mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
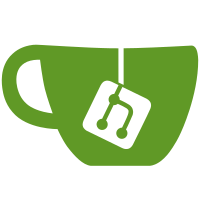
7148b74dc
[tests] Functional tests must explicitly set num_nodes (John Newbery)5448a1471
[tests] don't override __init__() in individual tests (John Newbery)6cf094a02
[tests] Avoid passing around member variables in test_framework (John Newbery)36b626867
[tests] TestNode: separate add_node from start_node (John Newbery)be2a2ab6a
[tests] fix - use rpc_timeout as rpc timeout (John Newbery) Pull request description: Some additional tidyups after the introduction of TestNode: - commit 1 makes TestNode use the correct rpc timeout. This should have been included in #11077 - commit 2 separates `add_node()` from `start_node()` as originally discussed here: https://github.com/bitcoin/bitcoin/pull/10556#discussion_r121161453 with @kallewoof . The test writer no longer needs to assign to `self.nodes` when starting/stopping nodes. - commit 3 adds a `set_test_params()` method, so individual tests don't need to override `__init__()` and call `super().__init__()` Tree-SHA512: 0adb030623b96675b5c29e2890ce99ccd837ed05f721d0c91b35378c5ac01b6658174aac12f1f77402e1d38b61f39b3c43b4df85c96952565dde1cda05b0db84
117 lines
5.0 KiB
Python
Executable File
117 lines
5.0 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2015-2016 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test transaction signing using the signrawtransaction RPC."""
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
|
|
|
|
class SignRawTransactionsTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 1
|
|
|
|
def successful_signing_test(self):
|
|
"""Create and sign a valid raw transaction with one input.
|
|
|
|
Expected results:
|
|
|
|
1) The transaction has a complete set of signatures
|
|
2) No script verification error occurred"""
|
|
privKeys = ['cUeKHd5orzT3mz8P9pxyREHfsWtVfgsfDjiZZBcjUBAaGk1BTj7N', 'cVKpPfVKSJxKqVpE9awvXNWuLHCa5j5tiE7K6zbUSptFpTEtiFrA']
|
|
|
|
inputs = [
|
|
# Valid pay-to-pubkey scripts
|
|
{'txid': '9b907ef1e3c26fc71fe4a4b3580bc75264112f95050014157059c736f0202e71', 'vout': 0,
|
|
'scriptPubKey': '76a91460baa0f494b38ce3c940dea67f3804dc52d1fb9488ac'},
|
|
{'txid': '83a4f6a6b73660e13ee6cb3c6063fa3759c50c9b7521d0536022961898f4fb02', 'vout': 0,
|
|
'scriptPubKey': '76a914669b857c03a5ed269d5d85a1ffac9ed5d663072788ac'},
|
|
]
|
|
|
|
outputs = {'ycwedq2f3sz2Yf9JqZsBCQPxp18WU3Hp4J': 0.1}
|
|
|
|
rawTx = self.nodes[0].createrawtransaction(inputs, outputs)
|
|
rawTxSigned = self.nodes[0].signrawtransaction(rawTx, inputs, privKeys)
|
|
|
|
# 1) The transaction has a complete set of signatures
|
|
assert 'complete' in rawTxSigned
|
|
assert_equal(rawTxSigned['complete'], True)
|
|
|
|
# 2) No script verification error occurred
|
|
assert 'errors' not in rawTxSigned
|
|
|
|
def script_verification_error_test(self):
|
|
"""Create and sign a raw transaction with valid (vin 0), invalid (vin 1) and one missing (vin 2) input script.
|
|
|
|
Expected results:
|
|
|
|
3) The transaction has no complete set of signatures
|
|
4) Two script verification errors occurred
|
|
5) Script verification errors have certain properties ("txid", "vout", "scriptSig", "sequence", "error")
|
|
6) The verification errors refer to the invalid (vin 1) and missing input (vin 2)"""
|
|
privKeys = ['cUeKHd5orzT3mz8P9pxyREHfsWtVfgsfDjiZZBcjUBAaGk1BTj7N']
|
|
|
|
inputs = [
|
|
# Valid pay-to-pubkey script
|
|
{'txid': '9b907ef1e3c26fc71fe4a4b3580bc75264112f95050014157059c736f0202e71', 'vout': 0},
|
|
# Invalid script
|
|
{'txid': '5b8673686910442c644b1f4993d8f7753c7c8fcb5c87ee40d56eaeef25204547', 'vout': 7},
|
|
# Missing scriptPubKey
|
|
{'txid': '9b907ef1e3c26fc71fe4a4b3580bc75264112f95050014157059c736f0202e71', 'vout': 1},
|
|
]
|
|
|
|
scripts = [
|
|
# Valid pay-to-pubkey script
|
|
{'txid': '9b907ef1e3c26fc71fe4a4b3580bc75264112f95050014157059c736f0202e71', 'vout': 0,
|
|
'scriptPubKey': '76a91460baa0f494b38ce3c940dea67f3804dc52d1fb9488ac'},
|
|
# Invalid script
|
|
{'txid': '5b8673686910442c644b1f4993d8f7753c7c8fcb5c87ee40d56eaeef25204547', 'vout': 7,
|
|
'scriptPubKey': 'badbadbadbad'}
|
|
]
|
|
|
|
outputs = {'ycwedq2f3sz2Yf9JqZsBCQPxp18WU3Hp4J': 0.1}
|
|
|
|
rawTx = self.nodes[0].createrawtransaction(inputs, outputs)
|
|
|
|
# Make sure decoderawtransaction is at least marginally sane
|
|
decodedRawTx = self.nodes[0].decoderawtransaction(rawTx)
|
|
for i, inp in enumerate(inputs):
|
|
assert_equal(decodedRawTx["vin"][i]["txid"], inp["txid"])
|
|
assert_equal(decodedRawTx["vin"][i]["vout"], inp["vout"])
|
|
|
|
# Make sure decoderawtransaction throws if there is extra data
|
|
assert_raises(JSONRPCException, self.nodes[0].decoderawtransaction, rawTx + "00")
|
|
|
|
rawTxSigned = self.nodes[0].signrawtransaction(rawTx, scripts, privKeys)
|
|
|
|
# 3) The transaction has no complete set of signatures
|
|
assert 'complete' in rawTxSigned
|
|
assert_equal(rawTxSigned['complete'], False)
|
|
|
|
# 4) Two script verification errors occurred
|
|
assert 'errors' in rawTxSigned
|
|
assert_equal(len(rawTxSigned['errors']), 2)
|
|
|
|
# 5) Script verification errors have certain properties
|
|
assert 'txid' in rawTxSigned['errors'][0]
|
|
assert 'vout' in rawTxSigned['errors'][0]
|
|
assert 'scriptSig' in rawTxSigned['errors'][0]
|
|
assert 'sequence' in rawTxSigned['errors'][0]
|
|
assert 'error' in rawTxSigned['errors'][0]
|
|
|
|
# 6) The verification errors refer to the invalid (vin 1) and missing input (vin 2)
|
|
assert_equal(rawTxSigned['errors'][0]['txid'], inputs[1]['txid'])
|
|
assert_equal(rawTxSigned['errors'][0]['vout'], inputs[1]['vout'])
|
|
assert_equal(rawTxSigned['errors'][1]['txid'], inputs[2]['txid'])
|
|
assert_equal(rawTxSigned['errors'][1]['vout'], inputs[2]['vout'])
|
|
|
|
def run_test(self):
|
|
self.successful_signing_test()
|
|
self.script_verification_error_test()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
SignRawTransactionsTest().main()
|