mirror of
https://github.com/dashpay/dash.git
synced 2024-12-25 20:12:57 +01:00
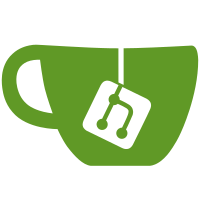
* Merge #11796: [tests] Functional test naming convention5fecd84
[tests] Remove redundant import in blocktools.py test (Anthony Towns)9b20bb4
[tests] Check tests conform to naming convention (Anthony Towns)7250b4e
[tests] README.md nit fixes (Anthony Towns)82b2712
[tests] move witness util functions to blocktools.py (John Newbery)1e10854
[tests] [docs] update README for new test naming scheme (John Newbery) Pull request description: Splitting #11774 into two parts -- this part updates the README with the proposed naming convention, and adds some checks to test_runner.py that the number of tests violating the naming convention doesn't increase too much. Idea is this part of the change should not introduce merge conflicts or require much rebasing, so reviews of the complicated bits won't become invalidated too often; while the second part will just be file renames, which will require regular rebasing and will introduce merge conflicts with pending PRs, but can be merged later, and should also be much easier to review, since it will only include relatively trivial changes. Tree-SHA512: b96557d41714addbbfe2aed62fb5a48639eaeb1eb3aba30ac1b3a86bb3cb8d796c6247f9c414c4695c4bf54c0ec9968ac88e2f88fb62483bc1a2f89368f7fc80 * update violation count Signed-off-by: pasta <pasta@dashboost.org> * Merge #11774: [tests] Rename functional tests6f881cc880
[tests] Remove EXPECTED_VIOLATION_COUNT (Anthony Towns)3150b3fea7
[tests] Rename misc functional tests. (Anthony Towns)81b79f2c39
[tests] Rename rpc_* functional tests. (Anthony Towns)61b8f7f273
[tests] Rename p2p_* functional tests. (Anthony Towns)90600bc7db
[tests] Rename wallet_* functional tests. (Anthony Towns)ca6523d0c8
[tests] Rename feature_* functional tests. (Anthony Towns) Pull request description: This PR changes the functional tests to have a consistent naming scheme: tests for individual RPC methods are named rpc_... tests for interfaces (REST, ZMQ, RPC features) are named interface_... tests that explicitly test the p2p interface are named p2p_... tests for wallet features are named wallet_... tests for mining features are named mining_... tests for mempool behaviour are named mempool_... tests for full features that aren't wallet/mining/mempool are named feature_... Rationale: it's sometimes difficult for new contributors to know what's already covered by existing tests and where new tests should be added. Naming in a consistent fashion makes it easier to see what's already covered at a glance. Tree-SHA512: 4246790552d42bbd95f6d5bdf67702b81b3b2c583ce7eaf1fe6d8e254721279b47315973c6e9ae82dad6e4c747f12188160764bf2624c0f8f3b4d39330ec8b16 * rename tests and edit associated strings to align test-suite with test name standards Signed-off-by: pasta <pasta@dashboost.org> * fix grammar in test/functional/test_runner.py Co-authored-by: dustinface <35775977+xdustinface@users.noreply.github.com> * ci: Fix excluded test names * rename feature_privatesend.py to rpc_privatesend.py Signed-off-by: pasta <pasta@dashboost.org> Co-authored-by: Wladimir J. van der Laan <laanwj@gmail.com> Co-authored-by: MarcoFalke <falke.marco@gmail.com> Co-authored-by: dustinface <35775977+xdustinface@users.noreply.github.com> Co-authored-by: xdustinface <xdustinfacex@gmail.com>
208 lines
7.3 KiB
Python
Executable File
208 lines
7.3 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2014-2016 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test the wallet backup features.
|
|
|
|
Test case is:
|
|
4 nodes. 1 2 and 3 send transactions between each other,
|
|
fourth node is a miner.
|
|
1 2 3 each mine a block to start, then
|
|
Miner creates 100 blocks so 1 2 3 each have 500 mature
|
|
coins to spend.
|
|
Then 5 iterations of 1/2/3 sending coins amongst
|
|
themselves to get transactions in the wallets,
|
|
and the miner mining one block.
