mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
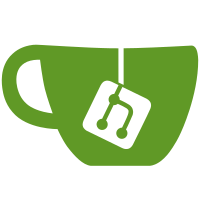
dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64 refactor: test: use _ variable for unused loop counters (Sebastian Falbesoner)
Pull request description:
This tiny PR substitutes Python loops in the form of `for x in range(N): ...` by `for _ in range(N): ...` where applicable. The idea is indicating to the reader that a block (or statement, in list comprehensions) is just repeated N times, and that the loop counter is not used in the body, hence using the throwaway variable. This is already done quite often in the current tests (see e.g. `$ git grep "for _ in range("`). Another alternative would be using `itertools.repeat` (according to Python core developer Raymond Hettinger it's [even faster](https://twitter.com/raymondh/status/1144527183341375488)), but that doesn't seem to be widespread in use and I'm not sure about a readability increase.
The only drawback I see is that whenever one wants to debug loop iterations, one would need to introduce a loop variable again. Reviewing this is basically a no-brainer, since tests would fail immediately if a a substitution has taken place on a loop where the variable is used.
Instances to replace were found by `$ git grep "for.*in range("` and manually checked.
ACKs for top commit:
darosior:
ACK dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64
instagibbs:
manual inspection ACK dac7a111bd
practicalswift:
ACK dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64 -- the updated code is easier to reason about since the throwaway nature of a variable is expressed explicitly (using the Pythonic `_` idiom) instead of implicitly. Explicit is better than implicit was we all know by now :)
Tree-SHA512: 5f43ded9ce14e5e00b3876ec445b90acda1842f813149ae7bafa93f3ac3d510bb778e2c701187fd2c73585e6b87797bb2d2987139bd1a9ba7d58775a59392406
98 lines
4.4 KiB
Python
Executable File
98 lines
4.4 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2015-2022 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.test_framework import DashTestFramework
|
|
|
|
'''
|
|
feature_llmq_dkgerrors.py
|
|
|
|
Simulate and check DKG errors
|
|
|
|
'''
|
|
|
|
class LLMQDKGErrors(DashTestFramework):
|
|
def set_test_params(self):
|
|
self.set_dash_test_params(4, 3, [["-whitelist=127.0.0.1"]] * 4, fast_dip3_enforcement=True)
|
|
|
|
def run_test(self):
|
|
self.activate_dip8()
|
|
|
|
self.nodes[0].sporkupdate("SPORK_17_QUORUM_DKG_ENABLED", 0)
|
|
self.wait_for_sporks_same()
|
|
|
|
self.log.info("Mine one quorum without simulating any errors")
|
|
qh = self.mine_quorum()
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, True)
|
|
|
|
self.log.info("Lets omit the contribution")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'contribution-omit', '1')
|
|
qh = self.mine_quorum(expected_contributions=2)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, False)
|
|
|
|
self.log.info("Lets lie in the contribution but provide a correct justification")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'contribution-omit', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'contribution-lie', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=2, expected_justifications=1)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, True)
|
|
|
|
self.log.info("Lets lie in the contribution and then omit the justification")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'justify-omit', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=2)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, False)
|
|
|
|
self.log.info("Heal some damage (don't get PoSe banned)")
|
|
self.heal_masternodes(33)
|
|
|
|
self.log.info("Lets lie in the contribution and then also lie in the justification")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'justify-omit', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'justify-lie', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=2, expected_justifications=1)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, False)
|
|
|
|
self.log.info("Lets lie about another MN")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'contribution-lie', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'justify-lie', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'complain-lie', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=1, expected_justifications=2)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, True)
|
|
|
|
self.log.info("Lets omit 1 premature commitments")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'complain-lie', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'commit-omit', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=0, expected_justifications=0, expected_commitments=2)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, True)
|
|
|
|
self.log.info("Lets lie in 1 premature commitments")
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'commit-omit', '0')
|
|
self.mninfo[0].node.quorum('dkgsimerror', 'commit-lie', '1')
|
|
qh = self.mine_quorum(expected_contributions=3, expected_complaints=0, expected_justifications=0, expected_commitments=2)
|
|
self.assert_member_valid(qh, self.mninfo[0].proTxHash, True)
|
|
|
|
def assert_member_valid(self, quorumHash, proTxHash, expectedValid):
|
|
q = self.nodes[0].quorum('info', 100, quorumHash, True)
|
|
for m in q['members']:
|
|
if m['proTxHash'] == proTxHash:
|
|
if expectedValid:
|
|
assert m['valid']
|
|
else:
|
|
assert not m['valid']
|
|
else:
|
|
assert m['valid']
|
|
|
|
def heal_masternodes(self, blockCount):
|
|
# We're not testing PoSe here, so lets heal the MNs :)
|
|
self.nodes[0].sporkupdate("SPORK_17_QUORUM_DKG_ENABLED", 4070908800)
|
|
self.wait_for_sporks_same()
|
|
for _ in range(blockCount):
|
|
self.bump_mocktime(1)
|
|
self.nodes[0].generate(1)
|
|
self.sync_all()
|
|
self.nodes[0].sporkupdate("SPORK_17_QUORUM_DKG_ENABLED", 0)
|
|
self.wait_for_sporks_same()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
LLMQDKGErrors().main()
|