mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
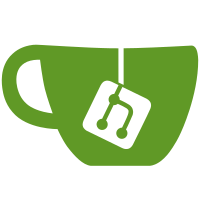
fa0ceae970242d8d6bdef150c98f04c67b06e20c test: Fix utxo set hash serialisation signedness (MarcoFalke)
Pull request description:
It is unsigned in Bitcoin Core, so the tests should match it:
5b8990a1f3/src/kernel/coinstats.cpp (L54)
Large positive values for the block height are too difficult to hit in tests, but it still seems fine to fix this.
The bug was introduced when the code was written in 6ccc8fc067bf516cda7bc5d7d721945be5ac2003.
(Lowercase `i` means signed, see https://docs.python.org/3/library/struct.html#format-characters)
ACKs for top commit:
epiccurious:
Tested ACK fa0ceae970242d8d6bdef150c98f04c67b06e20c.
fjahr:
utACK fa0ceae970242d8d6bdef150c98f04c67b06e20c
Tree-SHA512: ab4405c74fb191fff8520b456d3a800cd084d616bb9ddca27d56b8e5c8969bd537490f6e204c1870dbb09a3e130b03b22a27b6644252a024059c200bbd9004e7
79 lines
3.0 KiB
Python
Executable File
79 lines
3.0 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2020-2021 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test UTXO set hash value calculation in gettxoutsetinfo."""
|
|
|
|
from test_framework.messages import (
|
|
CBlock,
|
|
COutPoint,
|
|
from_hex,
|
|
)
|
|
from test_framework.crypto.muhash import MuHash3072
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal
|
|
from test_framework.wallet import MiniWallet
|
|
|
|
class UTXOSetHashTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 1
|
|
self.setup_clean_chain = True
|
|
|
|
def test_muhash_implementation(self):
|
|
self.log.info("Test MuHash implementation consistency")
|
|
|
|
node = self.nodes[0]
|
|
wallet = MiniWallet(node)
|
|
mocktime = node.getblockheader(node.getblockhash(0))['time'] + 1
|
|
node.setmocktime(mocktime)
|
|
|
|
# Generate 100 blocks and remove the first since we plan to spend its
|
|
# coinbase
|
|
block_hashes = self.generate(wallet, 1) + self.generate(node, 99)
|
|
blocks = list(map(lambda block: from_hex(CBlock(), node.getblock(block, False)), block_hashes))
|
|
blocks.pop(0)
|
|
|
|
# Create a spending transaction and mine a block which includes it
|
|
txid = wallet.send_self_transfer(from_node=node)['txid']
|
|
tx_block = self.generateblock(node, output=wallet.get_address(), transactions=[txid])['hash']
|
|
blocks.append(from_hex(CBlock(), node.getblock(tx_block, False)))
|
|
|
|
# Serialize the outputs that should be in the UTXO set and add them to
|
|
# a MuHash object
|
|
muhash = MuHash3072()
|
|
|
|
for height, block in enumerate(blocks):
|
|
# The Genesis block coinbase is not part of the UTXO set and we
|
|
# spent the first mined block
|
|
height += 2
|
|
|
|
for tx in block.vtx:
|
|
for n, tx_out in enumerate(tx.vout):
|
|
coinbase = 1 if not tx.vin[0].prevout.hash else 0
|
|
|
|
# Skip witness commitment
|
|
if (coinbase and n > 0):
|
|
continue
|
|
|
|
data = COutPoint(int(tx.rehash(), 16), n).serialize()
|
|
data += (height * 2 + coinbase).to_bytes(4, "little")
|
|
data += tx_out.serialize()
|
|
|
|
muhash.insert(data)
|
|
|
|
finalized = muhash.digest()
|
|
node_muhash = node.gettxoutsetinfo("muhash")['muhash']
|
|
|
|
assert_equal(finalized[::-1].hex(), node_muhash)
|
|
|
|
self.log.info("Test deterministic UTXO set hash results")
|
|
assert_equal(node.gettxoutsetinfo()['hash_serialized_2'], "4eb23b9673b7472e33ebf6e6aefee849fe5bc5e598fd387c2f9222070cc2f711")
|
|
assert_equal(node.gettxoutsetinfo("muhash")['muhash'], "dd74d7f1fb047577915d490e82d063e843f7853ae7543c9980a28c67326e1a2c")
|
|
|
|
def run_test(self):
|
|
self.test_muhash_implementation()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
UTXOSetHashTest().main()
|