|
|
|
|
Wallets are backed up using dumpwallet/backupwallet.
|
|
Then 5 more iterations of transactions and mining a block.
|
|
|
|
Miner then generates 101 more blocks, so any
|
|
transaction fees paid mature.
|
|
|
|
Sanity check:
|
|
Sum(1,2,3,4 balances) == 114*500
|
|
|
|
1/2/3 are shutdown, and their wallets erased.
|
|
Then restore using wallet.dat backup. And
|
|
confirm 1/2/3/4 balances are same as before.
|
|
|
|
Shutdown again, restore using importwallet,
|
|
and confirm again balances are correct.
|
|
"""
|
|
from random import randint
|
|
import shutil
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
|
|
class WalletBackupTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 4
|
|
self.setup_clean_chain = True
|
|
# nodes 1, 2,3 are spenders, let's give them a keypool=100
|
|
self.extra_args = [["-keypool=100"], ["-keypool=100"], ["-keypool=100"], []]
|
|
|
|
def setup_network(self, split=False):
|
|
self.setup_nodes()
|
|
connect_nodes(self.nodes[0], 3)
|
|
connect_nodes(self.nodes[1], 3)
|
|
connect_nodes(self.nodes[2], 3)
|
|
connect_nodes(self.nodes[2], 0)
|
|
self.sync_all()
|
|
|
|
def one_send(self, from_node, to_address):
|
|
if (randint(1,2) == 1):
|
|
amount = Decimal(randint(1,10)) / Decimal(10)
|
|
self.nodes[from_node].sendtoaddress(to_address, amount)
|
|
|
|
def do_one_round(self):
|
|
a0 = self.nodes[0].getnewaddress()
|
|
a1 = self.nodes[1].getnewaddress()
|
|
a2 = self.nodes[2].getnewaddress()
|
|
|
|
self.one_send(0, a1)
|
|
self.one_send(0, a2)
|
|
self.one_send(1, a0)
|
|
self.one_send(1, a2)
|
|
self.one_send(2, a0)
|
|
self.one_send(2, a1)
|
|
|
|
# Have the miner (node3) mine a block.
|
|
# Must sync mempools before mining.
|
|
self.sync_mempools()
|
|
self.nodes[3].generate(1)
|
|
self.sync_blocks()
|
|
|
|
# As above, this mirrors the original bash test.
|
|
def start_three(self):
|
|
self.start_node(0)
|
|
self.start_node(1)
|
|
self.start_node(2)
|
|
connect_nodes(self.nodes[0], 3)
|
|
connect_nodes(self.nodes[1], 3)
|
|
connect_nodes(self.nodes[2], 3)
|
|
connect_nodes(self.nodes[2], 0)
|
|
|
|
def stop_three(self):
|
|
self.stop_node(0)
|
|
self.stop_node(1)
|
|
self.stop_node(2)
|
|
|
|
def erase_three(self):
|
|
os.remove(self.options.tmpdir + "/node0/regtest/wallets/wallet.dat")
|
|
os.remove(self.options.tmpdir + "/node1/regtest/wallets/wallet.dat")
|
|
os.remove(self.options.tmpdir + "/node2/regtest/wallets/wallet.dat")
|
|
|
|
def run_test(self):
|
|
self.log.info("Generating initial blockchain")
|
|
self.nodes[0].generate(1)
|
|
self.sync_blocks()
|
|
self.nodes[1].generate(1)
|
|
self.sync_blocks()
|
|
self.nodes[2].generate(1)
|
|
self.sync_blocks()
|
|
self.nodes[3].generate(100)
|
|
self.sync_blocks()
|
|
|
|
assert_equal(self.nodes[0].getbalance(), 500)
|
|
assert_equal(self.nodes[1].getbalance(), 500)
|
|
assert_equal(self.nodes[2].getbalance(), 500)
|
|
assert_equal(self.nodes[3].getbalance(), 0)
|
|
|
|
self.log.info("Creating transactions")
