mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
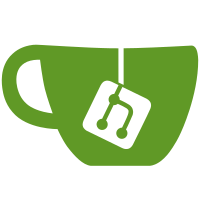
faa7d8a3f7cba02eca7e247108a6b98ea9daf373 util: Add SaturatingAdd helper (MarcoFalke) Pull request description: Seems good to have this in the repo, as it might be needed to write cleaner code. For example: * https://github.com/bitcoin/bitcoin/pull/24090#issuecomment-1019948200 * https://github.com/bitcoin/bitcoin/pull/23418#discussion_r744953272 * ... ACKs for top commit: MarcoFalke: Added a test. Should be trivial to re-ACK with `git range-diff bitcoin-core/master fa90189cbf faa7d8a3f7` klementtan: reACK faa7d8a3f7 vasild: ACK faa7d8a3f7cba02eca7e247108a6b98ea9daf373 Tree-SHA512: d0e6efdba7dfcbdd16ab4539a7f5e45a97d17792e42586c3c52caaae3fc70612dc9e364359658de5de5718fb8c2a765a59ceb2230098355394fa067a9732bc2a
51 lines
1.4 KiB
C++
51 lines
1.4 KiB
C++
// Copyright (c) 2021 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_UTIL_OVERFLOW_H
|
|
#define BITCOIN_UTIL_OVERFLOW_H
|
|
|
|
#include <limits>
|
|
#include <optional>
|
|
#include <type_traits>
|
|
|
|
template <class T>
|
|
[[nodiscard]] bool AdditionOverflow(const T i, const T j) noexcept
|
|
{
|
|
static_assert(std::is_integral<T>::value, "Integral required.");
|
|
if constexpr (std::numeric_limits<T>::is_signed) {
|
|
return (i > 0 && j > std::numeric_limits<T>::max() - i) ||
|
|
(i < 0 && j < std::numeric_limits<T>::min() - i);
|
|
}
|
|
return std::numeric_limits<T>::max() - i < j;
|
|
}
|
|
|
|
template <class T>
|
|
[[nodiscard]] std::optional<T> CheckedAdd(const T i, const T j) noexcept
|
|
{
|
|
if (AdditionOverflow(i, j)) {
|
|
return std::nullopt;
|
|
}
|
|
return i + j;
|
|
}
|
|
|
|
template <class T>
|
|
[[nodiscard]] T SaturatingAdd(const T i, const T j) noexcept
|
|
{
|
|
if constexpr (std::numeric_limits<T>::is_signed) {
|
|
if (i > 0 && j > std::numeric_limits<T>::max() - i) {
|
|
return std::numeric_limits<T>::max();
|
|
}
|
|
if (i < 0 && j < std::numeric_limits<T>::min() - i) {
|
|
return std::numeric_limits<T>::min();
|
|
}
|
|
} else {
|
|
if (std::numeric_limits<T>::max() - i < j) {
|
|
return std::numeric_limits<T>::max();
|
|
}
|
|
}
|
|
return i + j;
|
|
}
|
|
|
|
#endif // BITCOIN_UTIL_OVERFLOW_H
|