mirror of
https://github.com/dashpay/dash.git
synced 2024-12-30 22:35:51 +01:00
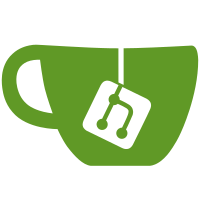
084e17cebd424b8e8ced674bc810eef4e6ee5d3b Remove unused includes (practicalswift) Pull request description: As requested by MarcoFalke in https://github.com/bitcoin/bitcoin/pull/16273#issuecomment-521332089: This PR removes unused includes. Please note that in contrast to #16273 I'm limiting the scope to the trivial cases of pure removals (i.e. no includes added) to make reviewing easier. I'm seeking "Concept ACK":s for this obviously non-urgent minor cleanup. Rationale: * Avoids unnecessary re-compiles in case of header changes. * Makes reasoning about code dependencies easier. * Reduces compile-time memory usage. * Reduces compilation time. * Warm fuzzy feeling of being lean :-) ACKs for top commit: ryanofsky: Code review ACK 084e17cebd424b8e8ced674bc810eef4e6ee5d3b. PR only removes include lines and it still compiles. In the worst case someone might have to explicitly add an include later for something now included implicitly. But maybe some effort was taken to avoid this, and it wouldn't be a tragedy anyway. Tree-SHA512: 89de56edc6ceea4696e9579bccff10c80080821685b9fb4e8c5ef593b6e43cf662f358788701bb09f84867693f66b2e4db035b92b522a0a775f50b7ecffd6a6d
141 lines
4.4 KiB
C++
141 lines
4.4 KiB
C++
// Copyright (c) 2016 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_BLOCKENCODINGS_H
|
|
#define BITCOIN_BLOCKENCODINGS_H
|
|
|
|
#include <primitives/block.h>
|
|
|
|
|
|
class CTxMemPool;
|
|
|
|
// Transaction compression schemes for compact block relay can be introduced by writing
|
|
// an actual formatter here.
|
|
using TransactionCompression = DefaultFormatter;
|
|
|
|
class DifferenceFormatter
|
|
{
|
|
uint64_t m_shift = 0;
|
|
|
|
public:
|
|
template<typename Stream, typename I>
|
|
void Ser(Stream& s, I v)
|
|
{
|
|
if (v < m_shift || v >= std::numeric_limits<uint64_t>::max()) throw std::ios_base::failure("differential value overflow");
|
|
WriteCompactSize(s, v - m_shift);
|
|
m_shift = uint64_t(v) + 1;
|
|
}
|
|
template<typename Stream, typename I>
|
|
void Unser(Stream& s, I& v)
|
|
{
|
|
uint64_t n = ReadCompactSize(s);
|
|
m_shift += n;
|
|
if (m_shift < n || m_shift >= std::numeric_limits<uint64_t>::max() || m_shift < std::numeric_limits<I>::min() || m_shift > std::numeric_limits<I>::max()) throw std::ios_base::failure("differential value overflow");
|
|
v = I(m_shift++);
|
|
}
|
|
};
|
|
|
|
class BlockTransactionsRequest {
|
|
public:
|
|
// A BlockTransactionsRequest message
|
|
uint256 blockhash;
|
|
std::vector<uint16_t> indexes;
|
|
|
|
SERIALIZE_METHODS(BlockTransactionsRequest, obj)
|
|
{
|
|
READWRITE(obj.blockhash, Using<VectorFormatter<DifferenceFormatter>>(obj.indexes));
|
|
}
|
|
};
|
|
|
|
class BlockTransactions {
|
|
public:
|
|
// A BlockTransactions message
|
|
uint256 blockhash;
|
|
std::vector<CTransactionRef> txn;
|
|
