mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
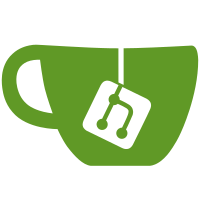
## Issue being fixed or feature implemented Given the hard fork that happened on testnet, there is now lots of the transactions that were made on the fork that is no longer valid. Some transactions could be relayed and mined again but some like coinjoin mixing won't be relayed because of 0 fee and transactions spending coinbases from the forked branch are no longer valid at all. ## What was done? Introduce `wipewallettxes` RPC and `wipetxes` command for `dash-wallet` tool to be able to get rid of some/all txes in the wallet. ## How Has This Been Tested? run tests, use rpc/command on testnet wallet ## Breaking Changes n/a ## Checklist: - [x] I have performed a self-review of my own code - [ ] I have commented my code, particularly in hard-to-understand areas - [ ] I have added or updated relevant unit/integration/functional/e2e tests - [ ] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone _(for repository code-owners and collaborators only)_
42 lines
1.8 KiB
Python
42 lines
1.8 KiB
Python
#!/usr/bin/env python3
|
|
# Copyright (c) 2023 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test transaction wiping using the wipewallettxes RPC."""
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal, assert_raises_rpc_error
|
|
|
|
|
|
class WipeWalletTxesTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 1
|
|
|
|
def skip_test_if_missing_module(self):
|
|
self.skip_if_no_wallet()
|
|
|
|
def run_test(self):
|
|
self.log.info("Test that wipewallettxes removes txes and rescanblockchain is able to recover them")
|
|
self.nodes[0].generate(101)
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[0].getnewaddress(), 1)
|
|
self.nodes[0].generate(1)
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 103)
|
|
self.nodes[0].wipewallettxes()
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 0)
|
|
self.nodes[0].rescanblockchain()
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 103)
|
|
|
|
self.log.info("Test that wipewallettxes removes txes but keeps confirmed ones when asked to")
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[0].getnewaddress(), 1)
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 104)
|
|
self.nodes[0].wipewallettxes(True)
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 103)
|
|
self.nodes[0].rescanblockchain()
|
|
assert_equal(self.nodes[0].getwalletinfo()["txcount"], 103)
|
|
assert_raises_rpc_error(-5, "Invalid or non-wallet transaction id", self.nodes[0].gettransaction, txid)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
WipeWalletTxesTest().main()
|