mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 21:42:47 +01:00
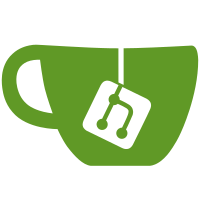
78e407ad0c26190a22de1bc8ed900164a44a36c3 GetKeyBirthTimes should return key ids, not destinations (Gregory Sanders)
70946e7fee54323ce6a5ea8aeb377e2c7c790bc6 Replace CScriptID and CKeyID in CTxDestination with dedicated types (Gregory Sanders)
Pull request description:
The current usage seems to be an overloading of meanings. `CScriptID` is used in the wallet as a lookup key, as well as a destination, and `CKeyID` likewise. Instead, have all destinations be dedicated types.
New types:
`CScriptID`->`ScriptHash`
`CKeyID`->`PKHash`
ACKs for commit 78e407:
ryanofsky:
utACK 78e407ad0c26190a22de1bc8ed900164a44a36c3. Only changes are removing extra CScriptID()s and fixing the test case.
Sjors:
utACK 78e407a
meshcollider:
utACK 78e407ad0c
Tree-SHA512: 437f59fc3afb83a40540da3351507aef5aed44e3a7f15b01ddad6226854edeee762ff0b0ef336fe3654c4cd99a205cef175211de8b639abe1130c8a6313337b9
78 lines
2.8 KiB
C++
78 lines
2.8 KiB
C++
// Copyright (c) 2017-2018 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <chainparams.h>
|
|
#include <index/txindex.h>
|
|
#include <script/standard.h>
|
|
#include <test/util/setup_common.h>
|
|
#include <util/time.h>
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
BOOST_AUTO_TEST_SUITE(txindex_tests)
|
|
|
|
BOOST_FIXTURE_TEST_CASE(txindex_initial_sync, TestChain100Setup)
|
|
{
|
|
TxIndex txindex(1 << 20, true);
|
|
|
|
CTransactionRef tx_disk;
|
|
uint256 block_hash;
|
|
|
|
// Transaction should not be found in the index before it is started.
|
|
for (const auto& txn : m_coinbase_txns) {
|
|
BOOST_CHECK(!txindex.FindTx(txn->GetHash(), block_hash, tx_disk));
|
|
}
|
|
|
|
// BlockUntilSyncedToCurrentChain should return false before txindex is started.
|
|
BOOST_CHECK(!txindex.BlockUntilSyncedToCurrentChain());
|
|
|
|
txindex.Start();
|
|
|
|
// Allow tx index to catch up with the block index.
|
|
constexpr int64_t timeout_ms = 10 * 1000;
|
|
int64_t time_start = GetTimeMillis();
|
|
while (!txindex.BlockUntilSyncedToCurrentChain()) {
|
|
BOOST_REQUIRE(time_start + timeout_ms > GetTimeMillis());
|
|
UninterruptibleSleep(std::chrono::milliseconds{100});
|
|
}
|
|
|
|
// Check that txindex excludes genesis block transactions.
|
|
const CBlock& genesis_block = Params().GenesisBlock();
|
|
for (const auto& txn : genesis_block.vtx) {
|
|
BOOST_CHECK(!txindex.FindTx(txn->GetHash(), block_hash, tx_disk));
|
|
}
|
|
|
|
// Check that txindex has all txs that were in the chain before it started.
|
|
for (const auto& txn : m_coinbase_txns) {
|
|
if (!txindex.FindTx(txn->GetHash(), block_hash, tx_disk)) {
|
|
BOOST_ERROR("FindTx failed");
|
|
} else if (tx_disk->GetHash() != txn->GetHash()) {
|
|
BOOST_ERROR("Read incorrect tx");
|
|
}
|
|
}
|
|
|
|
// Check that new transactions in new blocks make it into the index.
|
|
for (int i = 0; i < 10; i++) {
|
|
CScript coinbase_script_pub_key = GetScriptForDestination(PKHash(coinbaseKey.GetPubKey()));
|
|
std::vector<CMutableTransaction> no_txns;
|
|
const CBlock& block = CreateAndProcessBlock(no_txns, coinbase_script_pub_key);
|
|
const CTransaction& txn = *block.vtx[0];
|
|
|
|
BOOST_CHECK(txindex.BlockUntilSyncedToCurrentChain());
|
|
if (!txindex.FindTx(txn.GetHash(), block_hash, tx_disk)) {
|
|
BOOST_ERROR("FindTx failed");
|
|
} else if (tx_disk->GetHash() != txn.GetHash()) {
|
|
BOOST_ERROR("Read incorrect tx");
|
|
}
|
|
}
|
|
|
|
// shutdown sequence (c.f. Shutdown() in init.cpp)
|
|
txindex.Stop();
|
|
|
|
// Let scheduler events finish running to avoid accessing any memory related to txindex after it is destructed
|
|
SyncWithValidationInterfaceQueue();
|
|
}
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|