mirror of
https://github.com/dashpay/dash.git
synced 2024-12-25 20:12:57 +01:00
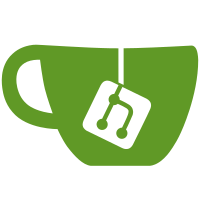
* scripted-diff: Replace #include "" with #include <> (ryanofsky) -BEGIN VERIFY SCRIPT- for f in \ src/*.cpp \ src/*.h \ src/bench/*.cpp \ src/bench/*.h \ src/compat/*.cpp \ src/compat/*.h \ src/consensus/*.cpp \ src/consensus/*.h \ src/crypto/*.cpp \ src/crypto/*.h \ src/crypto/ctaes/*.h \ src/policy/*.cpp \ src/policy/*.h \ src/primitives/*.cpp \ src/primitives/*.h \ src/qt/*.cpp \ src/qt/*.h \ src/qt/test/*.cpp \ src/qt/test/*.h \ src/rpc/*.cpp \ src/rpc/*.h \ src/script/*.cpp \ src/script/*.h \ src/support/*.cpp \ src/support/*.h \ src/support/allocators/*.h \ src/test/*.cpp \ src/test/*.h \ src/wallet/*.cpp \ src/wallet/*.h \ src/wallet/test/*.cpp \ src/wallet/test/*.h \ src/zmq/*.cpp \ src/zmq/*.h do base=${f%/*}/ relbase=${base#src/} sed -i "s:#include \"\(.*\)\"\(.*\):if test -e \$base'\\1'; then echo \"#include <\"\$relbase\"\\1>\\2\"; else echo \"#include <\\1>\\2\"; fi:e" $f done -END VERIFY SCRIPT- Signed-off-by: Pasta <pasta@dashboost.org> * scripted-diff: Replace #include "" with #include <> (Dash Specific) -BEGIN VERIFY SCRIPT- for f in \ src/bls/*.cpp \ src/bls/*.h \ src/evo/*.cpp \ src/evo/*.h \ src/governance/*.cpp \ src/governance/*.h \ src/llmq/*.cpp \ src/llmq/*.h \ src/masternode/*.cpp \ src/masternode/*.h \ src/privatesend/*.cpp \ src/privatesend/*.h do base=${f%/*}/ relbase=${base#src/} sed -i "s:#include \"\(.*\)\"\(.*\):if test -e \$base'\\1'; then echo \"#include <\"\$relbase\"\\1>\\2\"; else echo \"#include <\\1>\\2\"; fi:e" $f done -END VERIFY SCRIPT- Signed-off-by: Pasta <pasta@dashboost.org> * build: Remove -I for everything but project root Remove -I from build system for everything but the project root, and built-in dependencies. Signed-off-by: Pasta <pasta@dashboost.org> # Conflicts: # src/Makefile.test.include * qt: refactor: Use absolute include paths in .ui files * qt: refactor: Changes to make include paths absolute This makes all include paths in the GUI absolute. Many changes are involved as every single source file in src/qt/ assumes to be able to use relative includes. Signed-off-by: Pasta <pasta@dashboost.org> # Conflicts: # src/qt/dash.cpp # src/qt/optionsmodel.cpp # src/qt/test/rpcnestedtests.cpp * test: refactor: Use absolute include paths for test data files * Recommend #include<> syntax in developer notes * refactor: Include obj/build.h instead of build.h * END BACKPORT #11651 Remove trailing whitespace causing travis failure * fix backport 11651 Signed-off-by: Pasta <pasta@dashboost.org> * More of 11651 * fix blockchain.cpp Signed-off-by: pasta <pasta@dashboost.org> * Add missing "qt/" in includes * Add missing "test/" in includes * Fix trailing whitespaces Co-authored-by: Wladimir J. van der Laan <laanwj@gmail.com> Co-authored-by: Russell Yanofsky <russ@yanofsky.org> Co-authored-by: MeshCollider <dobsonsa68@gmail.com> Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
251 lines
9.5 KiB
C++
251 lines
9.5 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <pow.h>
|
|
|
|
#include <arith_uint256.h>
|
|
#include <chain.h>
|
|
#include <chainparams.h>
|
|
#include <primitives/block.h>
|
|
#include <uint256.h>
|
|
|
|
#include <math.h>
|
|
|
|
unsigned int static KimotoGravityWell(const CBlockIndex* pindexLast, const Consensus::Params& params) {
|
|
const CBlockIndex *BlockLastSolved = pindexLast;
|
|
const CBlockIndex *BlockReading = pindexLast;
|
|
uint64_t PastBlocksMass = 0;
|
|
int64_t PastRateActualSeconds = 0;
|
|
