mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
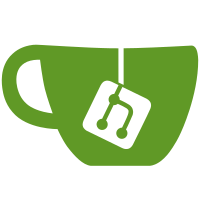
fa0074e2d82928016a43ca408717154a1c70a4db scripted-diff: Bump copyright headers (MarcoFalke) Pull request description: Needs to be done because no one has removed the years yet ACKs for top commit: practicalswift: ACK fa0074e2d82928016a43ca408717154a1c70a4db Tree-SHA512: 210e92acd7d400b556cf8259c3ec9967797420cfd19f0c2a4fa54cb2b3d32ad9ae27e771269201e7d554c0f4cd73a8b1c1a42c9f65d8685ca4d52e5134b071a3
46 lines
1.2 KiB
C++
46 lines
1.2 KiB
C++
// Copyright (c) 2018-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_UTIL_GOLOMBRICE_H
|
|
#define BITCOIN_UTIL_GOLOMBRICE_H
|
|
|
|
#include <util/fastrange.h>
|
|
|
|
#include <streams.h>
|
|
|
|
#include <cstdint>
|
|
|
|
template <typename OStream>
|
|
void GolombRiceEncode(BitStreamWriter<OStream>& bitwriter, uint8_t P, uint64_t x)
|
|
{
|
|
// Write quotient as unary-encoded: q 1's followed by one 0.
|
|
uint64_t q = x >> P;
|
|
while (q > 0) {
|
|
int nbits = q <= 64 ? static_cast<int>(q) : 64;
|
|
bitwriter.Write(~0ULL, nbits);
|
|
q -= nbits;
|
|
}
|
|
bitwriter.Write(0, 1);
|
|
|
|
// Write the remainder in P bits. Since the remainder is just the bottom
|
|
// P bits of x, there is no need to mask first.
|
|
bitwriter.Write(x, P);
|
|
}
|
|
|
|
template <typename IStream>
|
|
uint64_t GolombRiceDecode(BitStreamReader<IStream>& bitreader, uint8_t P)
|
|
{
|
|
// Read unary-encoded quotient: q 1's followed by one 0.
|
|
uint64_t q = 0;
|
|
while (bitreader.Read(1) == 1) {
|
|
++q;
|
|
}
|
|
|
|
uint64_t r = bitreader.Read(P);
|
|
|
|
return (q << P) + r;
|
|
}
|
|
|
|
#endif // BITCOIN_UTIL_GOLOMBRICE_H
|