mirror of
https://github.com/dashpay/dash.git
synced 2024-12-25 20:12:57 +01:00
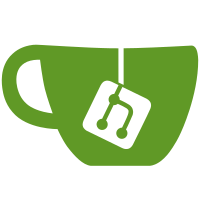
* build: use osslsigncode 2.0 in gitian The original osslsigncode project (https://sourceforge.net/projects/osslsigncode/) has been marked as abandonware, "This is now - and has been for a long while - abandonware. Feel free to create your own forks etc.". However, a fork at https://github.com/mtrojnar/osslsigncode has emerged that has incorporated theuni's patches, updated the tool to work with OpenSSL 1.1 and made other improvements. This commit switches the windows signer descriptor to use this new version of osslsigncode. * Fixed wget call in gitian-build.py Co-authored-by: Michael <fanquake@gmail.com> Co-authored-by: willyk <k.o.willy@gmail.com>
240 lines
13 KiB
Python
Executable File
240 lines
13 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
|
|
import argparse
|
|
import os
|
|
import subprocess
|
|
import sys
|
|
|
|
def setup():
|
|
global args, workdir
|
|
programs = ['ruby', 'git', 'make', 'wget']
|
|
if args.lxc:
|
|
programs += ['apt-cacher-ng', 'lxc', 'debootstrap']
|
|
elif args.kvm:
|
|
programs += ['apt-cacher-ng', 'python-vm-builder', 'qemu-kvm', 'qemu-utils']
|
|
elif args.docker and not os.path.isfile('/lib/systemd/system/docker.service'):
|
|
dockers = ['docker.io', 'docker-ce']
|
|
for i in dockers:
|
|
return_code = subprocess.call(['sudo', 'apt-get', 'install', '-qq', i])
|
|
if return_code == 0:
|
|
break
|
|
if return_code != 0:
|
|
print('Cannot find any way to install Docker', file=sys.stderr)
|
|
exit(1)
|
|
subprocess.check_call(['sudo', 'apt-get', 'install', '-qq'] + programs)
|
|
if not os.path.isdir('gitian.sigs'):
|
|
subprocess.check_call(['git', 'clone', 'https://github.com/dashpay/gitian.sigs.git'])
|
|
if not os.path.isdir('dash-detached-sigs'):
|
|
subprocess.check_call(['git', 'clone', 'https://github.com/dashpay/dash-detached-sigs.git'])
|
|
if not os.path.isdir('gitian-builder'):
|
|
subprocess.check_call(['git', 'clone', 'https://github.com/devrandom/gitian-builder.git'])
|
|
if not os.path.isdir('dash'):
|
|
subprocess.check_call(['git', 'clone', 'https://github.com/dashpay/dash.git'])
|
|
os.chdir('gitian-builder')
|
|
make_image_prog = ['bin/make-base-vm', '--suite', 'bionic', '--arch', 'amd64']
|
|
if args.docker:
|
|
make_image_prog += ['--docker']
|
|
elif args.lxc:
|
|
make_image_prog += ['--lxc']
|
|
subprocess.check_call(make_image_prog)
|
|
os.chdir(workdir)
|
|
if args.is_bionic and not args.kvm and not args.docker:
|
|
subprocess.check_call(['sudo', 'sed', '-i', 's/lxcbr0/br0/', '/etc/default/lxc-net'])
|
|
print('Reboot is required')
|
|
exit(0)
|
|
|
|
def build():
|
|
global args, workdir
|
|
|
|
os.makedirs('dashcore-binaries/' + args.version, exist_ok=True)
|
|
print('\nBuilding Dependencies\n')
|
|
os.chdir('gitian-builder')
|
|
os.makedirs('inputs', exist_ok=True)
|
|
|
|
subprocess.check_call(['wget', '-O', 'inputs/osslsigncode-2.0.tar.gz', 'https://github.com/mtrojnar/osslsigncode/archive/2.0.tar.gz'])
|
|
subprocess.check_call(["echo '5a60e0a4b3e0b4d655317b2f12a810211c50242138322b16e7e01c6fbb89d92f inputs/osslsigncode-2.0.tar.gz' | sha256sum -c"], shell=True)
|
|
subprocess.check_call(['make', '-C', '../dash/depends', 'download', 'SOURCES_PATH=' + os.getcwd() + '/cache/common'])
|
|
|
|
if args.linux:
|
|
print('\nCompiling ' + args.version + ' Linux')
|
|
subprocess.check_call(['bin/gbuild', '-j', args.jobs, '-m', args.memory, '--commit', 'dash='+args.commit, '--url', 'dash='+args.url, '../dash/contrib/gitian-descriptors/gitian-linux.yml'])
|
|
subprocess.check_call(['bin/gsign', '-p', args.sign_prog, '--signer', args.signer, '--release', args.version+'-linux', '--destination', '../gitian.sigs/', '../dash/contrib/gitian-descriptors/gitian-linux.yml'])
