mirror of
https://github.com/dashpay/dash.git
synced 2024-12-29 05:49:11 +01:00
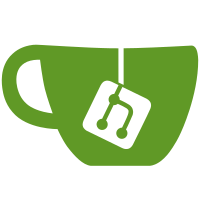
72f0060
Replace traditional for with ranged for in primitives (Dag Robole)
Pull request description:
Replace traditional for with ranged for in block and transaction primitives to improve readability
Tree-SHA512: c0fff603d2939149ca48b6aa72b59738a3658d49bd58b2d4ffbc85bdb774d8d5bb808fe526fe22bb9eb214de632834d373e2aab44f6019a83c0b09440cea6528
132 lines
4.4 KiB
C++
132 lines
4.4 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include "primitives/transaction.h"
|
|
|
|
#include "hash.h"
|
|
#include "tinyformat.h"
|
|
#include "utilstrencodings.h"
|
|
|
|
std::string COutPoint::ToString() const
|
|
{
|
|
return strprintf("COutPoint(%s, %u)", hash.ToString()/*.substr(0,10)*/, n);
|
|
}
|
|
|
|
std::string COutPoint::ToStringShort() const
|
|
{
|
|
return strprintf("%s-%u", hash.ToString().substr(0,64), n);
|
|
}
|
|
|
|
CTxIn::CTxIn(COutPoint prevoutIn, CScript scriptSigIn, uint32_t nSequenceIn)
|
|
{
|
|
prevout = prevoutIn;
|
|
scriptSig = scriptSigIn;
|
|
nSequence = nSequenceIn;
|
|
}
|
|
|
|
CTxIn::CTxIn(uint256 hashPrevTx, uint32_t nOut, CScript scriptSigIn, uint32_t nSequenceIn)
|
|
{
|
|
prevout = COutPoint(hashPrevTx, nOut);
|
|
scriptSig = scriptSigIn;
|
|
nSequence = nSequenceIn;
|
|
}
|
|
|
|
std::string CTxIn::ToString() const
|
|
{
|
|
std::string str;
|
|
str += "CTxIn(";
|
|
str += prevout.ToString();
|
|
if (prevout.IsNull())
|
|
str += strprintf(", coinbase %s", HexStr(scriptSig));
|
|
else
|
|
str += strprintf(", scriptSig=%s", HexStr(scriptSig).substr(0, 24));
|
|
if (nSequence != SEQUENCE_FINAL)
|
|
str += strprintf(", nSequence=%u", nSequence);
|
|
str += ")";
|
|
return str;
|
|
}
|
|
|
|
CTxOut::CTxOut(const CAmount& nValueIn, CScript scriptPubKeyIn, int nRoundsIn)
|
|
{
|
|
nValue = nValueIn;
|
|
scriptPubKey = scriptPubKeyIn;
|
|
nRounds = nRoundsIn;
|
|
}
|
|
|
|
std::string CTxOut::ToString() const
|
|
{
|
|
return strprintf("CTxOut(nValue=%d.%08d, scriptPubKey=%s)", nValue / COIN, nValue % COIN, HexStr(scriptPubKey).substr(0, 30));
|
|
}
|
|
|
|
CMutableTransaction::CMutableTransaction() : nVersion(CTransaction::CURRENT_VERSION), nType(TRANSACTION_NORMAL), nLockTime(0) {}
|
|
CMutableTransaction::CMutableTransaction(const CTransaction& tx) : nVersion(tx.nVersion), nType(tx.nType), vin(tx.vin), vout(tx.vout), nLockTime(tx.nLockTime), vExtraPayload(tx.vExtraPayload) {}
|
|
|
|
uint256 CMutableTransaction::GetHash() const
|
|
{
|
|
return SerializeHash(*this);
|
|
}
|
|
|
|
std::string CMutableTransaction::ToString() const
|
|
{
|
|
std::string str;
|
|
str += strprintf("CMutableTransaction(hash=%s, ver=%d, type=%d, vin.size=%u, vout.size=%u, nLockTime=%u, vExtraPayload.size=%d)\n",
|
|
GetHash().ToString().substr(0,10),
|
|
nVersion,
|
|
nType,
|
|
vin.size(),
|
|
vout.size(),
|
|
nLockTime,
|
|
vExtraPayload.size());
|
|
for (unsigned int i = 0; i < vin.size(); i++)
|
|
str += " " + vin[i].ToString() + "\n";
|
|
for (unsigned int i = 0; i < vout.size(); i++)
|
|
str += " " + vout[i].ToString() + "\n";
|
|
return str;
|
|
}
|
|
|
|
uint256 CTransaction::ComputeHash() const
|
|
{
|
|
return SerializeHash(*this);
|
|
}
|
|
|
|
/* For backward compatibility, the hash is initialized to 0. TODO: remove the need for this default constructor entirely. */
|
|
CTransaction::CTransaction() : nVersion(CTransaction::CURRENT_VERSION), nType(TRANSACTION_NORMAL), vin(), vout(), nLockTime(0), hash() {}
|
|
CTransaction::CTransaction(const CMutableTransaction &tx) : nVersion(tx.nVersion), nType(tx.nType), vin(tx.vin), vout(tx.vout), nLockTime(tx.nLockTime), vExtraPayload(tx.vExtraPayload), hash(ComputeHash()) {}
|
|
CTransaction::CTransaction(CMutableTransaction &&tx) : nVersion(tx.nVersion), nType(tx.nType), vin(std::move(tx.vin)), vout(std::move(tx.vout)), nLockTime(tx.nLockTime), vExtraPayload(tx.vExtraPayload), hash(ComputeHash()) {}
|
|
|
|
CAmount CTransaction::GetValueOut() const
|
|
{
|
|
CAmount nValueOut = 0;
|
|
for (const auto& tx_out : vout) {
|
|
nValueOut += tx_out.nValue;
|
|
if (!MoneyRange(tx_out.nValue) || !MoneyRange(nValueOut))
|
|
throw std::runtime_error(std::string(__func__) + ": value out of range");
|
|
}
|
|
return nValueOut;
|
|
}
|
|
|
|
unsigned int CTransaction::GetTotalSize() const
|
|
{
|
|
return ::GetSerializeSize(*this, SER_NETWORK, PROTOCOL_VERSION);
|
|
}
|
|
|
|
std::string CTransaction::ToString() const
|
|
{
|
|
std::string str;
|
|
str += strprintf("CTransaction(hash=%s, ver=%d, type=%d, vin.size=%u, vout.size=%u, nLockTime=%u, vExtraPayload.size=%d)\n",
|
|
GetHash().ToString().substr(0,10),
|
|
nVersion,
|
|
nType,
|
|
vin.size(),
|
|
vout.size(),
|
|
nLockTime,
|
|
vExtraPayload.size());
|
|
for (const auto& tx_in : vin)
|
|
str += " " + tx_in.ToString() + "\n";
|
|
for (const auto& tx_out : vout)
|
|
str += " " + tx_out.ToString() + "\n";
|
|
return str;
|
|
}
|