mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 13:32:47 +01:00
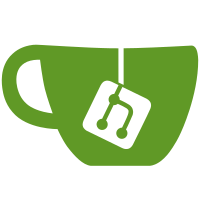
05e023f
Move CloseSocket out of SetSocketNonBlocking and pass SOCKET by const reference in SetSocket* functions (Dag Robole)
Pull request description:
Rationale:
Readability, SetSocketNonBlocking does what it says on the tin.
Consistency, More consistent with the rest of the API in this unit.
Reusability, SetSocketNonBlocking can also be used by clients that may not want to close the socket on failure.
This also moves the responsibility of closing the socket back to the caller that opened it, which in general should know better how and when to close it.
Tree-SHA512: 85027137f1b626e2b636549ee38cc757a587adcf464c84be6e65ca16e3b75d7ed1a1b21dd70dbe34c7c5d599af39e53b89932dfe3c74f91a22341ff3af5ea80a
71 lines
2.6 KiB
C++
71 lines
2.6 KiB
C++
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_NETBASE_H
|
|
#define BITCOIN_NETBASE_H
|
|
|
|
#if defined(HAVE_CONFIG_H)
|
|
#include "config/dash-config.h"
|
|
#endif
|
|
|
|
#include "compat.h"
|
|
#include "netaddress.h"
|
|
#include "serialize.h"
|
|
|
|
#include <stdint.h>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
extern int nConnectTimeout;
|
|
extern bool fNameLookup;
|
|
|
|
//! -timeout default
|
|
static const int DEFAULT_CONNECT_TIMEOUT = 5000;
|
|
//! -dns default
|
|
static const int DEFAULT_NAME_LOOKUP = true;
|
|
static const bool DEFAULT_ALLOWPRIVATENET = false;
|
|
|
|
class proxyType
|
|
{
|
|
public:
|
|
proxyType(): randomize_credentials(false) {}
|
|
proxyType(const CService &_proxy, bool _randomize_credentials=false): proxy(_proxy), randomize_credentials(_randomize_credentials) {}
|
|
|
|
bool IsValid() const { return proxy.IsValid(); }
|
|
|
|
CService proxy;
|
|
bool randomize_credentials;
|
|
};
|
|
|
|
enum Network ParseNetwork(std::string net);
|
|
std::string GetNetworkName(enum Network net);
|
|
bool SetProxy(enum Network net, const proxyType &addrProxy);
|
|
bool GetProxy(enum Network net, proxyType &proxyInfoOut);
|
|
bool IsProxy(const CNetAddr &addr);
|
|
bool SetNameProxy(const proxyType &addrProxy);
|
|
bool HaveNameProxy();
|
|
bool LookupHost(const char *pszName, std::vector<CNetAddr>& vIP, unsigned int nMaxSolutions, bool fAllowLookup);
|
|
bool LookupHost(const char *pszName, CNetAddr& addr, bool fAllowLookup);
|
|
bool Lookup(const char *pszName, CService& addr, int portDefault, bool fAllowLookup);
|
|
bool Lookup(const char *pszName, std::vector<CService>& vAddr, int portDefault, bool fAllowLookup, unsigned int nMaxSolutions);
|
|
CService LookupNumeric(const char *pszName, int portDefault = 0);
|
|
bool LookupSubNet(const char *pszName, CSubNet& subnet);
|
|
bool ConnectSocket(const CService &addr, SOCKET& hSocketRet, int nTimeout, bool *outProxyConnectionFailed = 0);
|
|
bool ConnectSocketByName(CService &addr, SOCKET& hSocketRet, const char *pszDest, int portDefault, int nTimeout, bool *outProxyConnectionFailed = 0);
|
|
/** Return readable error string for a network error code */
|
|
std::string NetworkErrorString(int err);
|
|
/** Close socket and set hSocket to INVALID_SOCKET */
|
|
bool CloseSocket(SOCKET& hSocket);
|
|
/** Disable or enable blocking-mode for a socket */
|
|
bool SetSocketNonBlocking(const SOCKET& hSocket, bool fNonBlocking);
|
|
/** Set the TCP_NODELAY flag on a socket */
|
|
bool SetSocketNoDelay(const SOCKET& hSocket);
|
|
/**
|
|
* Convert milliseconds to a struct timeval for e.g. select.
|
|
*/
|
|
struct timeval MillisToTimeval(int64_t nTimeout);
|
|
void InterruptSocks5(bool interrupt);
|
|
|
|
#endif // BITCOIN_NETBASE_H
|