mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
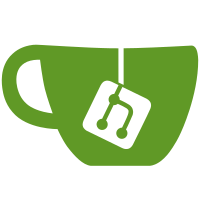
* qt|wallet|privatesend: Rename PrivateSend to CoinJoin in GUI strings * qt: Move CoinJoin next to Transactions * qt: Adjust status tip of privateSendCoinsMenuAction Co-authored-by: thephez <thephez@users.noreply.github.com> * rename: privateSend -> coinJoin * rename: privatesend -> coinjoin * rename: PrivateSend -> CoinJoin * rename: use_ps -> use_cj * rename: PRIVATESEND -> COINJOIN * rename: privatesend -> coinjoin for files and folders * refactor: Re-order coinjoin files in cmake/make files * refactor: Re-order coinjoin includes where it makes sense * test: Update lint-circular-dependencies.sh * Few cleanups * test: test/coinjoin_tests.cpp -> wallet/test/coinjoin_test.cpp * s/AdvancedPSUI/AdvancedCJUI/g * s/privateSentAmountChanged/coinJoinAmountChanged/g * wallet: Rename "ps_salt" backwards compatible * Minimal PrivateSend -> CoinJoin migration for settings and cmd-line * wallet: Fix privatesendrounds -> coinjoinrounds migration * qt: Migrate nPrivateSendAmount -> nCoinJoinAmount * `-coinjoindenoms` never existed * Migrate all PS options/settings * rpc: Formatting only * qt: Make Send/CoinJoin tabs a bit more distinguishable Co-authored-by: thephez <thephez@users.noreply.github.com> Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
61 lines
1.8 KiB
Python
Executable File
61 lines
1.8 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2019 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal
|
|
|
|
'''
|
|
rpc_coinjoin.py
|
|
|
|
Tests CoinJoin basic RPC
|
|
'''
|
|
|
|
class CoinJoinTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 1
|
|
|
|
def run_test(self):
|
|
self.test_coinjoin_start_stop()
|
|
self.test_coinjoin_setamount()
|
|
self.test_coinjoin_setrounds()
|
|
|
|
def test_coinjoin_start_stop(self):
|
|
# Start Mixing
|
|
self.nodes[0].coinjoin("start")
|
|
# Get CoinJoin info
|
|
cj_info = self.nodes[0].getcoinjoininfo()
|
|
# Ensure that it started properly
|
|
assert_equal(cj_info['enabled'], True)
|
|
assert_equal(cj_info['running'], True)
|
|
|
|
# Stop mixing
|
|
self.nodes[0].coinjoin("stop")
|
|
# Get CoinJoin info
|
|
cj_info = self.nodes[0].getcoinjoininfo()
|
|
# Ensure that it stopped properly
|
|
assert_equal(cj_info['enabled'], True)
|
|
assert_equal(cj_info['running'], False)
|
|
|
|
def test_coinjoin_setamount(self):
|
|
# Try normal values
|
|
self.nodes[0].setcoinjoinamount(50)
|
|
cj_info = self.nodes[0].getcoinjoininfo()
|
|
assert_equal(cj_info['max_amount'], 50)
|
|
|
|
# Try large values
|
|
self.nodes[0].setcoinjoinamount(1200000)
|
|
cj_info = self.nodes[0].getcoinjoininfo()
|
|
assert_equal(cj_info['max_amount'], 1200000)
|
|
|
|
def test_coinjoin_setrounds(self):
|
|
# Try normal values
|
|
self.nodes[0].setcoinjoinrounds(5)
|
|
cj_info = self.nodes[0].getcoinjoininfo()
|
|
assert_equal(cj_info['max_rounds'], 5)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
CoinJoinTest().main()
|