mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
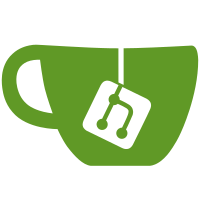
643aad17fa
Enable additional flake8 rules (practicalswift)f020aca297
Minor Python cleanups to make flake8 pass with the new rules enabled (practicalswift) Pull request description: Enabled rules: ``` * E242: tab after ',' * E266: too many leading '#' for block comment * E401: multiple imports on one line * E402: module level import not at top of file * E701: multiple statements on one line (colon) * E901: SyntaxError: invalid syntax * E902: TokenError: EOF in multi-line string * F821: undefined name 'Foo' * W293: blank line contains whitespace * W606: 'async' and 'await' are reserved keywords starting with Python 3.7 ``` Note to reviewers: * In general we don't allow whitespace cleanups to existing code, but in order to allow for enabling Travis checking for these rules a few smaller whitespace cleanups had to made as part of this PR. * Use [this `?w=1` link](https://github.com/bitcoin/bitcoin/pull/12987/files?w=1) to show a diff without whitespace changes. Before this commit: ``` $ flake8 -qq --statistics --ignore=B,C,E,F,I,N,W --select=E112,E113,E115,E116,E125,E131,E133,E223,E224,E242,E266,E271,E272,E273,E274,E275,E304,E306,E401,E402,E502,E701,E702,E703,E714,E721,E741,E742,E743,F401,E901,E902,F402,F404,F406,F407,F601,F602,F621,F622,F631,F701,F702,F703,F704,F705,F706,F707,F811,F812,F821,F822,F823,F831,F841,W292,W293,W504,W601,W602,W603,W604,W605,W606 . 5 E266 too many leading '#' for block comment 4 E401 multiple imports on one line 6 E402 module level import not at top of file 5 E701 multiple statements on one line (colon) 1 F812 list comprehension redefines 'n' from line 159 4 F821 undefined name 'ConnectionRefusedError' 28 W293 blank line contains whitespace ``` After this commit: ``` $ flake8 -qq --statistics --ignore=B,C,E,F,I,N,W --select=E112,E113,E115,E116,E125,E131,E133,E223,E224,E242,E266,E271,E272,E273,E274,E275,E304,E306,E401,E402,E502,E701,E702,E703,E714,E721,E741,E742,E743,F401,E901,E902,F402,F404,F406,F407,F601,F602,F621,F622,F631,F701,F702,F703,F704,F705,F706,F707,F811,F812,F821,F822,F823,F831,F841,W292,W293,W504,W601,W602,W603,W604,W605,W606 . $ ``` Tree-SHA512: fc7d5e752298a50d4248afc620ee2c173135b4ca008e48e02913ac968e5a24a5fd5396926047ec62f1d580d537434ccae01f249bb2f3338fa59dc630bf97ca7a Signed-off-by: pasta <pasta@dashboost.org>
115 lines
4.5 KiB
Python
Executable File
115 lines
4.5 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2014-2016 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test the importprunedfunds and removeprunedfunds RPCs."""
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
|
|
class ImportPrunedFundsTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 2
|
|
|
|
def run_test(self):
|
|
self.log.info("Mining blocks...")
