mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 21:42:47 +01:00
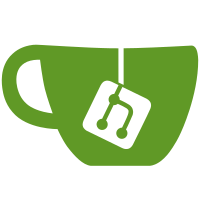
78e407ad0c26190a22de1bc8ed900164a44a36c3 GetKeyBirthTimes should return key ids, not destinations (Gregory Sanders)
70946e7fee54323ce6a5ea8aeb377e2c7c790bc6 Replace CScriptID and CKeyID in CTxDestination with dedicated types (Gregory Sanders)
Pull request description:
The current usage seems to be an overloading of meanings. `CScriptID` is used in the wallet as a lookup key, as well as a destination, and `CKeyID` likewise. Instead, have all destinations be dedicated types.
New types:
`CScriptID`->`ScriptHash`
`CKeyID`->`PKHash`
ACKs for commit 78e407:
ryanofsky:
utACK 78e407ad0c26190a22de1bc8ed900164a44a36c3. Only changes are removing extra CScriptID()s and fixing the test case.
Sjors:
utACK 78e407a
meshcollider:
utACK 78e407ad0c
Tree-SHA512: 437f59fc3afb83a40540da3351507aef5aed44e3a7f15b01ddad6226854edeee762ff0b0ef336fe3654c4cd99a205cef175211de8b639abe1130c8a6313337b9
243 lines
9.7 KiB
C++
243 lines
9.7 KiB
C++
// Copyright (c) 2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2019 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <rpc/rawtransaction_util.h>
|
|
|
|
#include <coins.h>
|
|
#include <core_io.h>
|
|
#include <interfaces/chain.h>
|
|
#include <key_io.h>
|
|
#include <policy/policy.h>
|
|
#include <primitives/transaction.h>
|
|
#include <rpc/request.h>
|
|
#include <rpc/util.h>
|
|
#include <script/signingprovider.h>
|
|
#include <univalue.h>
|
|
#include <util/strencodings.h>
|
|
#include <validation.h>
|
|
#include <txmempool.h>
|
|
|
|
CMutableTransaction ConstructTransaction(const UniValue& inputs_in, const UniValue& outputs_in, const UniValue& locktime)
|
|
{
|
|
if (inputs_in.isNull() || outputs_in.isNull())
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, arguments 1 and 2 must be non-null");
|
|
|
|
UniValue inputs = inputs_in.get_array();
|
|
const bool outputs_is_obj = outputs_in.isObject();
|
|
UniValue outputs = outputs_is_obj ? outputs_in.get_obj() : outputs_in.get_array();
|
|
|
|
CMutableTransaction rawTx;
|
|
if (!locktime.isNull()) {
|
|
int64_t nLockTime = locktime.get_int64();
|
|
if (nLockTime < 0 || nLockTime > LOCKTIME_MAX)
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, locktime out of range");
|
|
rawTx.nLockTime = nLockTime;
|
|
}
|
|
|
|
for (unsigned int idx = 0; idx < inputs.size(); idx++) {
|
|
const UniValue& input = inputs[idx];
|
|
const UniValue& o = input.get_obj();
|
|
|
|
uint256 txid = ParseHashO(o, "txid");
|
|
|
|
const UniValue& vout_v = find_value(o, "vout");
|
|
if (!vout_v.isNum())
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, missing vout key");
|
|
int nOutput = vout_v.get_int();
|
|
if (nOutput < 0)
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, vout cannot be negative");
|
|
|
|
uint32_t nSequence = (rawTx.nLockTime ? CTxIn::SEQUENCE_FINAL - 1 : CTxIn::SEQUENCE_FINAL);
|
|
|
|
// set the sequence number if passed in the parameters object
|
|
const UniValue& sequenceObj = find_value(o, "sequence");
|
|
if (sequenceObj.isNum()) {
|
|
int64_t seqNr64 = sequenceObj.get_int64();
|
|
if (seqNr64 < 0 || seqNr64 > CTxIn::SEQUENCE_FINAL)
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, sequence number is out of range");
