mirror of
https://github.com/dashpay/dash.git
synced 2024-12-27 13:03:17 +01:00
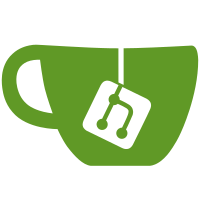
fa0074e2d82928016a43ca408717154a1c70a4db scripted-diff: Bump copyright headers (MarcoFalke) Pull request description: Needs to be done because no one has removed the years yet ACKs for top commit: practicalswift: ACK fa0074e2d82928016a43ca408717154a1c70a4db Tree-SHA512: 210e92acd7d400b556cf8259c3ec9967797420cfd19f0c2a4fa54cb2b3d32ad9ae27e771269201e7d554c0f4cd73a8b1c1a42c9f65d8685ca4d52e5134b071a3
96 lines
3.3 KiB
C++
96 lines
3.3 KiB
C++
// Copyright (c) 2019-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
//
|
|
#include <test/util/setup_common.h>
|
|
#include <util/system.h>
|
|
#include <univalue.h>
|
|
|
|
#ifdef HAVE_BOOST_PROCESS
|
|
#include <boost/process.hpp>
|
|
#endif // HAVE_BOOST_PROCESS
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
BOOST_FIXTURE_TEST_SUITE(system_tests, BasicTestingSetup)
|
|
|
|
// At least one test is required (in case HAVE_BOOST_PROCESS is not defined).
|
|
// Workaround for https://github.com/bitcoin/bitcoin/issues/19128
|
|
BOOST_AUTO_TEST_CASE(dummy)
|
|
{
|
|
BOOST_CHECK(true);
|
|
}
|
|
|
|
#ifdef HAVE_BOOST_PROCESS
|
|
|
|
BOOST_AUTO_TEST_CASE(run_command)
|
|
{
|
|
{
|
|
const UniValue result = RunCommandParseJSON("");
|
|
BOOST_CHECK(result.isNull());
|
|
}
|
|
{
|
|
#ifdef WIN32
|
|
const UniValue result = RunCommandParseJSON("cmd.exe /c echo {\"success\": true}");
|
|
#else
|
|
const UniValue result = RunCommandParseJSON("echo \"{\"success\": true}\"");
|
|
#endif
|
|
BOOST_CHECK(result.isObject());
|
|
const UniValue& success = find_value(result, "success");
|
|
BOOST_CHECK(!success.isNull());
|
|
BOOST_CHECK_EQUAL(success.getBool(), true);
|
|
}
|
|
{
|
|
// An invalid command is handled by Boost
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON("invalid_command"), boost::process::process_error, [&](const boost::process::process_error& e) {
|
|
BOOST_CHECK(std::string(e.what()).find("RunCommandParseJSON error:") == std::string::npos);
|
|
BOOST_CHECK_EQUAL(e.code().value(), 2);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
// Return non-zero exit code, no output to stderr
|
|
#ifdef WIN32
|
|
const std::string command{"cmd.exe /c call"};
|
|
#else
|
|
const std::string command{"false"};
|
|
#endif
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON(command), std::runtime_error, [&](const std::runtime_error& e) {
|
|
BOOST_CHECK(std::string(e.what()).find(strprintf("RunCommandParseJSON error: process(%s) returned 1: \n", command)) != std::string::npos);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
// Return non-zero exit code, with error message for stderr
|
|
#ifdef WIN32
|
|
const std::string command{"cmd.exe /c dir nosuchfile"};
|
|
const std::string expected{"File Not Found"};
|
|
#else
|
|
const std::string command{"ls nosuchfile"};
|
|
const std::string expected{"No such file or directory"};
|
|
#endif
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON(command), std::runtime_error, [&](const std::runtime_error& e) {
|
|
const std::string what(e.what());
|
|
BOOST_CHECK(what.find(strprintf("RunCommandParseJSON error: process(%s) returned", command)) != std::string::npos);
|
|
BOOST_CHECK(what.find(expected) != std::string::npos);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
BOOST_REQUIRE_THROW(RunCommandParseJSON("echo \"{\""), std::runtime_error); // Unable to parse JSON
|
|
}
|
|
// Test std::in, except for Windows
|
|
#ifndef WIN32
|
|
{
|
|
const UniValue result = RunCommandParseJSON("cat", "{\"success\": true}");
|
|
BOOST_CHECK(result.isObject());
|
|
const UniValue& success = find_value(result, "success");
|
|
BOOST_CHECK(!success.isNull());
|
|
BOOST_CHECK_EQUAL(success.getBool(), true);
|
|
}
|
|
#endif
|
|
}
|
|
#endif // HAVE_BOOST_PROCESS
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|