mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
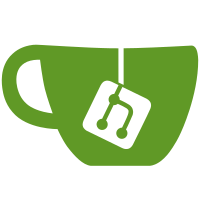
that's a result of: contrib/devtools/copyright_header.py update ./ it is not scripted diff, because it works differentlly on my localhost and in CI: CI doesn't want to use git commit date which is mocked to 30th Dec of 2023
73 lines
2.4 KiB
C++
73 lines
2.4 KiB
C++
// Copyright (c) 2014-2023 The Dash Core developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <key_io.h>
|
|
#include <hash.h>
|
|
#include <util/message.h> // For MESSAGE_MAGIC
|
|
#include <messagesigner.h>
|
|
#include <tinyformat.h>
|
|
#include <util/strencodings.h>
|
|
|
|
bool CMessageSigner::GetKeysFromSecret(const std::string& strSecret, CKey& keyRet, CPubKey& pubkeyRet)
|
|
{
|
|
keyRet = DecodeSecret(strSecret);
|
|
if (!keyRet.IsValid()) {
|
|
return false;
|
|
}
|
|
pubkeyRet = keyRet.GetPubKey();
|
|
|
|
return true;
|
|
}
|
|
|
|
bool CMessageSigner::SignMessage(const std::string& strMessage, std::vector<unsigned char>& vchSigRet, const CKey& key)
|
|
{
|
|
CHashWriter ss(SER_GETHASH, 0);
|
|
ss << MESSAGE_MAGIC;
|
|
ss << strMessage;
|
|
|
|
return CHashSigner::SignHash(ss.GetHash(), key, vchSigRet);
|
|
}
|
|
|
|
bool CMessageSigner::VerifyMessage(const CPubKey& pubkey, const std::vector<unsigned char>& vchSig, const std::string& strMessage, std::string& strErrorRet)
|
|
{
|
|
return VerifyMessage(pubkey.GetID(), vchSig, strMessage, strErrorRet);
|
|
}
|
|
|
|
bool CMessageSigner::VerifyMessage(const CKeyID& keyID, const std::vector<unsigned char>& vchSig, const std::string& strMessage, std::string& strErrorRet)
|
|
{
|
|
CHashWriter ss(SER_GETHASH, 0);
|
|
ss << MESSAGE_MAGIC;
|
|
ss << strMessage;
|
|
|
|
return CHashSigner::VerifyHash(ss.GetHash(), keyID, vchSig, strErrorRet);
|
|
}
|
|
|
|
bool CHashSigner::SignHash(const uint256& hash, const CKey& key, std::vector<unsigned char>& vchSigRet)
|
|
{
|
|
return key.SignCompact(hash, vchSigRet);
|
|
}
|
|
|
|
bool CHashSigner::VerifyHash(const uint256& hash, const CPubKey& pubkey, const std::vector<unsigned char>& vchSig, std::string& strErrorRet)
|
|
{
|
|
return VerifyHash(hash, pubkey.GetID(), vchSig, strErrorRet);
|
|
}
|
|
|
|
bool CHashSigner::VerifyHash(const uint256& hash, const CKeyID& keyID, const std::vector<unsigned char>& vchSig, std::string& strErrorRet)
|
|
{
|
|
CPubKey pubkeyFromSig;
|
|
if(!pubkeyFromSig.RecoverCompact(hash, vchSig)) {
|
|
strErrorRet = "Error recovering public key.";
|
|
return false;
|
|
}
|
|
|
|
if(pubkeyFromSig.GetID() != keyID) {
|
|
strErrorRet = strprintf("Keys don't match: pubkey=%s, pubkeyFromSig=%s, hash=%s, vchSig=%s",
|
|
keyID.ToString(), pubkeyFromSig.GetID().ToString(), hash.ToString(),
|
|
EncodeBase64(vchSig));
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|