mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
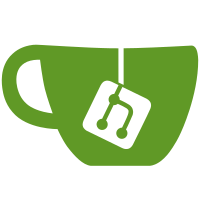
## Issue being fixed or feature implemented Requested by @QuantumExplorer for platform needs ## What was done? New rpc `gettransactionsarelocked` that returns list of txes. it does less heavy calculations and transfer less data by gRPC. ## How Has This Been Tested? ``` $ src/dash-cli gettransactionsarelocked '["e469de7994b9c1da8efd262fee8843efd7bdcab80c700dc1059c98b28f7c5c1b", "0d9fdf00c9568ff9103742b64e6b8287794633072f8824fa2c475f59e71dbace","0d3f48eebead54d640a7fc5692ddfcba619d8b49347d9a7c04586057c02dec9f"]' [ { "height": 907801, "chainlock": true }, { "height": 101, "chainlock": true }, { "height": -1, "chainlock": false } ] ``` Limiter tested by this call: ``` src/dash-cli gettransactionsarelocked '["", "","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","","",""]' | wc ``` ## Breaking Changes N/A ## Checklist: - [x] I have performed a self-review of my own code - [ ] I have commented my code, particularly in hard-to-understand areas - [ ] I have added or updated relevant unit/integration/functional/e2e tests - [ ] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone --------- Co-authored-by: pasta <pasta@dashboost.org> Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
75 lines
3.2 KiB
Python
Executable File
75 lines
3.2 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2021-2022 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.test_framework import DashTestFramework
|
|
from test_framework.util import assert_equal, assert_raises_rpc_error
|
|
|
|
'''
|
|
rpc_verifychainlock.py
|
|
|
|
Test the following RPC:
|
|
- gettxchainlocks
|
|
- verifychainlock
|
|
|
|
'''
|
|
|
|
|
|
class RPCVerifyChainLockTest(DashTestFramework):
|
|
def set_test_params(self):
|
|
# -whitelist is needed to avoid the trickling logic on node0
|
|
self.set_dash_test_params(5, 3, [["-whitelist=127.0.0.1"], [], [], [], []], fast_dip3_enforcement=True)
|
|
self.set_dash_llmq_test_params(3, 2)
|
|
|
|
def run_test(self):
|
|
node0 = self.nodes[0]
|
|
node1 = self.nodes[1]
|
|
self.activate_dip8()
|
|
self.nodes[0].sporkupdate("SPORK_17_QUORUM_DKG_ENABLED", 0)
|
|
self.wait_for_sporks_same()
|
|
self.mine_quorum()
|
|
self.wait_for_chainlocked_block(node0, node0.generate(1)[0])
|
|
chainlock = node0.getbestchainlock()
|
|
block_hash = chainlock["blockhash"]
|
|
height = chainlock["height"]
|
|
chainlock_signature = chainlock["signature"]
|
|
# No "blockHeight"
|
|
assert node0.verifychainlock(block_hash, chainlock_signature)
|
|
# Invalid "blockHeight"
|
|
assert not node0.verifychainlock(block_hash, chainlock_signature, 0)
|
|
# Valid "blockHeight"
|
|
assert node0.verifychainlock(block_hash, chainlock_signature, height)
|
|
# Invalid "blockHash"
|
|
assert not node0.verifychainlock(node0.getblockhash(0), chainlock_signature, height)
|
|
self.wait_for_chainlocked_block_all_nodes(block_hash)
|
|
# Isolate node1, mine a block on node0 and wait for its ChainLock
|
|
node1.setnetworkactive(False)
|
|
node0.generate(1)
|
|
self.wait_for_chainlocked_block(node0, node0.generate(1)[0])
|
|
chainlock = node0.getbestchainlock()
|
|
assert chainlock != node1.getbestchainlock()
|
|
block_hash = chainlock["blockhash"]
|
|
height = chainlock["height"]
|
|
chainlock_signature = chainlock["signature"]
|
|
# Now it should verify on node0 without "blockHeight" but fail on node1
|
|
assert node0.verifychainlock(block_hash, chainlock_signature)
|
|
assert_raises_rpc_error(-32603, "blockHash not found", node1.verifychainlock, block_hash, chainlock_signature)
|
|
# But they should still both verify with the height provided
|
|
assert node0.verifychainlock(block_hash, chainlock_signature, height)
|
|
assert node1.verifychainlock(block_hash, chainlock_signature, height)
|
|
|
|
node1.generate(1)
|
|
height1 = node1.getblockcount()
|
|
tx0 = node0.getblock(node0.getbestblockhash())['tx'][0]
|
|
tx1 = node1.getblock(node1.getbestblockhash())['tx'][0]
|
|
locks0 = node0.gettxchainlocks([tx0, tx1])
|
|
locks1 = node1.gettxchainlocks([tx0, tx1])
|
|
unknown_cl_helper = {'height': -1, 'chainlock': False}
|
|
assert_equal(locks0, [{'height': height, 'chainlock': True}, unknown_cl_helper])
|
|
assert_equal(locks1, [unknown_cl_helper, {'height': height1, 'chainlock': False}])
|
|
|
|
|
|
if __name__ == '__main__':
|
|
RPCVerifyChainLockTest().main()
|