|
|
else
|
|
nSequence = (uint32_t)seqNr64;
|
|
}
|
|
|
|
CTxIn in(COutPoint(txid, nOutput), CScript(), nSequence);
|
|
|
|
rawTx.vin.push_back(in);
|
|
}
|
|
|
|
if (!outputs_is_obj) {
|
|
// Translate array of key-value pairs into dict
|
|
UniValue outputs_dict = UniValue(UniValue::VOBJ);
|
|
for (size_t i = 0; i < outputs.size(); ++i) {
|
|
const UniValue& output = outputs[i];
|
|
if (!output.isObject()) {
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, key-value pair not an object as expected");
|
|
}
|
|
if (output.size() != 1) {
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, key-value pair must contain exactly one key");
|
|
}
|
|
outputs_dict.pushKVs(output);
|
|
}
|
|
outputs = std::move(outputs_dict);
|
|
}
|
|
|
|
// Duplicate checking
|
|
std::set<CTxDestination> destinations;
|
|
bool has_data{false};
|
|
|
|
for (const std::string& name_ : outputs.getKeys()) {
|
|
if (name_ == "data") {
|
|
if (has_data) {
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Invalid parameter, duplicate key: data");
|
|
}
|
|
has_data = true;
|
|
std::vector<unsigned char> data = ParseHexV(outputs[name_].getValStr(), "Data");
|
|
|
|
CTxOut out(0, CScript() << OP_RETURN << data);
|
|
rawTx.vout.push_back(out);
|
|
} else {
|
|
CTxDestination destination = DecodeDestination(name_);
|
|
if (!IsValidDestination(destination)) {
|
|
throw JSONRPCError(RPC_INVALID_ADDRESS_OR_KEY, std::string("Invalid Dash address: ") + name_);
|
|
}
|
|
|
|
if (!destinations.insert(destination).second) {
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, std::string("Invalid parameter, duplicated address: ") + name_);
|
|
}
|
|
|
|
CScript scriptPubKey = GetScriptForDestination(destination);
|
|
CAmount nAmount = AmountFromValue(outputs[name_]);
|
|
|
|
CTxOut out(nAmount, scriptPubKey);
|
|
rawTx.vout.push_back(out);
|
|
}
|
|
}
|
|
|
|
return rawTx;
|
|
}
|
|
|
|
/** Pushes a JSON object for script verification or signing errors to vErrorsRet. */
|
|
static void TxInErrorToJSON(const CTxIn& txin, UniValue& vErrorsRet, const std::string& strMessage)
|
|
{
|
|
UniValue entry(UniValue::VOBJ);
|
|
entry.pushKV("txid", txin.prevout.hash.ToString());
|
|
entry.pushKV("vout", (uint64_t)txin.prevout.n);
|
|
entry.pushKV("scriptSig", HexStr(txin.scriptSig));
|
|
entry.pushKV("sequence", (uint64_t)txin.nSequence);
|
|
entry.pushKV("error", strMessage);
|
|
vErrorsRet.push_back(entry);
|
|
}
|
|
|
|
void ParsePrevouts(const UniValue& prevTxsUnival, FillableSigningProvider* keystore, std::map<COutPoint, Coin>& coins)
|
|
{
|
|
if (!prevTxsUnival.isNull()) {
|
|
UniValue prevTxs = prevTxsUnival.get_array();
|
|
for (unsigned int idx = 0; idx < prevTxs.size(); ++idx) {
|
|
const UniValue& p = prevTxs[idx];
|
|
if (!p.isObject()) {
|
|
throw JSONRPCError(RPC_DESERIALIZATION_ERROR, "expected object with {\"txid'\",\"vout\",\"scriptPubKey\"}");
|
|
}
|
|
|
|
UniValue prevOut = p.get_obj();
|
|
|
|
RPCTypeCheckObj(prevOut,
|
|
{
|
|
{"txid", UniValueType(UniValue::VSTR)},
|
|
{"vout", UniValueType(UniValue::VNUM)},
|
|
{"scriptPubKey", UniValueType(UniValue::VSTR)},
|
|
});
|
|
|
|
uint256 txid = ParseHashO(prevOut, "txid");
|
|
|
|
int nOut = find_value(prevOut, "vout").get_int();
|
|
if (nOut < 0) {
|
|