int64_t PastRateTargetSeconds = 0;
|
|
double PastRateAdjustmentRatio = double(1);
|
|
arith_uint256 PastDifficultyAverage;
|
|
arith_uint256 PastDifficultyAveragePrev;
|
|
double EventHorizonDeviation;
|
|
double EventHorizonDeviationFast;
|
|
double EventHorizonDeviationSlow;
|
|
|
|
uint64_t pastSecondsMin = params.nPowTargetTimespan * 0.025;
|
|
uint64_t pastSecondsMax = params.nPowTargetTimespan * 7;
|
|
uint64_t PastBlocksMin = pastSecondsMin / params.nPowTargetSpacing;
|
|
uint64_t PastBlocksMax = pastSecondsMax / params.nPowTargetSpacing;
|
|
|
|
if (BlockLastSolved == nullptr || BlockLastSolved->nHeight == 0 || (uint64_t)BlockLastSolved->nHeight < PastBlocksMin) { return UintToArith256(params.powLimit).GetCompact(); }
|
|
|
|
for (unsigned int i = 1; BlockReading && BlockReading->nHeight > 0; i++) {
|
|
if (PastBlocksMax > 0 && i > PastBlocksMax) { break; }
|
|
PastBlocksMass++;
|
|
|
|
PastDifficultyAverage.SetCompact(BlockReading->nBits);
|
|
if (i > 1) {
|
|
// handle negative arith_uint256
|
|
if(PastDifficultyAverage >= PastDifficultyAveragePrev)
|
|
PastDifficultyAverage = ((PastDifficultyAverage - PastDifficultyAveragePrev) / i) + PastDifficultyAveragePrev;
|
|
else
|
|
PastDifficultyAverage = PastDifficultyAveragePrev - ((PastDifficultyAveragePrev - PastDifficultyAverage) / i);
|
|
}
|
|
PastDifficultyAveragePrev = PastDifficultyAverage;
|
|
|
|
PastRateActualSeconds = BlockLastSolved->GetBlockTime() - BlockReading->GetBlockTime();
|
|
PastRateTargetSeconds = params.nPowTargetSpacing * PastBlocksMass;
|
|
PastRateAdjustmentRatio = double(1);
|
|
if (PastRateActualSeconds < 0) { PastRateActualSeconds = 0; }
|
|
if (PastRateActualSeconds != 0 && PastRateTargetSeconds != 0) {
|
|
PastRateAdjustmentRatio = double(PastRateTargetSeconds) / double(PastRateActualSeconds);
|
|
}
|
|
EventHorizonDeviation = 1 + (0.7084 * pow((double(PastBlocksMass)/double(28.2)), -1.228));
|
|
EventHorizonDeviationFast = EventHorizonDeviation;
|
|
EventHorizonDeviationSlow = 1 / EventHorizonDeviation;
|
|
|
|
if (PastBlocksMass >= PastBlocksMin) {
|
|
if ((PastRateAdjustmentRatio <= EventHorizonDeviationSlow) || (PastRateAdjustmentRatio >= EventHorizonDeviationFast))
|
|
{ assert(BlockReading); break; }
|
|
}
|
|
if (BlockReading->pprev == nullptr) { assert(BlockReading); break; }
|
|
BlockReading = BlockReading->pprev;
|
|
}
|
|
|
|
arith_uint256 bnNew(PastDifficultyAverage);
|
|
if (PastRateActualSeconds != 0 && PastRateTargetSeconds != 0) {
|
|
bnNew *= PastRateActualSeconds;
|
|
bnNew /= PastRateTargetSeconds;
|
|
}
|
|
|
|
if (bnNew > UintToArith256(params.powLimit)) {
|
|
bnNew = UintToArith256(params.powLimit);
|
|
}
|
|
|
|
return bnNew.GetCompact();
|
|
}
|
|
|
|
unsigned int static DarkGravityWave(const CBlockIndex* pindexLast, const Consensus::Params& params) {
|
|
/* current difficulty formula, dash - DarkGravity v3, written by Evan Duffield - evan@dash.org */
|
|
const arith_uint256 bnPowLimit = UintToArith256(params.powLimit);
|
|
int64_t nPastBlocks = 24;
|
|
|
|
// make sure we have at least (nPastBlocks + 1) blocks, otherwise just return powLimit
|
|
if (!pindexLast || pindexLast->nHeight < nPastBlocks) {
|
|
return bnPowLimit.GetCompact();
|
|
}
|
|
|
|
const CBlockIndex *pindex = pindexLast;
|
|
arith_uint256 bnPastTargetAvg;
|
|
|
|
for (unsigned int nCountBlocks = 1; nCountBlocks <= nPastBlocks; nCountBlocks++) {
|
|
arith_uint256 bnTarget = arith_uint256().SetCompact(pindex->nBits);
|
|
if (nCountBlocks == 1) {
|
|
bnPastTargetAvg = bnTarget;
|
|
} else {
|
|
// NOTE: that's not an average really...
