mirror of
https://github.com/dashpay/dash.git
synced 2024-12-29 05:49:11 +01:00
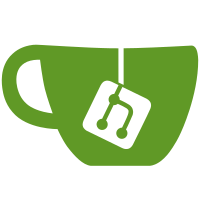
* llmq: move initialization logic to 'LLMQContext', add unique pointer to NodeContext * llmq: add aliases to LLMQ globals, expose them to RPC via LLMQContext * rpc: replace most global invocations with LLMQContext aliases * rpc: replace quorum RPC global invocations with LLMQContext aliases * llmq: replace individual global member arguments with context pointer * llmq: pass aliased context pointer instead of individual globals in tests * llmq: move BLS worker to LLMQContext, remove global * llmq: move DKG debug manager to LLMQContext, remove global * llmq: move DKG session manager to LLMQContext, remove global * llmq: move quorum share manager to LLMQContext, remove global * llmq: move quorum signing manager to LLMQContext, remove global
55 lines
2.8 KiB
C++
55 lines
2.8 KiB
C++
// Copyright (c) 2022 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <test/util/setup_common.h>
|
|
|
|
#include <llmq/context.h>
|
|
#include <llmq/utils.h>
|
|
#include <llmq/params.h>
|
|
#include <llmq/quorums.h>
|
|
|
|
#include <chainparams.h>
|
|
|
|
#include <validation.h>
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
/* TODO: rename this file and test to llmq_utils_test */
|
|
BOOST_AUTO_TEST_SUITE(evo_utils_tests)
|
|
|
|
void Test(llmq::CQuorumManager& qman)
|
|
{
|
|
using namespace llmq::utils;
|
|
const auto& consensus_params = Params().GetConsensus();
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeInstantSend, qman, nullptr, false, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeInstantSend, qman, nullptr, true, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeInstantSend, qman, nullptr, true, true), false);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeDIP0024InstantSend, qman, nullptr, false, false), false);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeDIP0024InstantSend, qman, nullptr, true, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeDIP0024InstantSend, qman, nullptr, true, true), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeChainLocks, qman, nullptr, false, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeChainLocks, qman, nullptr, false, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeChainLocks, qman, nullptr, true, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypePlatform, qman, nullptr, true, false), Params().IsTestChain());
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypePlatform, qman, nullptr, true, true), Params().IsTestChain());
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypePlatform, qman, nullptr, true, true), Params().IsTestChain());
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeMnhf, qman, nullptr, true, false), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeMnhf, qman, nullptr, true, true), true);
|
|
BOOST_CHECK_EQUAL(IsQuorumTypeEnabledInternal(consensus_params.llmqTypeMnhf, qman, nullptr, true, true), true);
|
|
}
|
|
|
|
BOOST_FIXTURE_TEST_CASE(utils_IsQuorumTypeEnabled_tests_regtest, RegTestingSetup)
|
|
{
|
|
assert(m_node.llmq_ctx->qman);
|
|
Test(*m_node.llmq_ctx->qman);
|
|
}
|
|
|
|
BOOST_FIXTURE_TEST_CASE(utils_IsQuorumTypeEnabled_tests_mainnet, TestingSetup)
|
|
{
|
|
assert(m_node.llmq_ctx->qman);
|
|
Test(*m_node.llmq_ctx->qman);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|