|
|
BlockTransactions() {}
|
|
explicit BlockTransactions(const BlockTransactionsRequest& req) :
|
|
blockhash(req.blockhash), txn(req.indexes.size()) {}
|
|
|
|
SERIALIZE_METHODS(BlockTransactions, obj)
|
|
{
|
|
READWRITE(obj.blockhash, Using<VectorFormatter<TransactionCompression>>(obj.txn));
|
|
}
|
|
};
|
|
|
|
// Dumb serialization/storage-helper for CBlockHeaderAndShortTxIDs and PartiallyDownloadedBlock
|
|
struct PrefilledTransaction {
|
|
// Used as an offset since last prefilled tx in CBlockHeaderAndShortTxIDs,
|
|
// as a proper transaction-in-block-index in PartiallyDownloadedBlock
|
|
uint16_t index;
|
|
CTransactionRef tx;
|
|
|
|
SERIALIZE_METHODS(PrefilledTransaction, obj) { READWRITE(COMPACTSIZE(obj.index), Using<TransactionCompression>(obj.tx)); }
|
|
};
|
|
|
|
typedef enum ReadStatus_t
|
|
{
|
|
READ_STATUS_OK,
|
|
READ_STATUS_INVALID, // Invalid object, peer is sending bogus crap
|
|
READ_STATUS_FAILED, // Failed to process object
|
|
READ_STATUS_CHECKBLOCK_FAILED, // Used only by FillBlock to indicate a
|
|
// failure in CheckBlock.
|
|
} ReadStatus;
|
|
|
|
class CBlockHeaderAndShortTxIDs {
|
|
private:
|
|
mutable uint64_t shorttxidk0, shorttxidk1;
|
|
uint64_t nonce;
|
|
|
|
void FillShortTxIDSelector() const;
|
|
|
|
friend class PartiallyDownloadedBlock;
|
|
|
|
protected:
|
|
std::vector<uint64_t> shorttxids;
|
|
std::vector<PrefilledTransaction> prefilledtxn;
|
|
|
|
public:
|
|
static constexpr int SHORTTXIDS_LENGTH = 6;
|
|
|
|
CBlockHeader header;
|
|
|
|
// Dummy for deserialization
|
|
CBlockHeaderAndShortTxIDs() {}
|
|
|
|
CBlockHeaderAndShortTxIDs(const CBlock& block);
|
|
|
|
uint64_t GetShortID(const uint256& txhash) const;
|
|
|
|
size_t BlockTxCount() const { return shorttxids.size() + prefilledtxn.size(); }
|
|
|
|
SERIALIZE_METHODS(CBlockHeaderAndShortTxIDs, obj)
|
|
{
|
|
READWRITE(obj.header, obj.nonce, Using<VectorFormatter<CustomUintFormatter<SHORTTXIDS_LENGTH>>>(obj.shorttxids), obj.prefilledtxn);
|
|
if (ser_action.ForRead()) {
|
|
if (obj.BlockTxCount() > std::numeric_limits<uint16_t>::max()) {
|
|
throw std::ios_base::failure("indexes overflowed 16 bits");
|
|
}
|
|
obj.FillShortTxIDSelector();
|
|
}
|
|
}
|
|
};
|
|
|
|
class PartiallyDownloadedBlock {
|
|
protected:
|
|
std::vector<CTransactionRef> txn_available;
|
|
size_t prefilled_count = 0, mempool_count = 0, extra_count = 0;
|
|
const CTxMemPool* pool;
|
|
public:
|
|
CBlockHeader header;
|
|
explicit PartiallyDownloadedBlock(CTxMemPool* poolIn) : pool(poolIn) {}
|
|
|
|
// extra_txn is a list of extra transactions to look at, in <hash, reference> form
|
|
ReadStatus InitData(const CBlockHeaderAndShortTxIDs& cmpctblock, const std::vector<std::pair<uint256, CTransactionRef>>& extra_txn);
|
|
bool IsTxAvailable(size_t index) const;
|
|
ReadStatus FillBlock(CBlock& block, const std::vector<CTransactionRef>& vtx_missing);
|
|
};
|
|
|
|
#endif // BITCOIN_BLOCKENCODINGS_H
|