mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 13:32:47 +01:00
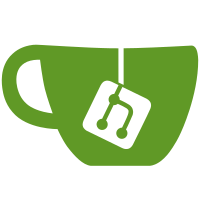
* clear trafficgraph on clear button click * set default sample height set default sample height so after clearing traffic graph have some scale * reduce available traffic graph ranges, add optimized graph data storage reduce available traffic graph ranges to 10 (5m,10m,15m,30m,1h,2h,3h,6h,12h,24h), store graph data so range change is possible, data storage contains only necessary data to create graphs for all supported ranges eg. for 10m range storage only half of 10m samples - the second half is calculated from 5m range samples, encapsulate all traffic graph related data into one class * code formatting corrections
51 lines
1.1 KiB
C++
51 lines
1.1 KiB
C++
// Copyright (c) 2011-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_QT_TRAFFICGRAPHWIDGET_H
|
|
#define BITCOIN_QT_TRAFFICGRAPHWIDGET_H
|
|
|
|
#include "trafficgraphdata.h"
|
|
|
|
#include <boost/function.hpp>
|
|
|
|
#include <QWidget>
|
|
#include <QQueue>
|
|
|
|
class ClientModel;
|
|
|
|
QT_BEGIN_NAMESPACE
|
|
class QPaintEvent;
|
|
class QTimer;
|
|
QT_END_NAMESPACE
|
|
|
|
class TrafficGraphWidget : public QWidget
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit TrafficGraphWidget(QWidget *parent = 0);
|
|
void setClientModel(ClientModel *model);
|
|
int getGraphRangeMins() const;
|
|
|
|
protected:
|
|
void paintEvent(QPaintEvent *);
|
|
|
|
public Q_SLOTS:
|
|
void updateRates();
|
|
void setGraphRangeMins(int value);
|
|
void clear();
|
|
|
|
private:
|
|
typedef boost::function<float(const TrafficSample&)> SampleChooser;
|
|
void paintPath(QPainterPath &path, const TrafficGraphData::SampleQueue &queue, SampleChooser chooser);
|
|
|
|
QTimer *timer;
|
|
float fMax;
|
|
int nMins;
|
|
ClientModel *clientModel;
|
|
TrafficGraphData trafficGraphData;
|
|
};
|
|
|
|
#endif // BITCOIN_QT_TRAFFICGRAPHWIDGET_H
|