mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
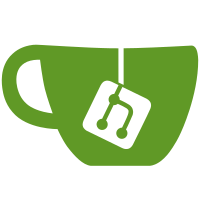
fab0c820fa4c0c3227eec85c64310a3bf938a149 rpc: Clarify that block count means height excl genesis (MarcoFalke)
Pull request description:
There is a common misconception that the block count returned by the blockchain rpcs includes the genesis block. See for example the discussion in https://github.com/bitcoin/bitcoin/pull/16292#issuecomment-506303256.
However, it really returns the height, which is `0` for the genesis block.
So clarify that and also remove the misleading "longest blockchain" comment.
Finally, fix the wallet test that incorrectly used this rpc.
ACKs for top commit:
instagibbs:
utACK fab0c820fa
promag:
ACK fab0c82, sorry for the misconception.
Tree-SHA512: 0d087cbb628d3866352bca6420402f392e6a997e579941701a408a7fca355d84645045661f39b022e4479cc07f85a6cddaa9095b6fd9911b245692482420a5e4
89 lines
3.3 KiB
Python
Executable File
89 lines
3.3 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2017 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test that the wallet resends transactions periodically."""
|
|
from collections import defaultdict
|
|
import time
|
|
|
|
from test_framework.blocktools import create_block, create_coinbase
|
|
from test_framework.messages import ToHex
|
|
from test_framework.mininode import P2PInterface, mininode_lock
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal, wait_until
|
|
|
|
|
|
class P2PStoreTxInvs(P2PInterface):
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.tx_invs_received = defaultdict(int)
|
|
|
|
def on_inv(self, message):
|
|
# Store how many times invs have been received for each tx.
|
|
for i in message.inv:
|
|
if i.type == 1:
|
|
# save txid
|
|
self.tx_invs_received[i.hash] += 1
|
|
|
|
|
|
class ResendWalletTransactionsTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 1
|
|
|
|
def skip_test_if_missing_module(self):
|
|
self.skip_if_no_wallet()
|
|
|
|
def run_test(self):
|
|
node = self.nodes[0] # alias
|
|
|
|
node.add_p2p_connection(P2PStoreTxInvs())
|
|
|
|
self.log.info("Create a new transaction and wait until it's broadcast")
|
|
txid = int(node.sendtoaddress(node.getnewaddress(), 1), 16)
|
|
|
|
# Wallet rebroadcast is first scheduled 1 sec after startup (see
|
|
# nNextResend in ResendWalletTransactions()). Sleep for just over a
|
|
# second to be certain that it has been called before the first
|
|
# setmocktime call below.
|
|
time.sleep(1.1)
|
|
|
|
# Can take a few seconds due to transaction trickling
|
|
def wait_p2p():
|
|
self.bump_mocktime(1)
|
|
return node.p2p.tx_invs_received[txid] >= 1
|
|
wait_until(wait_p2p, lock=mininode_lock)
|
|
|
|
# Add a second peer since txs aren't rebroadcast to the same peer (see filterInventoryKnown)
|
|
node.add_p2p_connection(P2PStoreTxInvs())
|
|
|
|
self.log.info("Create a block")
|
|
# Create and submit a block without the transaction.
|
|
# Transactions are only rebroadcast if there has been a block at least five minutes
|
|
# after the last time we tried to broadcast. Use mocktime and give an extra minute to be sure.
|
|
block_time = self.mocktime + 6 * 60
|
|
node.setmocktime(block_time)
|
|
block = create_block(int(node.getbestblockhash(), 16), create_coinbase(node.getblockcount() + 1), block_time)
|
|
block.rehash()
|
|
block.solve()
|
|
node.submitblock(ToHex(block))
|
|
|
|
# Transaction should not be rebroadcast
|
|
node.syncwithvalidationinterfacequeue()
|
|
node.p2ps[1].sync_with_ping()
|
|
assert_equal(node.p2ps[1].tx_invs_received[txid], 0)
|
|
|
|
self.log.info("Transaction should be rebroadcast after 30 minutes")
|
|
# Use mocktime and give an extra 5 minutes to be sure.
|
|
rebroadcast_time = self.mocktime + 41 * 60
|
|
node.setmocktime(rebroadcast_time)
|
|
self.mocktime = rebroadcast_time
|
|
|
|
def wait_p2p_1():
|
|
self.bump_mocktime(1)
|
|
return node.p2ps[1].tx_invs_received[txid] >= 1
|
|
wait_until(wait_p2p_1, lock=mininode_lock)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
ResendWalletTransactionsTest().main()
|