|
|
# Five rounds of sending each other transactions.
|
|
for i in range(5):
|
|
self.do_one_round()
|
|
|
|
self.log.info("Backing up")
|
|
tmpdir = self.options.tmpdir
|
|
self.nodes[0].backupwallet(tmpdir + "/node0/wallet.bak")
|
|
self.nodes[0].dumpwallet(tmpdir + "/node0/wallet.dump")
|
|
self.nodes[1].backupwallet(tmpdir + "/node1/wallet.bak")
|
|
self.nodes[1].dumpwallet(tmpdir + "/node1/wallet.dump")
|
|
self.nodes[2].backupwallet(tmpdir + "/node2/wallet.bak")
|
|
self.nodes[2].dumpwallet(tmpdir + "/node2/wallet.dump")
|
|
|
|
self.log.info("More transactions")
|
|
for i in range(5):
|
|
self.do_one_round()
|
|
|
|
# Generate 101 more blocks, so any fees paid mature
|
|
self.nodes[3].generate(101)
|
|
self.sync_all()
|
|
|
|
balance0 = self.nodes[0].getbalance()
|
|
balance1 = self.nodes[1].getbalance()
|
|
balance2 = self.nodes[2].getbalance()
|
|
balance3 = self.nodes[3].getbalance()
|
|
total = balance0 + balance1 + balance2 + balance3
|
|
|
|
# At this point, there are 214 blocks (103 for setup, then 10 rounds, then 101.)
|
|
# 114 are mature, so the sum of all wallets should be 114 * 500 = 57000.
|
|
assert_equal(total, 57000)
|
|
|
|
##
|
|
# Test restoring spender wallets from backups
|
|
##
|
|
self.log.info("Restoring using wallet.dat")
|
|
self.stop_three()
|
|
self.erase_three()
|
|
|
|
# Start node2 with no chain
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/blocks")
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/chainstate")
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/evodb")
|
|
|
|
# Restore wallets from backup
|
|
shutil.copyfile(tmpdir + "/node0/wallet.bak", tmpdir + "/node0/regtest/wallets/wallet.dat")
|
|
shutil.copyfile(tmpdir + "/node1/wallet.bak", tmpdir + "/node1/regtest/wallets/wallet.dat")
|
|
shutil.copyfile(tmpdir + "/node2/wallet.bak", tmpdir + "/node2/regtest/wallets/wallet.dat")
|
|
|
|
self.log.info("Re-starting nodes")
|
|
self.start_three()
|
|
self.sync_blocks()
|
|
|
|
assert_equal(self.nodes[0].getbalance(), balance0)
|
|
assert_equal(self.nodes[1].getbalance(), balance1)
|
|
assert_equal(self.nodes[2].getbalance(), balance2)
|
|
|
|
self.log.info("Restoring using dumped wallet")
|
|
self.stop_three()
|
|
self.erase_three()
|
|
|
|
#start node2 with no chain
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/blocks")
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/chainstate")
|
|
shutil.rmtree(self.options.tmpdir + "/node2/regtest/evodb")
|
|
|
|
self.start_three()
|
|
|
|
assert_equal(self.nodes[0].getbalance(), 0)
|
|
assert_equal(self.nodes[1].getbalance(), 0)
|
|
assert_equal(self.nodes[2].getbalance(), 0)
|
|
|
|
self.nodes[0].importwallet(tmpdir + "/node0/wallet.dump")
|
|
self.nodes[1].importwallet(tmpdir + "/node1/wallet.dump")
|
|
self.nodes[2].importwallet(tmpdir + "/node2/wallet.dump")
|
|
|
|
self.sync_blocks()
|
|
|
|
assert_equal(self.nodes[0].getbalance(), balance0)
|
|
assert_equal(self.nodes[1].getbalance(), balance1)
|
|
assert_equal(self.nodes[2].getbalance(), balance2)
|
|
|
|
# Backup to source wallet file must fail
|
|
sourcePaths = [
|
|
tmpdir + "/node0/regtest/wallets/wallet.dat",
|
|
tmpdir + "/node0/./regtest/wallets/wallet.dat",
|
|
tmpdir + "/node0/regtest/wallets/",
|
|
tmpdir + "/node0/regtest/wallets"]
|
|
|
|
for sourcePath in sourcePaths:
|
|
assert_raises_rpc_error(-4, "backup failed", self.nodes[0].backupwallet, sourcePath)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
WalletBackupTest().main()
|