|
|
bnPastTargetAvg = (bnPastTargetAvg * nCountBlocks + bnTarget) / (nCountBlocks + 1);
|
|
}
|
|
|
|
if(nCountBlocks != nPastBlocks) {
|
|
assert(pindex->pprev); // should never fail
|
|
pindex = pindex->pprev;
|
|
}
|
|
}
|
|
|
|
arith_uint256 bnNew(bnPastTargetAvg);
|
|
|
|
int64_t nActualTimespan = pindexLast->GetBlockTime() - pindex->GetBlockTime();
|
|
// NOTE: is this accurate? nActualTimespan counts it for (nPastBlocks - 1) blocks only...
|
|
int64_t nTargetTimespan = nPastBlocks * params.nPowTargetSpacing;
|
|
|
|
if (nActualTimespan < nTargetTimespan/3)
|
|
nActualTimespan = nTargetTimespan/3;
|
|
if (nActualTimespan > nTargetTimespan*3)
|
|
nActualTimespan = nTargetTimespan*3;
|
|
|
|
// Retarget
|
|
bnNew *= nActualTimespan;
|
|
bnNew /= nTargetTimespan;
|
|
|
|
if (bnNew > bnPowLimit) {
|
|
bnNew = bnPowLimit;
|
|
}
|
|
|
|
return bnNew.GetCompact();
|
|
}
|
|
|
|
unsigned int GetNextWorkRequiredBTC(const CBlockIndex* pindexLast, const CBlockHeader *pblock, const Consensus::Params& params)
|
|
{
|
|
assert(pindexLast != nullptr);
|
|
unsigned int nProofOfWorkLimit = UintToArith256(params.powLimit).GetCompact();
|
|
|
|
// Only change once per interval
|
|
if ((pindexLast->nHeight+1) % params.DifficultyAdjustmentInterval() != 0)
|
|
{
|
|
if (params.fPowAllowMinDifficultyBlocks)
|
|
{
|
|
// Special difficulty rule for testnet:
|
|
// If the new block's timestamp is more than 2* 2.5 minutes
|
|
// then allow mining of a min-difficulty block.
