mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
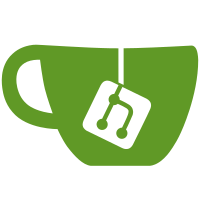
* Introduce SynchronousUpdatedBlockTip signal This version of UpdatedBlockTip mirrors the asynchronous behavior that we had before the introduction of asynchronous signal handling. * Fix tab spacing in validationinterface.cpp * Invoke CDeterministicMNManager::UpdatedBlockTip from validation thread It must be invoked synchronously as otherwise things become inconsistent. * Call CActiveMasternodeManager::Init with block index pindexNew in UpdatedBlockTip is not necessarily the current tip, so we shouldn't rely on it in Init(). This is due to the async nature of the UpdatedBlockTip invocation.
36 lines
1.7 KiB
C++
36 lines
1.7 KiB
C++
// Copyright (c) 2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_DSNOTIFICATIONINTERFACE_H
|
|
#define BITCOIN_DSNOTIFICATIONINTERFACE_H
|
|
|
|
#include <validationinterface.h>
|
|
|
|
class CDSNotificationInterface : public CValidationInterface
|
|
{
|
|
public:
|
|
explicit CDSNotificationInterface(CConnman& connmanIn): connman(connmanIn) {}
|
|
virtual ~CDSNotificationInterface() = default;
|
|
|
|
// a small helper to initialize current block height in sub-modules on startup
|
|
void InitializeCurrentBlockTip();
|
|
|
|
protected:
|
|
// CValidationInterface
|
|
void AcceptedBlockHeader(const CBlockIndex *pindexNew) override;
|
|
void NotifyHeaderTip(const CBlockIndex *pindexNew, bool fInitialDownload) override;
|
|
void SynchronousUpdatedBlockTip(const CBlockIndex *pindexNew, const CBlockIndex *pindexFork, bool fInitialDownload) override;
|
|
void UpdatedBlockTip(const CBlockIndex *pindexNew, const CBlockIndex *pindexFork, bool fInitialDownload) override;
|
|
void TransactionAddedToMempool(const CTransactionRef& tx, int64_t nAcceptTime) override;
|
|
void BlockConnected(const std::shared_ptr<const CBlock>& pblock, const CBlockIndex* pindex, const std::vector<CTransactionRef>& vtxConflicted) override;
|
|
void BlockDisconnected(const std::shared_ptr<const CBlock>& pblock, const CBlockIndex* pindexDisconnected) override;
|
|
void NotifyMasternodeListChanged(bool undo, const CDeterministicMNList& oldMNList, const CDeterministicMNListDiff& diff) override;
|
|
void NotifyChainLock(const CBlockIndex* pindex, const llmq::CChainLockSig& clsig) override;
|
|
|
|
private:
|
|
CConnman& connman;
|
|
};
|
|
|
|
#endif // BITCOIN_DSNOTIFICATIONINTERFACE_H
|