|
|
subprocess.check_call('mv build/out/dashcore-*.tar.gz build/out/src/dashcore-*.tar.gz ../dashcore-binaries/'+args.version, shell=True)
|
|
|
|
if args.windows:
|
|
print('\nCompiling ' + args.version + ' Windows')
|
|
subprocess.check_call(['bin/gbuild', '-j', args.jobs, '-m', args.memory, '--commit', 'dash='+args.commit, '--url', 'dash='+args.url, '../dash/contrib/gitian-descriptors/gitian-win.yml'])
|
|
subprocess.check_call(['bin/gsign', '-p', args.sign_prog, '--signer', args.signer, '--release', args.version+'-win-unsigned', '--destination', '../gitian.sigs/', '../dash/contrib/gitian-descriptors/gitian-win.yml'])
|
|
subprocess.check_call('mv build/out/dashcore-*-win-unsigned.tar.gz inputs/dashcore-win-unsigned.tar.gz', shell=True)
|
|
subprocess.check_call('mv build/out/dashcore-*.zip build/out/dashcore-*.exe ../dashcore-binaries/'+args.version, shell=True)
|
|
|
|
if args.macos:
|
|
print('\nCompiling ' + args.version + ' MacOS')
|
|
subprocess.check_call(['wget', '-N', '-P', 'inputs', 'https://bitcoincore.org/depends-sources/sdks/MacOSX10.11.sdk.tar.gz'])
|
|
subprocess.check_output(["echo 'bec9d089ebf2e2dd59b1a811a38ec78ebd5da18cbbcd6ab39d1e59f64ac5033f inputs/MacOSX10.11.sdk.tar.gz' | sha256sum -c"], shell=True)
|
|
subprocess.check_call(['bin/gbuild', '-j', args.jobs, '-m', args.memory, '--commit', 'dash='+args.commit, '--url', 'dash='+args.url, '../dash/contrib/gitian-descriptors/gitian-osx.yml'])
|
|
subprocess.check_call(['bin/gsign', '-p', args.sign_prog, '--signer', args.signer, '--release', args.version+'-osx-unsigned', '--destination', '../gitian.sigs/', '../dash/contrib/gitian-descriptors/gitian-osx.yml'])
|
|
subprocess.check_call('mv build/out/dashcore-*-osx-unsigned.tar.gz inputs/dashcore-osx-unsigned.tar.gz', shell=True)
|
|
subprocess.check_call('mv build/out/dashcore-*.tar.gz build/out/dashcore-*.dmg ../dashcore-binaries/'+args.version, shell=True)
|
|
|
|
os.chdir(workdir)
|
|
|
|
if args.commit_files:
|
|
print('\nCommitting '+args.version+' Unsigned Sigs\n')
|
|
os.chdir('gitian.sigs')
|
|
subprocess.check_call(['git', 'add', args.version+'-linux/'+args.signer])
|
|
subprocess.check_call(['git', 'add', args.version+'-win-unsigned/'+args.signer])
|
|
subprocess.check_call(['git', 'add', args.version+'-osx-unsigned/'+args.signer])
|
|
subprocess.check_call(['git', 'commit', '-m', 'Add '+args.version+' unsigned sigs for '+args.signer])
|
|
os.chdir(workdir)
|
|
|
|
def sign():
|
|
global args, workdir
|
|
os.chdir('gitian-builder')
|
|
|
|
if args.windows:
|
|
print('\nSigning ' + args.version + ' Windows')
|
|
subprocess.check_call(['bin/gbuild', '-i', '--commit', 'signature='+args.commit, '../dash/contrib/gitian-descriptors/gitian-win-signer.yml'])
|
|
subprocess.check_call(['bin/gsign', '-p', args.sign_prog, '--signer', args.signer, '--release', args.version+'-win-signed', '--destination', '../gitian.sigs/', '../dash/contrib/gitian-descriptors/gitian-win-signer.yml'])
|
|
subprocess.check_call('mv build/out/dashcore-*win64-setup.exe ../dashcore-binaries/'+args.version, shell=True)
|
|
subprocess.check_call('mv build/out/dashcore-*win32-setup.exe ../dashcore-binaries/'+args.version, shell=True)
|
|
|
|
if args.macos:
|
|
print('\nSigning ' + args.version + ' MacOS')
|
|
subprocess.check_call(['bin/gbuild', '-i', '--commit', 'signature='+args.commit, '../dash/contrib/gitian-descriptors/gitian-osx-signer.yml'])
|
|
subprocess.check_call(['bin/gsign', '-p', args.sign_prog, '--signer', args.signer, '--release', args.version+'-osx-signed', '--destination', '../gitian.sigs/', '../dash/contrib/gitian-descriptors/gitian-osx-signer.yml'])
|
|
