mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
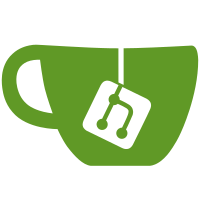
* Remove leftover RBF code from BTC
* remove rbf #include
* remove rbf in rpc-tests
* removes replace-by-fee.py
* remove help text related to rbf
* remove comment text relating to rbf
* remove "-mempoolreplacement" cli option
* Remove (effectively dead) RBF code which would never have been called anyway and some assosiated variables
* since `setConflicts` is always empty, this is dead code
* Since we don't have RBF, don't have to do this check. Also, since `setConflicts` is always empty this is dead code
* removes unneccesary if as it will always be true
* remove unused `set<uint256> setConflicts`
* Removes replacement of conflicting txs, as conflicting txs are never accepted
* removes RBF from `validForFeeEstimation`
* removes (probably) unnecessary lock
* remove replacing part of the AcceptToMemoryPool and AcceptToMemoryPoolWIthTime
* fixes err in ps.cpp, didn't remove arg
* RBF in net_processing.cpp
* remove arg in ps-server.cpp
* removes another arg in PS code
* removes rawtx.c AcceptToMemoryPool arg
* removes arg in txvalidationcache_tests.cpp
* remove extra args
* forgot an arg
* fix typo in 82898b0
* remove unused fEnableReplacement in validation.h
* remove the removal reason REPLACED in txmempool.h
* removed unused variable
* comment typo
99 lines
4.8 KiB
Python
Executable File
99 lines
4.8 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2014-2016 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
# Exercise the listtransactions API
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
from test_framework.mininode import CTransaction, COIN
|
|
from io import BytesIO
|
|
|
|
def txFromHex(hexstring):
|
|
tx = CTransaction()
|
|
f = BytesIO(hex_str_to_bytes(hexstring))
|
|
tx.deserialize(f)
|
|
return tx
|
|
|
|
class ListTransactionsTest(BitcoinTestFramework):
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.num_nodes = 4
|
|
self.setup_clean_chain = False
|
|
|
|
def run_test(self):
|
|
# Simple send, 0 to 1:
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[1].getnewaddress(), 0.1)
|
|
self.sync_all()
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"txid":txid},
|
|
{"category":"send","account":"","amount":Decimal("-0.1"),"confirmations":0})
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"txid":txid},
|
|
{"category":"receive","account":"","amount":Decimal("0.1"),"confirmations":0})
|
|
# mine a block, confirmations should change:
|
|
self.nodes[0].generate(1)
|
|
self.sync_all()
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"txid":txid},
|
|
{"category":"send","account":"","amount":Decimal("-0.1"),"confirmations":1})
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"txid":txid},
|
|
{"category":"receive","account":"","amount":Decimal("0.1"),"confirmations":1})
|
|
|
|
# send-to-self:
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[0].getnewaddress(), 0.2)
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"txid":txid, "category":"send"},
|
|
{"amount":Decimal("-0.2")})
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"txid":txid, "category":"receive"},
|
|
{"amount":Decimal("0.2")})
|
|
|
|
# sendmany from node1: twice to self, twice to node2:
|
|
send_to = { self.nodes[0].getnewaddress() : 0.11,
|
|
self.nodes[1].getnewaddress() : 0.22,
|
|
self.nodes[0].getaccountaddress("from1") : 0.33,
|
|
self.nodes[1].getaccountaddress("toself") : 0.44 }
|
|
txid = self.nodes[1].sendmany("", send_to)
|
|
self.sync_all()
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"send","amount":Decimal("-0.11")},
|
|
{"txid":txid} )
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"category":"receive","amount":Decimal("0.11")},
|
|
{"txid":txid} )
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"send","amount":Decimal("-0.22")},
|
|
{"txid":txid} )
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"receive","amount":Decimal("0.22")},
|
|
{"txid":txid} )
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"send","amount":Decimal("-0.33")},
|
|
{"txid":txid} )
|
|
assert_array_result(self.nodes[0].listtransactions(),
|
|
{"category":"receive","amount":Decimal("0.33")},
|
|
{"txid":txid, "account" : "from1"} )
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"send","amount":Decimal("-0.44")},
|
|
{"txid":txid, "account" : ""} )
|
|
assert_array_result(self.nodes[1].listtransactions(),
|
|
{"category":"receive","amount":Decimal("0.44")},
|
|
{"txid":txid, "account" : "toself"} )
|
|
|
|
multisig = self.nodes[1].createmultisig(1, [self.nodes[1].getnewaddress()])
|
|
self.nodes[0].importaddress(multisig["redeemScript"], "watchonly", False, True)
|
|
txid = self.nodes[1].sendtoaddress(multisig["address"], 0.1)
|
|
self.nodes[1].generate(1)
|
|
self.sync_all()
|
|
assert(len(self.nodes[0].listtransactions("watchonly", 100, 0, False)) == 0)
|
|
assert_array_result(self.nodes[0].listtransactions("watchonly", 100, 0, True),
|
|
{"category":"receive","amount":Decimal("0.1")},
|
|
{"txid":txid, "account" : "watchonly"} )
|
|
|
|
if __name__ == '__main__':
|
|
ListTransactionsTest().main()
|
|
|