mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
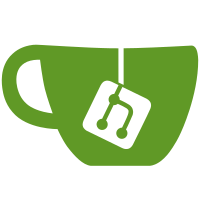
that's a result of: contrib/devtools/copyright_header.py update ./ it is not scripted diff, because it works differentlly on my localhost and in CI: CI doesn't want to use git commit date which is mocked to 30th Dec of 2023
79 lines
1.7 KiB
C++
79 lines
1.7 KiB
C++
// Copyright (c) 2018-2023 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <evo/evodb.h>
|
|
|
|
#include <uint256.h>
|
|
|
|
CEvoDBScopedCommitter::CEvoDBScopedCommitter(CEvoDB &_evoDB) :
|
|
evoDB(_evoDB)
|
|
{
|
|
}
|
|
|
|
CEvoDBScopedCommitter::~CEvoDBScopedCommitter()
|
|
{
|
|
if (!didCommitOrRollback)
|
|
Rollback();
|
|
}
|
|
|
|
void CEvoDBScopedCommitter::Commit()
|
|
{
|
|
assert(!didCommitOrRollback);
|
|
didCommitOrRollback = true;
|
|
evoDB.CommitCurTransaction();
|
|
}
|
|
|
|
void CEvoDBScopedCommitter::Rollback()
|
|
{
|
|
assert(!didCommitOrRollback);
|
|
didCommitOrRollback = true;
|
|
evoDB.RollbackCurTransaction();
|
|
}
|
|
|
|
CEvoDB::CEvoDB(size_t nCacheSize, bool fMemory, bool fWipe) :
|
|
db(fMemory ? "" : (GetDataDir() / "evodb"), nCacheSize, fMemory, fWipe),
|
|
rootBatch(db),
|
|
rootDBTransaction(db, rootBatch),
|
|
curDBTransaction(rootDBTransaction, rootDBTransaction)
|
|
{
|
|
}
|
|
|
|
void CEvoDB::CommitCurTransaction()
|
|
{
|
|
LOCK(cs);
|
|
curDBTransaction.Commit();
|
|
}
|
|
|
|
void CEvoDB::RollbackCurTransaction()
|
|
{
|
|
LOCK(cs);
|
|
curDBTransaction.Clear();
|
|
}
|
|
|
|
bool CEvoDB::CommitRootTransaction()
|
|
{
|
|
LOCK(cs);
|
|
assert(curDBTransaction.IsClean());
|
|
rootDBTransaction.Commit();
|
|
bool ret = db.WriteBatch(rootBatch);
|
|
rootBatch.Clear();
|
|
return ret;
|
|
}
|
|
|
|
bool CEvoDB::VerifyBestBlock(const uint256& hash)
|
|
{
|
|
// Make sure evodb is consistent.
|
|
// If we already have best block hash saved, the previous block should match it.
|
|
uint256 hashBestBlock;
|
|
if (!Read(EVODB_BEST_BLOCK, hashBestBlock)) {
|
|
return false;
|
|
}
|
|
return hashBestBlock == hash;
|
|
}
|
|
|
|
void CEvoDB::WriteBestBlock(const uint256& hash)
|
|
{
|
|
Write(EVODB_BEST_BLOCK, hash);
|
|
}
|