mirror of
https://github.com/dashpay/dash.git
synced 2024-12-27 21:12:48 +01:00
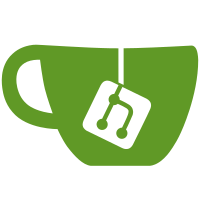
* Merge #7506: Use CCoinControl selection in CWallet::FundTransactiond6cc6a1
Use CCoinControl selection in CWallet::FundTransaction (João Barbosa) * Merge #7732: [Qt] Debug window: replace "Build date" with "Datadir"fc737d1
[Qt] remove unused formatBuildDate method (Jonas Schnelli)4856f1d
[Qt] Debug window: replace "Build date" with "Datadir" (Jonas Schnelli) * Merge #7707: [RPC][QT] UI support for abandoned transactions8efed3b
[Qt] Support for abandoned/abandoning transactions (Jonas Schnelli) * Merge #7688: List solvability in listunspent output and improve helpc3932b3
List solvability in listunspent output and improve help (Pieter Wuille) * Merge #8006: Qt: Add option to disable the system tray icon8b0e497
Qt: Add option to hide the system tray icon (Tyler Hardin) * Merge #8073: qt: askpassphrasedialog: Clear pass fields on accept02ce2a3
qt: askpassphrasedialog: Clear pass fields on accept (Pavel Vasin) * Merge #8231: [Qt] fix a bug where the SplashScreen will not be hidden during startupb3e1348
[Qt] fix a bug where the SplashScreen will not be hidden during startup (Jonas Schnelli) * Merge #8257: Do not ask a UI question from bitcoind1acf1db
Do not ask a UI question from bitcoind (Pieter Wuille) * Merge #8463: [qt] Remove Priority from coincontrol dialogfa8dd78
[qt] Remove Priority from coincontrol dialog (MarcoFalke) * Merge #8678: [Qt][CoinControl] fix UI bug that could result in paying unexpected fee0480293
[Qt][CoinControl] fix UI bug that could result in paying unexpected fee (Jonas Schnelli) * Merge #8672: Qt: Show transaction size in transaction details windowc015634
qt: Adding transaction size to transaction details window (Hampus Sjöberg) \-- merge fix for s/size/total size/fdf82fb
Adding method GetTotalSize() to CTransaction (Hampus Sjöberg) * Merge #8371: [Qt] Add out-of-sync modal info layer08827df
[Qt] modalinfolayer: removed unused comments, renamed signal, code style overhaul (Jonas Schnelli)d8b062e
[Qt] only update "amount of blocks left" when the header chain is in-sync (Jonas Schnelli)e3245b4
[Qt] add out-of-sync modal info layer (Jonas Schnelli)e47052f
[Qt] ClientModel add method to get the height of the header chain (Jonas Schnelli)a001f18
[Qt] Always pass the numBlocksChanged signal for headers tip changed (Jonas Schnelli)bd44a04
[Qt] make Out-Of-Sync warning icon clickable (Jonas Schnelli)0904c3c
[Refactor] refactor function that forms human readable text out of a timeoffset (Jonas Schnelli) * Merge #8805: Trivial: Grammar and capitalizationc9ce17b
Trivial: Grammar and capitalization (Derek Miller) * Merge #8885: gui: fix ban from qt consolecb78c60
gui: fix ban from qt console (Cory Fields) * Merge #8821: [qt] sync-overlay: Don't block during reindexfa85e86
[qt] sync-overlay: Don't show estimated number of headers left (MarcoFalke)faa4de2
[qt] sync-overlay: Don't block during reindex (MarcoFalke) * Support themes for new transaction_abandoned icon * Fix constructor call to COutput * Merge #7842: RPC: do not print minping time in getpeerinfo when no ping received yet62a6486
RPC: do not print ping info in getpeerinfo when no ping received yet, fix help (Pavel Janík) * Merge #8918: Qt: Add "Copy URI" to payment request context menu21f5a63
Qt: Add "Copy URI" to payment request context menu (Luke Dashjr) * Merge #8925: qt: Display minimum ping in debug window.1724a40
Display minimum ping in debug window. (R E Broadley) * Merge #8972: [Qt] make warnings label selectable (jonasschnelli)ef0c9ee
[Qt] make warnings label selectable (Jonas Schnelli) * Make background of warning icon transparent in modaloverlay * Merge #9088: Reduce ambiguity of warning message77cbbd9
Make warning message about wallet balance possibly being incorrect less ambiguous. (R E Broadley) * Replace Bitcoin with Dash in modal overlay * Remove clicked signals from labelWalletStatus and labelTransactionsStatus As both are really just labels, clicking on those is not possible. This is different in Bitcoin, where these labels are actually buttons. * Pull out modaloverlay show/hide into it's own if/else block and switch to time based check Also don't use masternodeSync.IsBlockchainSynced() for now as it won't report the blockchain being synced before the first block (or other MN data?) arrives. This would otherwise give the impression that sync is being stuck.
60 lines
1.8 KiB
C++
60 lines
1.8 KiB
C++
// Copyright (c) 2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2014 The Bitcoin Core developers
|
|
// Copyright (c) 2014-2017 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include "noui.h"
|
|
|
|
#include "ui_interface.h"
|
|
#include "util.h"
|
|
|
|
#include <cstdio>
|
|
#include <stdint.h>
|
|
#include <string>
|
|
|
|
static bool noui_ThreadSafeMessageBox(const std::string& message, const std::string& caption, unsigned int style)
|
|
{
|
|
bool fSecure = style & CClientUIInterface::SECURE;
|
|
style &= ~CClientUIInterface::SECURE;
|
|
|
|
std::string strCaption;
|
|
// Check for usage of predefined caption
|
|
switch (style) {
|
|
case CClientUIInterface::MSG_ERROR:
|
|
strCaption += _("Error");
|
|
break;
|
|
case CClientUIInterface::MSG_WARNING:
|
|
strCaption += _("Warning");
|
|
break;
|
|
case CClientUIInterface::MSG_INFORMATION:
|
|
strCaption += _("Information");
|
|
break;
|
|
default:
|
|
strCaption += caption; // Use supplied caption (can be empty)
|
|
}
|
|
|
|
if (!fSecure)
|
|
LogPrintf("%s: %s\n", strCaption, message);
|
|
fprintf(stderr, "%s: %s\n", strCaption.c_str(), message.c_str());
|
|
return false;
|
|
}
|
|
|
|
static bool noui_ThreadSafeQuestion(const std::string& /* ignored interactive message */, const std::string& message, const std::string& caption, unsigned int style)
|
|
{
|
|
return noui_ThreadSafeMessageBox(message, caption, style);
|
|
}
|
|
|
|
static void noui_InitMessage(const std::string& message)
|
|
{
|
|
LogPrintf("init message: %s\n", message);
|
|
}
|
|
|
|
void noui_connect()
|
|
{
|
|
// Connect dashd signal handlers
|
|
uiInterface.ThreadSafeMessageBox.connect(noui_ThreadSafeMessageBox);
|
|
uiInterface.ThreadSafeQuestion.connect(noui_ThreadSafeQuestion);
|
|
uiInterface.InitMessage.connect(noui_InitMessage);
|
|
}
|