mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
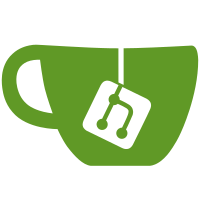
* Initial devnet * Move genesis block adding into its own method * Introduce -allowprivatenet to lift limitation on RFC1918 addresses Normally, RFC1918 (192.168.x.x/10.x.x.x/...) addresses are not allowed to be relayed. Also, masternodes won't start when the address is considered invalid. This is needed to test local devnet or regtest based networks. * Lift the requirement of minimum MN age for regtest/devnet * Implement named devnets This allows the creation of multiple independent devnets. Each one is identified by a name which is hardened into a "devnet genesis" block, which is automatically positioned at height 1. Validation rules will ensure that a node from devnet=test1 never be able to accept blocks from devnet=test2. This is done by checking the expected devnet genesis block. The genesis block of the devnet is the same as the one from regtest. This starts the devnet with a very low difficulty, allowing us to fill up needed balances for masternodes very fast. Also, the devnet name is put into the sub-version of the VERSION message. If a node connects to the wrong network, it will immediately be disconnected. * Allow to select multiple addresses from the same group in devnet/regtest The selection code normally only allows to select addresses from the same group (e.g. 192.168.x.x) once. This results in connecting to only a single node in devnet/regtest. * Show the devnet name in the title bar and on the loading screen * Add AllowMultipleAddressesFromGroup to chainparams and use it in net.cpp * Remove unused/unneeded scripts from devnet geneses creation 1. OP_RETURN not needed in input script of devnet genesis 2. genesisOutputScript was unused * Fix copy/paste error in -allowprivatenet description * Improve -devnet parameter error handling - Only allow one of -devnet, -regtest or -testnet - Only allow -devnet=name to be specified once * Use different datadir for each devnet * Fix `devnet-devnet` issue * Fix devnet splashscreen (should use testnet img) * Avoid passing devNetName around (most of the time) * Remove nMaxTipAge from CDevNetParams Not present anymore after rebase on develop
66 lines
1.8 KiB
C++
66 lines
1.8 KiB
C++
// Copyright (c) 2014-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_CHAINPARAMSBASE_H
|
|
#define BITCOIN_CHAINPARAMSBASE_H
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
/**
|
|
* CBaseChainParams defines the base parameters (shared between dash-cli and dashd)
|
|
* of a given instance of the Dash system.
|
|
*/
|
|
class CBaseChainParams
|
|
{
|
|
public:
|
|
/** BIP70 chain name strings (main, test or regtest) */
|
|
static const std::string MAIN;
|
|
static const std::string TESTNET;
|
|
static const std::string DEVNET;
|
|
static const std::string REGTEST;
|
|
|
|
const std::string& DataDir() const { return strDataDir; }
|
|
int RPCPort() const { return nRPCPort; }
|
|
|
|
protected:
|
|
CBaseChainParams() {}
|
|
|
|
int nRPCPort;
|
|
std::string strDataDir;
|
|
};
|
|
|
|
/**
|
|
* Append the help messages for the chainparams options to the
|
|
* parameter string.
|
|
*/
|
|
void AppendParamsHelpMessages(std::string& strUsage, bool debugHelp=true);
|
|
|
|
/**
|
|
* Return the currently selected parameters. This won't change after app
|
|
* startup, except for unit tests.
|
|
*/
|
|
const CBaseChainParams& BaseParams();
|
|
|
|
CBaseChainParams& BaseParams(const std::string& chain);
|
|
|
|
/** Sets the params returned by Params() to those for the given network. */
|
|
void SelectBaseParams(const std::string& chain);
|
|
|
|
/**
|
|
* Looks for -regtest, -testnet and returns the appropriate BIP70 chain name.
|
|
* @return CBaseChainParams::MAX_NETWORK_TYPES if an invalid combination is given. CBaseChainParams::MAIN by default.
|
|
*/
|
|
std::string ChainNameFromCommandLine();
|
|
|
|
std::string GetDevNetName();
|
|
|
|
/**
|
|
* Return true if SelectBaseParamsFromCommandLine() has been called to select
|
|
* a network.
|
|
*/
|
|
bool AreBaseParamsConfigured();
|
|
|
|
#endif // BITCOIN_CHAINPARAMSBASE_H
|