|
|
self.nodes[0].generate(101)
|
|
|
|
self.sync_all()
|
|
|
|
# address
|
|
address1 = self.nodes[0].getnewaddress()
|
|
# pubkey
|
|
address2 = self.nodes[0].getnewaddress()
|
|
# privkey
|
|
address3 = self.nodes[0].getnewaddress()
|
|
address3_privkey = self.nodes[0].dumpprivkey(address3) # Using privkey
|
|
|
|
#Check only one address
|
|
address_info = self.nodes[0].validateaddress(address1)
|
|
assert_equal(address_info['ismine'], True)
|
|
|
|
self.sync_all()
|
|
|
|
#Node 1 sync test
|
|
assert_equal(self.nodes[1].getblockcount(),101)
|
|
|
|
#Address Test - before import
|
|
address_info = self.nodes[1].validateaddress(address1)
|
|
assert_equal(address_info['iswatchonly'], False)
|
|
assert_equal(address_info['ismine'], False)
|
|
|
|
address_info = self.nodes[1].validateaddress(address2)
|
|
assert_equal(address_info['iswatchonly'], False)
|
|
assert_equal(address_info['ismine'], False)
|
|
|
|
address_info = self.nodes[1].validateaddress(address3)
|
|
assert_equal(address_info['iswatchonly'], False)
|
|
assert_equal(address_info['ismine'], False)
|
|
|
|
#Send funds to self
|
|
txnid1 = self.nodes[0].sendtoaddress(address1, 0.1)
|
|
self.nodes[0].generate(1)
|
|
rawtxn1 = self.nodes[0].gettransaction(txnid1)['hex']
|
|
proof1 = self.nodes[0].gettxoutproof([txnid1])
|
|
|
|
txnid2 = self.nodes[0].sendtoaddress(address2, 0.05)
|
|
self.nodes[0].generate(1)
|
|
rawtxn2 = self.nodes[0].gettransaction(txnid2)['hex']
|
|
proof2 = self.nodes[0].gettxoutproof([txnid2])
|
|
|
|
txnid3 = self.nodes[0].sendtoaddress(address3, 0.025)
|
|
self.nodes[0].generate(1)
|
|
rawtxn3 = self.nodes[0].gettransaction(txnid3)['hex']
|
|
proof3 = self.nodes[0].gettxoutproof([txnid3])
|
|
|
|
self.sync_all()
|
|
|
|
#Import with no affiliated address
|
|
assert_raises_rpc_error(-5, "No addresses", self.nodes[1].importprunedfunds, rawtxn1, proof1)
|
|
|
|
balance1 = self.nodes[1].getbalance("", 0, False, True)
|
|
assert_equal(balance1, Decimal(0))
|
|
|
|
#Import with affiliated address with no rescan
|
|
self.nodes[1].importaddress(address2, "add2", False)
|
|
self.nodes[1].importprunedfunds(rawtxn2, proof2)
|
|
balance2 = self.nodes[1].getbalance("add2", 0, False, True)
|
|
assert_equal(balance2, Decimal('0.05'))
|
|
|
|
#Import with private key with no rescan
|
|
self.nodes[1].importprivkey(privkey=address3_privkey, label="add3", rescan=False)
|
|
self.nodes[1].importprunedfunds(rawtxn3, proof3)
|
|
balance3 = self.nodes[1].getbalance("add3", 0, False, False)
|
|
assert_equal(balance3, Decimal('0.025'))
|
|
balance3 = self.nodes[1].getbalance("*", 0, False, True)
|
|
assert_equal(balance3, Decimal('0.075'))
|
|
|
|
#Addresses Test - after import
|
|
address_info = self.nodes[1].validateaddress(address1)
|
|
assert_equal(address_info['iswatchonly'], False)
|
|
assert_equal(address_info['ismine'], False)
|
|
address_info = self.nodes[1].validateaddress(address2)
|
|
assert_equal(address_info['iswatchonly'], True)
|
|
assert_equal(address_info['ismine'], False)
|
|
address_info = self.nodes[1].validateaddress(address3)
|
|
assert_equal(address_info['iswatchonly'], False)
|
|
assert_equal(address_info['ismine'], True)
|
|
|
|
#Remove transactions
|
|
assert_raises_rpc_error(-8, "Transaction does not exist in wallet.", self.nodes[1].removeprunedfunds, txnid1)
|
|
|
|
balance1 = self.nodes[1].getbalance("*", 0, False, True)
|
|
assert_equal(balance1, Decimal('0.075'))
|
|
|
|
self.nodes[1].removeprunedfunds(txnid2)
|
|
balance2 = self.nodes[1].getbalance("*", 0, False, True)
|
|
assert_equal(balance2, Decimal('0.025'))
|
|
|
|
self.nodes[1].removeprunedfunds(txnid3)
|
|
balance3 = self.nodes[1].getbalance("*", 0, False, True)
|
|
assert_equal(balance3, Decimal('0.0'))
|
|
|
|
if __name__ == '__main__':
|
|
ImportPrunedFundsTest().main()
|