mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
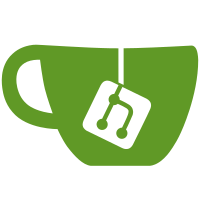
bdb8b9a347e68f80a2e8d44ce5590a2e8214b6bb test: doc: improve doc for `from_hex` helper (mention `to_hex` alternative) (Sebastian Falbesoner) 191405420815d49ab50184513717a303fc2744d6 scripted-diff: test: rename `FromHex` to `from_hex` (Sebastian Falbesoner) a79396fe5f8f81c78cf84117a87074c6ff6c9d95 test: remove `ToHex` helper, use .serialize().hex() instead (Sebastian Falbesoner) 2ce7b47958c4a10ba20dc86c011d71cda4b070a5 test: introduce `tx_from_hex` helper for tx deserialization (Sebastian Falbesoner) Pull request description: There are still many functional tests that perform conversions from a hex-string to a message object (deserialization) manually. This PR identifies all those instances and replaces them with a newly introduced helper `tx_from_hex`. Instances were found via * `git grep "deserialize.*BytesIO"` and some of them manually, when it were not one-liners. Further, the helper `ToHex` was removed and simply replaced by `.serialize().hex()`, since now both variants are in use (sometimes even within the same test) and using the helper doesn't really have an advantage in readability. (see discussion https://github.com/bitcoin/bitcoin/pull/22257#discussion_r652404782) ACKs for top commit: MarcoFalke: review re-ACK bdb8b9a347e68f80a2e8d44ce5590a2e8214b6bb 😁 Tree-SHA512: e25d7dc85918de1d6755a5cea65471b07a743204c20ad1c2f71ff07ef48cc1b9ad3fe5f515c1efaba2b2e3d89384e7980380c5d81895f9826e2046808cd3266e
76 lines
2.9 KiB
Python
Executable File
76 lines
2.9 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2015-2023 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
from test_framework.messages import COIN, COutPoint, CTransaction, CTxIn, CTxOut
|
|
from test_framework.script import CScript, OP_CAT, OP_DROP, OP_TRUE
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_raises_rpc_error, softfork_active, satoshi_round
|
|
|
|
'''
|
|
feature_dip0020_activation.py
|
|
|
|
This test checks activation of DIP0020 opcodes
|
|
'''
|
|
|
|
DISABLED_OPCODE_ERROR = "non-mandatory-script-verify-flag (Attempted to use a disabled opcode)"
|
|
|
|
|
|
class DIP0020ActivationTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 1
|
|
self.extra_args = [["-acceptnonstdtxn=1"]]
|
|
|
|
def skip_test_if_missing_module(self):
|
|
self.skip_if_no_wallet()
|
|
|
|
def run_test(self):
|
|
self.node = self.nodes[0]
|
|
self.relayfee = satoshi_round(self.nodes[0].getnetworkinfo()["relayfee"])
|
|
|
|
# We should have some coins already
|
|
utxos = self.node.listunspent()
|
|
assert len(utxos) > 0
|
|
|
|
# Lock some coins using disabled opcodes
|
|
utxo = utxos[len(utxos) - 1]
|
|
value = int(satoshi_round(utxo["amount"] - self.relayfee) * COIN)
|
|
tx = CTransaction()
|
|
tx.vin.append(CTxIn(COutPoint(int(utxo["txid"], 16), utxo["vout"])))
|
|
tx.vout.append(CTxOut(value, CScript([b'1', b'2', OP_CAT])))
|
|
tx_signed_hex = self.node.signrawtransactionwithwallet(tx.serialize().hex())["hex"]
|
|
txid = self.node.sendrawtransaction(tx_signed_hex)
|
|
|
|
# This tx should be completely valid, should be included in mempool and mined in the next block
|
|
assert txid in set(self.node.getrawmempool())
|
|
self.node.generate(1)
|
|
assert txid not in set(self.node.getrawmempool())
|
|
|
|
# Create spending tx
|
|
value = int(value - self.relayfee * COIN)
|
|
tx0 = CTransaction()
|
|
tx0.vin.append(CTxIn(COutPoint(int(txid, 16), 0)))
|
|
tx0.vout.append(CTxOut(value, CScript([OP_TRUE, OP_DROP] * 15 + [OP_TRUE])))
|
|
tx0.rehash()
|
|
tx0_hex = tx0.serialize().hex()
|
|
|
|
# This tx isn't valid yet
|
|
assert not softfork_active(self.nodes[0], 'dip0020')
|
|
assert_raises_rpc_error(-26, DISABLED_OPCODE_ERROR, self.node.sendrawtransaction, tx0_hex)
|
|
|
|
# Generate enough blocks to activate DIP0020 opcodes
|
|
self.node.generate(98)
|
|
assert softfork_active(self.nodes[0], 'dip0020')
|
|
|
|
# Still need 1 more block for mempool to accept new opcodes
|
|
assert_raises_rpc_error(-26, DISABLED_OPCODE_ERROR, self.node.sendrawtransaction, tx0_hex)
|
|
self.node.generate(1)
|
|
|
|
# Should be spendable now
|
|
tx0id = self.node.sendrawtransaction(tx0_hex)
|
|
assert tx0id in set(self.node.getrawmempool())
|
|
|
|
|
|
if __name__ == '__main__':
|
|
DIP0020ActivationTest().main()
|