mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
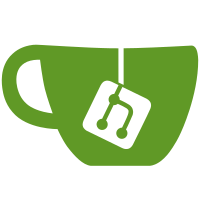
fdf82ba18 Update all subprocess.check_output functions in CI scripts to be Python 3.4 compatible (Graham Krizek) Pull request description: CI is failing the `lint` stage on every Cron run (regular PR/Push runs still pass). The failure was introduced in 74ce326 and has been broken since. The Python version running in CI was downgraded to 3.4 from 3.6. There were a couple files that were using the `encoding` argument in the `subprocess.check_output` function. This was introduced in Python 3.6 and therefore broke the scripts that were using it. The `universal_newlines` argument was used as well, but in order to use it we must be able to set encoding because of issues on some BSD systems. To get CI to pass, I removed all `universal_newline` and `encoding` args to the `check_ouput` function. Then I decoded all `check_output` return values. This should keep the same behavior but be Python 3.4 compatible. Tree-SHA512: f5e5885e98cf4777be9cc254446a873eedb03bdccbd8e06772a964db95e9fcf46736aa9cdcab1d8f123ea9f4947ed6020679898d8b2f47ffb1d94c21a4b08209
53 lines
2.0 KiB
Python
Executable File
53 lines
2.0 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2015 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
'''
|
|
This checks if all command line args are documented.
|
|
Return value is 0 to indicate no error.
|
|
|
|
Author: @MarcoFalke
|
|
'''
|
|
|
|
from subprocess import check_output
|
|
import re
|
|
import sys
|
|
|
|
FOLDER_GREP = 'src'
|
|
FOLDER_TEST = 'src/test/'
|
|
REGEX_ARG = '(?:ForceSet|SoftSet|Get|Is)(?:Bool)?Args?(?:Set)?\("(-[^"]+)"'
|
|
REGEX_DOC = 'AddArg\("(-[^"=]+?)(?:=|")'
|
|
CMD_ROOT_DIR = '`git rev-parse --show-toplevel`/{}'.format(FOLDER_GREP)
|
|
CMD_GREP_ARGS = r"git grep --perl-regexp '{}' -- {} ':(exclude){}'".format(REGEX_ARG, CMD_ROOT_DIR, FOLDER_TEST)
|
|
CMD_GREP_DOCS = r"git grep --perl-regexp '{}' {}".format(REGEX_DOC, CMD_ROOT_DIR)
|
|
# list unsupported, deprecated and duplicate args as they need no documentation
|
|
SET_DOC_OPTIONAL = set(['-rpcssl', '-benchmark', '-h', '-help', '-socks', '-tor', '-debugnet', '-whitelistalwaysrelay', '-blockminsize', '-dbcrashratio', '-forcecompactdb'])
|
|
|
|
|
|
def main():
|
|
if sys.version_info >= (3, 6):
|
|
used = check_output(CMD_GREP_ARGS, shell=True, universal_newlines=True, encoding='utf8')
|
|
docd = check_output(CMD_GREP_DOCS, shell=True, universal_newlines=True, encoding='utf8')
|
|
else:
|
|
used = check_output(CMD_GREP_ARGS, shell=True).decode('utf8').strip()
|
|
docd = check_output(CMD_GREP_DOCS, shell=True).decode('utf8').strip()
|
|
|
|
args_used = set(re.findall(re.compile(REGEX_ARG), used))
|
|
args_docd = set(re.findall(re.compile(REGEX_DOC), docd)).union(SET_DOC_OPTIONAL)
|
|
args_need_doc = args_used.difference(args_docd)
|
|
args_unknown = args_docd.difference(args_used)
|
|
|
|
print("Args used : {}".format(len(args_used)))
|
|
print("Args documented : {}".format(len(args_docd)))
|
|
print("Args undocumented: {}".format(len(args_need_doc)))
|
|
print(args_need_doc)
|
|
print("Args unknown : {}".format(len(args_unknown)))
|
|
print(args_unknown)
|
|
|
|
sys.exit(len(args_need_doc))
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|