncodeExtKey(key) == test[3].get_str());
|
|
}
|
|
}
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|