throw JSONRPCError(RPC_DESERIALIZATION_ERROR, "vout cannot be negative");
|
|
}
|
|
|
|
COutPoint out(txid, nOut);
|
|
std::vector<unsigned char> pkData(ParseHexO(prevOut, "scriptPubKey"));
|
|
CScript scriptPubKey(pkData.begin(), pkData.end());
|
|
|
|
{
|
|
auto coin = coins.find(out);
|
|
if (coin != coins.end() && !coin->second.IsSpent() && coin->second.out.scriptPubKey != scriptPubKey) {
|
|
std::string err("Previous output scriptPubKey mismatch:\n");
|
|
err = err + ScriptToAsmStr(coin->second.out.scriptPubKey) + "\nvs:\n"+
|
|
ScriptToAsmStr(scriptPubKey);
|
|
throw JSONRPCError(RPC_DESERIALIZATION_ERROR, err);
|
|
}
|
|
Coin newcoin;
|
|
newcoin.out.scriptPubKey = scriptPubKey;
|
|
newcoin.out.nValue = 0;
|
|
if (prevOut.exists("amount")) {
|
|
newcoin.out.nValue = AmountFromValue(find_value(prevOut, "amount"));
|
|
}
|
|
newcoin.nHeight = 1;
|
|
coins[out] = std::move(newcoin);
|
|
}
|
|
|
|
// if redeemScript and private keys were given, add redeemScript to the keystore so it can be signed
|
|
const bool is_p2sh = scriptPubKey.IsPayToScriptHash();
|
|
if (keystore && is_p2sh) {
|
|
RPCTypeCheckObj(prevOut,
|
|
{
|
|
{"redeemScript", UniValueType(UniValue::VSTR)},
|
|
});
|
|
UniValue rs = find_value(prevOut, "redeemScript");
|
|
if (rs.isNull()) {
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "Missing redeemScript");
|
|
}
|
|
|
|
std::vector<unsigned char> scriptData(ParseHexV(rs, "redeemScript"));
|
|
CScript script(scriptData.begin(), scriptData.end());
|
|
keystore->AddCScript(script);
|
|
|
|
if (is_p2sh) {
|
|
const CTxDestination p2sh{ScriptHash(script)};
|
|
if (scriptPubKey == GetScriptForDestination(p2sh)) {
|
|
// traditional p2sh; arguably an error if
|
|
// we got here with rs.IsNull(), because
|
|
// that means the p2sh script was specified
|
|
// via witnessScript param, but for now
|
|
// we'll just quietly accept it
|
|
} else {
|
|
// otherwise, can't generate scriptPubKey from
|
|
// either script, so we got unusable parameters
|
|
throw JSONRPCError(RPC_INVALID_PARAMETER, "redeemScript does not match scriptPubKey");
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
void SignTransaction(CMutableTransaction& mtx, const SigningProvider* keystore, const std::map<COutPoint, Coin>& coins, const UniValue& hashType, UniValue& result)
|
|
{
|
|
int nHashType = ParseSighashString(hashType);
|
|
|
|
// Script verification errors
|
|
std::map<int, std::string> input_errors;
|
|
|
|
bool complete = SignTransaction(mtx, keystore, coins, nHashType, input_errors);
|
|
SignTransactionResultToJSON(mtx, complete, coins, input_errors, result);
|
|
}
|
|
|
|
void SignTransactionResultToJSON(CMutableTransaction& mtx, bool complete, const std::map<COutPoint, Coin>& coins, const std::map<int, std::string>& input_errors, UniValue& result)
|
|
{
|
|
// Make errors UniValue
|
|
UniValue vErrors(UniValue::VARR);
|
|
for (const auto& err_pair : input_errors) {
|
|
TxInErrorToJSON(mtx.vin.at(err_pair.first), vErrors, err_pair.second);
|
|
}
|
|
|
|
result.pushKV("hex", EncodeHexTx(CTransaction(mtx)));
|
|
result.pushKV("complete", complete);
|
|
if (!vErrors.empty()) {
|
|
if (result.exists("errors")) {
|
|
vErrors.push_backV(result["errors"].getValues());
|
|
}
|
|
result.pushKV("errors", vErrors);
|
|
}
|
|
}
|