mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
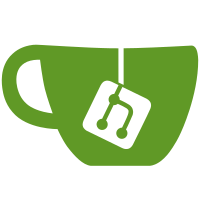
## Issue being fixed or feature implemented Client version string is inconsistent. Building `v20.0.0-beta.8` tag locally produces binaries that report `v20.0.0-beta.8` version but binaries built in guix would report `v20.0.0rc1-g3e732a952226a20505f907e4fd9b3fdbb14ea5ee` instead. Building any commit after `v20.0.0-beta.8` locally would result in versions like `v20.0.0rc1-8c94153d2497` which is close but it's still yet another format. And both versions with `rc1` in their names are confusing cause you'd expect them to mention `beta.8` instead maybe (or is it just me? :D ). ## What was done? Change it so that the version string would look like this: on tag: ~`v20.0.0-beta.8-dev` or `v20.0.0-beta.8-gitarc`~ `v20.0.0-beta.8` post-tag: ~`v20.0.0-beta.8-1-gb837e08164-gitarc`~ `v20.0.0-beta.8-1-gb837e08164` post-tag format is `recent tag`-`commits since that tag`-`g+12 chars of commit hash`-`dirty (optional)` ~-`dev or gitarc`~ ~`dev`/`gitarc` suffixes should help avoiding confusion with the release versions and they also indicate the way non-release binaries were built.~ Note that release binaries do not use any of this, they still use `PACKAGE_VERSION` from `configure` like before. Also, `CLIENT_VERSION_RC` is no longer used in this setup so it was removed. Few things aren't clear to me yet: 1. Version bump in `configure.ac` no longer affects the reported version (unless it's an actual release). Are there any downsides I might be missing? 2. Which tag should we use on `develop` once we bump version in configure? `v21.0.0-init`? `v21.0.0-alpha1`? 3. How is it going to behave once `merge master back into develop` kind of PR is merged? E.g. say `develop` branch is on `v21.0.0-alpha1` tag and we merge v20.1.0 from `master` back into it. Will this bring `v20.1.0` release tag into `develop`? Will it become the one that will be used from that moment? If so we will probably need another tag on `develop` every time such PR is merged e.g. `v21.0.0-alpha2` (or whatever the next number is). Don't think these are blockers but would like to hear thoughts from others. ## How Has This Been Tested? Built binaries locally, built them using guix at a specific tag and at some commit on top of it. ## Breaking Changes n/a ## Checklist: - [x] I have performed a self-review of my own code - [x] I have commented my code, particularly in hard-to-understand areas - [ ] I have added or updated relevant unit/integration/functional/e2e tests - [ ] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone _(for repository code-owners and collaborators only)_
77 lines
2.4 KiB
C++
77 lines
2.4 KiB
C++
// Copyright (c) 2012-2014 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <clientversion.h>
|
|
|
|
#include <tinyformat.h>
|
|
|
|
|
|
/**
|
|
* Name of client reported in the 'version' message. Report the same name
|
|
* for both dashd and dash-qt, to make it harder for attackers to
|
|
* target servers or GUI users specifically.
|
|
*/
|
|
const std::string CLIENT_NAME("Dash Core");
|
|
|
|
|
|
#ifdef HAVE_BUILD_INFO
|
|
#include <obj/build.h>
|
|
// The <obj/build.h>, which is generated by the build environment (share/genbuild.sh),
|
|
// could contain only one line of the following:
|
|
// - "#define BUILD_GIT_DESCRIPTION ...", if the top commit is not tagged
|
|
// - "// No build information available", if proper git information is not available
|
|
#endif
|
|
|
|
//! git will put "#define ARCHIVE_GIT_DESCRIPTION ..." on the next line inside archives. $Format:%n#define ARCHIVE_GIT_DESCRIPTION "%(describe:abbrev=12)"$
|
|
|
|
#if CLIENT_VERSION_IS_RELEASE
|
|
#define BUILD_DESC "v" PACKAGE_VERSION
|
|
#define BUILD_SUFFIX ""
|
|
#else
|
|
#if defined(BUILD_GIT_DESCRIPTION)
|
|
// build in a cloned folder
|
|
#define BUILD_DESC BUILD_GIT_DESCRIPTION
|
|
#define BUILD_SUFFIX ""
|
|
#elif defined(ARCHIVE_GIT_DESCRIPTION)
|
|
// build in a folder from git archive
|
|
#define BUILD_DESC ARCHIVE_GIT_DESCRIPTION
|
|
#define BUILD_SUFFIX ""
|
|
#else
|
|
#define BUILD_DESC "v" PACKAGE_VERSION
|
|
#define BUILD_SUFFIX "-unk"
|
|
#endif
|
|
#endif
|
|
|
|
const std::string CLIENT_BUILD(BUILD_DESC BUILD_SUFFIX);
|
|
|
|
std::string FormatVersion(int nVersion)
|
|
{
|
|
return strprintf("%d.%d.%d", nVersion / 10000, (nVersion / 100) % 100, nVersion % 100);
|
|
}
|
|
|
|
std::string FormatFullVersion()
|
|
{
|
|
return CLIENT_BUILD;
|
|
}
|
|
|
|
/**
|
|
* Format the subversion field according to BIP 14 spec (https://github.com/bitcoin/bips/blob/master/bip-0014.mediawiki)
|
|
*/
|
|
std::string FormatSubVersion(const std::string& name, int nClientVersion, const std::vector<std::string>& comments)
|
|
{
|
|
std::ostringstream ss;
|
|
ss << "/";
|
|
ss << name << ":" << FormatVersion(nClientVersion);
|
|
if (!comments.empty())
|
|
{
|
|
std::vector<std::string>::const_iterator it(comments.begin());
|
|
ss << "(" << *it;
|
|
for(++it; it != comments.end(); ++it)
|
|
ss << "; " << *it;
|
|
ss << ")";
|
|
}
|
|
ss << "/";
|
|
return ss.str();
|
|
}
|