mirror of
https://github.com/dashpay/dash.git
synced 2024-12-27 04:52:59 +01:00
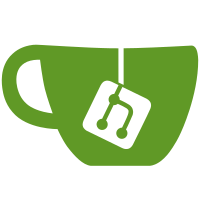
fixes a bug that would happen when an output would match an input with the same address and index, and would lead to the outputs not appearing in results.
83 lines
2.1 KiB
C
83 lines
2.1 KiB
C
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_ADDRESSINDEX_H
|
|
#define BITCOIN_ADDRESSINDEX_H
|
|
|
|
#include "uint256.h"
|
|
#include "amount.h"
|
|
|
|
struct CMempoolAddressDelta
|
|
{
|
|
int64_t time;
|
|
CAmount amount;
|
|
uint256 prevhash;
|
|
unsigned int prevout;
|
|
|
|
CMempoolAddressDelta(int64_t t, CAmount a, uint256 hash, unsigned int out) {
|
|
time = t;
|
|
amount = a;
|
|
prevhash = hash;
|
|
prevout = out;
|
|
}
|
|
|
|
CMempoolAddressDelta(int64_t t, CAmount a) {
|
|
time = t;
|
|
amount = a;
|
|
prevhash.SetNull();
|
|
prevout = 0;
|
|
}
|
|
};
|
|
|
|
struct CMempoolAddressDeltaKey
|
|
{
|
|
int type;
|
|
uint160 addressBytes;
|
|
uint256 txhash;
|
|
unsigned int index;
|
|
int spending;
|
|
|
|
CMempoolAddressDeltaKey(int addressType, uint160 addressHash, uint256 hash, unsigned int i, int s) {
|
|
type = addressType;
|
|
addressBytes = addressHash;
|
|
txhash = hash;
|
|
index = i;
|
|
spending = s;
|
|
}
|
|
|
|
CMempoolAddressDeltaKey(int addressType, uint160 addressHash) {
|
|
type = addressType;
|
|
addressBytes = addressHash;
|
|
txhash.SetNull();
|
|
index = 0;
|
|
spending = 0;
|
|
}
|
|
};
|
|
|
|
struct CMempoolAddressDeltaKeyCompare
|
|
{
|
|
bool operator()(const CMempoolAddressDeltaKey& a, const CMempoolAddressDeltaKey& b) const {
|
|
if (a.type == b.type) {
|
|
if (a.addressBytes == b.addressBytes) {
|
|
if (a.txhash == b.txhash) {
|
|
if (a.index == b.index) {
|
|
return a.spending < b.spending;
|
|
} else {
|
|
return a.index < b.index;
|
|
}
|
|
} else {
|
|
return a.txhash < b.txhash;
|
|
}
|
|
} else {
|
|
return a.addressBytes < b.addressBytes;
|
|
}
|
|
} else {
|
|
return a.type < b.type;
|
|
}
|
|
}
|
|
};
|
|
|
|
#endif // BITCOIN_ADDRESSINDEX_H
|