|
|
if (pblock->GetBlockTime() > pindexLast->GetBlockTime() + params.nPowTargetSpacing*2)
|
|
return nProofOfWorkLimit;
|
|
else
|
|
{
|
|
// Return the last non-special-min-difficulty-rules-block
|
|
const CBlockIndex* pindex = pindexLast;
|
|
while (pindex->pprev && pindex->nHeight % params.DifficultyAdjustmentInterval() != 0 && pindex->nBits == nProofOfWorkLimit)
|
|
pindex = pindex->pprev;
|
|
return pindex->nBits;
|
|
}
|
|
}
|
|
return pindexLast->nBits;
|
|
}
|
|
|
|
// Go back by what we want to be 1 day worth of blocks
|
|
int nHeightFirst = pindexLast->nHeight - (params.DifficultyAdjustmentInterval()-1);
|
|
assert(nHeightFirst >= 0);
|
|
const CBlockIndex* pindexFirst = pindexLast->GetAncestor(nHeightFirst);
|
|
assert(pindexFirst);
|
|
|
|
return CalculateNextWorkRequired(pindexLast, pindexFirst->GetBlockTime(), params);
|
|
}
|
|
|
|
unsigned int GetNextWorkRequired(const CBlockIndex* pindexLast, const CBlockHeader *pblock, const Consensus::Params& params)
|
|
{
|
|
assert(pindexLast != nullptr);
|
|
assert(pblock != nullptr);
|
|
const arith_uint256 bnPowLimit = UintToArith256(params.powLimit);
|
|
|
|
// this is only active on devnets
|
|
if (pindexLast->nHeight < params.nMinimumDifficultyBlocks) {
|
|
return bnPowLimit.GetCompact();
|
|
}
|
|
|
|
if (pindexLast->nHeight + 1 < params.nPowKGWHeight) {
|
|
return GetNextWorkRequiredBTC(pindexLast, pblock, params);
|
|
}
|
|
|
|
// Note: GetNextWorkRequiredBTC has it's own special difficulty rule,
|
|
// so we only apply this to post-BTC algos.
|
|
if (params.fPowAllowMinDifficultyBlocks) {
|
|
// recent block is more than 2 hours old
|
|
if (pblock->GetBlockTime() > pindexLast->GetBlockTime() + 2 * 60 * 60) {
|
|
return bnPowLimit.GetCompact();
|
|
}
|
|
// recent block is more than 10 minutes old
|
|
if (pblock->GetBlockTime() > pindexLast->GetBlockTime() + params.nPowTargetSpacing * 4) {
|
|
arith_uint256 bnNew = arith_uint256().SetCompact(pindexLast->nBits) * 10;
|
|
if (bnNew > bnPowLimit) {
|
|
return bnPowLimit.GetCompact();
|
|
}
|
|
return bnNew.GetCompact();
|
|
}
|
|
}
|
|
|
|
if (pindexLast->nHeight + 1 < params.nPowDGWHeight) {
|
|
return KimotoGravityWell(pindexLast, params);
|
|
}
|
|
|
|
return DarkGravityWave(pindexLast, params);
|
|
}
|
|
|
|
// for DIFF_BTC only!
|
|
unsigned int CalculateNextWorkRequired(const CBlockIndex* pindexLast, int64_t nFirstBlockTime, const Consensus::Params& params)
|
|
{
|
|
if (params.fPowNoRetargeting)
|
|
return pindexLast->nBits;
|
|
|
|
// Limit adjustment step
|
|
int64_t nActualTimespan = pindexLast->GetBlockTime() - nFirstBlockTime;
|
|
if (nActualTimespan < params.nPowTargetTimespan/4)
|
|
nActualTimespan = params.nPowTargetTimespan/4;
|
|
if (nActualTimespan > params.nPowTargetTimespan*4)
|
|
nActualTimespan = params.nPowTargetTimespan*4;
|
|
|
|
// Retarget
|
|
const arith_uint256 bnPowLimit = UintToArith256(params.powLimit);
|
|
arith_uint256 bnNew;
|
|
bnNew.SetCompact(pindexLast->nBits);
|
|
bnNew *= nActualTimespan;
|
|
bnNew /= params.nPowTargetTimespan;
|
|
|
|
if (bnNew > bnPowLimit)
|
|
bnNew = bnPowLimit;
|
|
|
|
return bnNew.GetCompact();
|
|
}
|
|
|
|
bool CheckProofOfWork(uint256 hash, unsigned int nBits, const Consensus::Params& params)
|
|
{
|
|
bool fNegative;
|
|
bool fOverflow;
|
|
arith_uint256 bnTarget;
|
|
|
|
bnTarget.SetCompact(nBits, &fNegative, &fOverflow);
|
|
|
|
// Check range
|
|
if (fNegative || bnTarget == 0 || fOverflow || bnTarget > UintToArith256(params.powLimit))
|
|
return false;
|
|
|
|
// Check proof of work matches claimed amount
|
|
if (UintToArith256(hash) > bnTarget)
|
|
return false;
|
|
|
|
return true;
|
|
}
|