subprocess.check_call('mv build/out/dashcore-osx-signed.dmg ../dashcore-binaries/'+args.version+'/dashcore-'+args.version+'-osx.dmg', shell=True)
|
|
|
|
os.chdir(workdir)
|
|
|
|
if args.commit_files:
|
|
print('\nCommitting '+args.version+' Signed Sigs\n')
|
|
os.chdir('gitian.sigs')
|
|
subprocess.check_call(['git', 'add', args.version+'-win-signed/'+args.signer])
|
|
subprocess.check_call(['git', 'add', args.version+'-osx-signed/'+args.signer])
|
|
subprocess.check_call(['git', 'commit', '-a', '-m', 'Add '+args.version+' signed binary sigs for '+args.signer])
|
|
os.chdir(workdir)
|
|
|
|
def verify():
|
|
global args, workdir
|
|
os.chdir('gitian-builder')
|
|
|
|
print('\nVerifying v'+args.version+' Linux\n')
|
|
subprocess.call(['bin/gverify', '-v', '-d', '../gitian.sigs/', '-r', args.version+'-linux', '../dash/contrib/gitian-descriptors/gitian-linux.yml'])
|
|
print('\nVerifying v'+args.version+' Windows\n')
|
|
subprocess.call(['bin/gverify', '-v', '-d', '../gitian.sigs/', '-r', args.version+'-win-unsigned', '../dash/contrib/gitian-descriptors/gitian-win.yml'])
|
|
print('\nVerifying v'+args.version+' MacOS\n')
|
|
subprocess.call(['bin/gverify', '-v', '-d', '../gitian.sigs/', '-r', args.version+'-osx-unsigned', '../dash/contrib/gitian-descriptors/gitian-osx.yml'])
|
|
print('\nVerifying v'+args.version+' Signed Windows\n')
|
|
subprocess.call(['bin/gverify', '-v', '-d', '../gitian.sigs/', '-r', args.version+'-win-signed', '../dash/contrib/gitian-descriptors/gitian-win-signer.yml'])
|
|
print('\nVerifying v'+args.version+' Signed MacOS\n')
|
|
subprocess.call(['bin/gverify', '-v', '-d', '../gitian.sigs/', '-r', args.version+'-osx-signed', '../dash/contrib/gitian-descriptors/gitian-osx-signer.yml'])
|
|
|
|
os.chdir(workdir)
|
|
|
|
def main():
|
|
global args, workdir
|
|
|
|
parser = argparse.ArgumentParser(usage='%(prog)s [options] signer version')
|
|
parser.add_argument('-c', '--commit', action='store_true', dest='commit', help='Indicate that the version argument is for a commit or branch')
|
|
parser.add_argument('-p', '--pull', action='store_true', dest='pull', help='Indicate that the version argument is the number of a github repository pull request')
|
|
parser.add_argument('-u', '--url', dest='url', default='https://github.com/dashpay/dash', help='Specify the URL of the repository. Default is %(default)s')
|
|
parser.add_argument('-v', '--verify', action='store_true', dest='verify', help='Verify the Gitian build')
|
|
parser.add_argument('-b', '--build', action='store_true', dest='build', help='Do a Gitian build')
|
|
parser.add_argument('-s', '--sign', action='store_true', dest='sign', help='Make signed binaries for Windows and MacOS')
|
|
parser.add_argument('-B', '--buildsign', action='store_true', dest='buildsign', help='Build both signed and unsigned binaries')
|
|
parser.add_argument('-o', '--os', dest='os', default='lwm', help='Specify which Operating Systems the build is for. Default is %(default)s. l for Linux, w for Windows, m for MacOS')
|
|
parser.add_argument('-j', '--jobs', dest='jobs', default='2', help='Number of processes to use. Default %(default)s')
|
|
parser.add_argument('-m', '--memory', dest='memory', default='2000', help='Memory to allocate in MiB. Default %(default)s')
|
|
parser.add_argument('-V', '--virtualization', dest='virtualization', default='docker', help='Specify virtualization technology to use: lxc for LXC, kvm for KVM, docker for Docker. Default is %(default)s')
|
|
parser.add_argument('-S', '--setup', action='store_true', dest='setup', help='Set up the Gitian building environment. Only works on Debian-based systems (Ubuntu, Debian)')
|
|
parser.add_argument('-D', '--detach-sign', action='store_true', dest='detach_sign', help='Create the assert file for detached signing. Will not commit anything.')
