mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
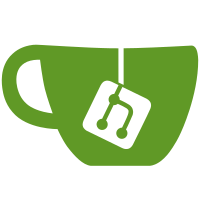
* scripted-diff: Replace #include "" with #include <> (ryanofsky) -BEGIN VERIFY SCRIPT- for f in \ src/*.cpp \ src/*.h \ src/bench/*.cpp \ src/bench/*.h \ src/compat/*.cpp \ src/compat/*.h \ src/consensus/*.cpp \ src/consensus/*.h \ src/crypto/*.cpp \ src/crypto/*.h \ src/crypto/ctaes/*.h \ src/policy/*.cpp \ src/policy/*.h \ src/primitives/*.cpp \ src/primitives/*.h \ src/qt/*.cpp \ src/qt/*.h \ src/qt/test/*.cpp \ src/qt/test/*.h \ src/rpc/*.cpp \ src/rpc/*.h \ src/script/*.cpp \ src/script/*.h \ src/support/*.cpp \ src/support/*.h \ src/support/allocators/*.h \ src/test/*.cpp \ src/test/*.h \ src/wallet/*.cpp \ src/wallet/*.h \ src/wallet/test/*.cpp \ src/wallet/test/*.h \ src/zmq/*.cpp \ src/zmq/*.h do base=${f%/*}/ relbase=${base#src/} sed -i "s:#include \"\(.*\)\"\(.*\):if test -e \$base'\\1'; then echo \"#include <\"\$relbase\"\\1>\\2\"; else echo \"#include <\\1>\\2\"; fi:e" $f done -END VERIFY SCRIPT- Signed-off-by: Pasta <pasta@dashboost.org> * scripted-diff: Replace #include "" with #include <> (Dash Specific) -BEGIN VERIFY SCRIPT- for f in \ src/bls/*.cpp \ src/bls/*.h \ src/evo/*.cpp \ src/evo/*.h \ src/governance/*.cpp \ src/governance/*.h \ src/llmq/*.cpp \ src/llmq/*.h \ src/masternode/*.cpp \ src/masternode/*.h \ src/privatesend/*.cpp \ src/privatesend/*.h do base=${f%/*}/ relbase=${base#src/} sed -i "s:#include \"\(.*\)\"\(.*\):if test -e \$base'\\1'; then echo \"#include <\"\$relbase\"\\1>\\2\"; else echo \"#include <\\1>\\2\"; fi:e" $f done -END VERIFY SCRIPT- Signed-off-by: Pasta <pasta@dashboost.org> * build: Remove -I for everything but project root Remove -I from build system for everything but the project root, and built-in dependencies. Signed-off-by: Pasta <pasta@dashboost.org> # Conflicts: # src/Makefile.test.include * qt: refactor: Use absolute include paths in .ui files * qt: refactor: Changes to make include paths absolute This makes all include paths in the GUI absolute. Many changes are involved as every single source file in src/qt/ assumes to be able to use relative includes. Signed-off-by: Pasta <pasta@dashboost.org> # Conflicts: # src/qt/dash.cpp # src/qt/optionsmodel.cpp # src/qt/test/rpcnestedtests.cpp * test: refactor: Use absolute include paths for test data files * Recommend #include<> syntax in developer notes * refactor: Include obj/build.h instead of build.h * END BACKPORT #11651 Remove trailing whitespace causing travis failure * fix backport 11651 Signed-off-by: Pasta <pasta@dashboost.org> * More of 11651 * fix blockchain.cpp Signed-off-by: pasta <pasta@dashboost.org> * Add missing "qt/" in includes * Add missing "test/" in includes * Fix trailing whitespaces Co-authored-by: Wladimir J. van der Laan <laanwj@gmail.com> Co-authored-by: Russell Yanofsky <russ@yanofsky.org> Co-authored-by: MeshCollider <dobsonsa68@gmail.com> Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
68 lines
2.3 KiB
C++
68 lines
2.3 KiB
C++
// Copyright (c) 2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_CORE_MEMUSAGE_H
|
|
#define BITCOIN_CORE_MEMUSAGE_H
|
|
|
|
#include <primitives/transaction.h>
|
|
#include <primitives/block.h>
|
|
#include <memusage.h>
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CScript& script) {
|
|
return memusage::DynamicUsage(script);
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const COutPoint& out) {
|
|
return 0;
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CTxIn& in) {
|
|
return RecursiveDynamicUsage(in.scriptSig) + RecursiveDynamicUsage(in.prevout);
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CTxOut& out) {
|
|
return RecursiveDynamicUsage(out.scriptPubKey);
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CTransaction& tx) {
|
|
size_t mem = memusage::DynamicUsage(tx.vin) + memusage::DynamicUsage(tx.vout);
|
|
for (std::vector<CTxIn>::const_iterator it = tx.vin.begin(); it != tx.vin.end(); it++) {
|
|
mem += RecursiveDynamicUsage(*it);
|
|
}
|
|
for (std::vector<CTxOut>::const_iterator it = tx.vout.begin(); it != tx.vout.end(); it++) {
|
|
mem += RecursiveDynamicUsage(*it);
|
|
}
|
|
return mem;
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CMutableTransaction& tx) {
|
|
size_t mem = memusage::DynamicUsage(tx.vin) + memusage::DynamicUsage(tx.vout);
|
|
for (std::vector<CTxIn>::const_iterator it = tx.vin.begin(); it != tx.vin.end(); it++) {
|
|
mem += RecursiveDynamicUsage(*it);
|
|
}
|
|
for (std::vector<CTxOut>::const_iterator it = tx.vout.begin(); it != tx.vout.end(); it++) {
|
|
mem += RecursiveDynamicUsage(*it);
|
|
}
|
|
return mem;
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CBlock& block) {
|
|
size_t mem = memusage::DynamicUsage(block.vtx);
|
|
for (const auto& tx : block.vtx) {
|
|
mem += memusage::DynamicUsage(tx) + RecursiveDynamicUsage(*tx);
|
|
}
|
|
return mem;
|
|
}
|
|
|
|
static inline size_t RecursiveDynamicUsage(const CBlockLocator& locator) {
|
|
return memusage::DynamicUsage(locator.vHave);
|
|
}
|
|
|
|
template<typename X>
|
|
static inline size_t RecursiveDynamicUsage(const std::shared_ptr<X>& p) {
|
|
return p ? memusage::DynamicUsage(p) + RecursiveDynamicUsage(*p) : 0;
|
|
}
|
|
|
|
#endif // BITCOIN_CORE_MEMUSAGE_H
|