|
|
parser.add_argument('-n', '--no-commit', action='store_false', dest='commit_files', help='Do not commit anything to git')
|
|
parser.add_argument('signer', help='GPG signer to sign each build assert file')
|
|
parser.add_argument('version', help='Version number, commit, or branch to build. If building a commit or branch, the -c option must be specified')
|
|
|
|
args = parser.parse_args()
|
|
workdir = os.getcwd()
|
|
|
|
args.linux = 'l' in args.os
|
|
args.windows = 'w' in args.os
|
|
args.macos = 'm' in args.os
|
|
|
|
args.is_bionic = b'bionic' in subprocess.check_output(['lsb_release', '-cs'])
|
|
|
|
if args.buildsign:
|
|
args.build = True
|
|
args.sign = True
|
|
|
|
args.sign_prog = 'true' if args.detach_sign else 'gpg --detach-sign'
|
|
|
|
args.lxc = (args.virtualization == 'lxc')
|
|
args.kvm = (args.virtualization == 'kvm')
|
|
args.docker = (args.virtualization == 'docker')
|
|
|
|
script_name = os.path.basename(sys.argv[0])
|
|
# Set all USE_* environment variables for gitian-builder: USE_LXC, USE_DOCKER and USE_VBOX
|
|
os.environ['USE_VBOX'] = ''
|
|
if args.lxc:
|
|
os.environ['USE_LXC'] = '1'
|
|
os.environ['USE_DOCKER'] = ''
|
|
if 'GITIAN_HOST_IP' not in os.environ.keys():
|
|
os.environ['GITIAN_HOST_IP'] = '10.0.3.1'
|
|
if 'LXC_GUEST_IP' not in os.environ.keys():
|
|
os.environ['LXC_GUEST_IP'] = '10.0.3.5'
|
|
elif args.kvm:
|
|
os.environ['USE_LXC'] = ''
|
|
os.environ['USE_DOCKER'] = ''
|
|
elif args.docker:
|
|
os.environ['USE_LXC'] = ''
|
|
os.environ['USE_DOCKER'] = '1'
|
|
else:
|
|
print(script_name+': Wrong virtualization option.')
|
|
print('Try '+script_name+' --help for more information')
|
|
exit(1)
|
|
|
|
# Signer and version shouldn't be empty
|
|
if args.signer == '':
|
|
print(script_name+': Missing signer.')
|
|
print('Try '+script_name+' --help for more information')
|
|
exit(1)
|
|
if args.version == '':
|
|
print(script_name+': Missing version.')
|
|
print('Try '+script_name+' --help for more information')
|
|
exit(1)
|
|
|
|
# Add leading 'v' for tags
|
|
if args.commit and args.pull:
|
|
raise Exception('Cannot have both commit and pull')
|
|
args.commit = ('' if args.commit else 'v') + args.version
|
|
|
|
if args.setup:
|
|
setup()
|
|
|
|
if not args.build and not args.sign and not args.verify:
|
|
exit(0)
|
|
|
|
os.chdir('dash')
|
|
if args.pull:
|
|
subprocess.check_call(['git', 'fetch', args.url, 'refs/pull/'+args.version+'/merge'])
|
|
os.chdir('../gitian-builder/inputs/dash')
|
|
subprocess.check_call(['git', 'fetch', args.url, 'refs/pull/'+args.version+'/merge'])
|
|
args.commit = subprocess.check_output(['git', 'show', '-s', '--format=%H', 'FETCH_HEAD'], universal_newlines=True, encoding='utf8').strip()
|
|
args.version = 'pull-' + args.version
|
|
print(args.commit)
|
|
subprocess.check_call(['git', 'fetch'])
|
|
subprocess.check_call(['git', 'checkout', args.commit])
|
|
os.chdir(workdir)
|
|
|
|
if args.build:
|
|
build()
|
|
|
|
if args.sign:
|
|
sign()
|
|
|
|
if args.verify:
|
|
verify()
|
|
|
|
if __name__ == '__main__':
|
|
main()
|