mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
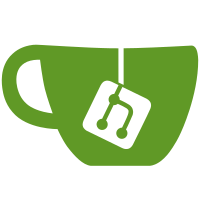
* Remove ppszTypeName from protocol.cpp and reimplement GetCommand This removes the need to carefully maintain ppszTypeName, which required correct order and also did not allow to permanently remove old message types. To get the command name for an INV type, GetCommandInternal uses a switch which needs to be maintained from now on. The way this is implemented also resembles the way it is implemented in Bitcoin today, but it's not identical. The original PR that introduced the switch case in Bitcoin was part of the Segwit changes and thus never got backported. I decided to implement it in a slightly different way that avoids throwing exceptions when an unknown INV type is encountered. IsKnownType will now also leverage GetCommandInternal() to figure out if the INV type is known locally. This has the side effect of old/legacy message types to return false from now on. We will depend on this side effect in later commits when we remove legacy InstantSend code. * Stop handling/relaying legacy IX messages When we receive an IX message, we simply treat it as a regular TX and relay it as such. We'll however still request IX messages when they are announced to us. We can't simply revert to requesting TX messages in this case as it might result in the other peer not answering due to the TX not being in mapRelay yet. We should at some point in the future completely drop handling of IX messages instead. * Remove IsNewInstantSendEnabled() and only use IsInstantSendEnabled() * Remove legacy InstantSend from GUI * Remove InstantSend from Bitcoin/Dash URIs * Remove legacy InstantSend from RPC commands * Remove legacy InstantSend from wallet * Remove legacy instantsend.h include * Remove legacy InstantSend from validation code * Completely remove remaining legacy InstantSend code * Remove now unused spork * Fix InstantSend related test failures * Remove now obsolete auto IS tests * Make spork2 and spork3 disabled by default This should have no influence on mainnet as these sporks are actually set there. This will however affect regtest, which shouldn't have LLMQ based InstantSend enabled by default. * Remove instantsend tests from dip3-deterministicmns.py These were only testing legacy InstantSend * Fix .QCheckBox#checkUsePrivateSend styling a bit * s/TXLEGACYLOCKREQUEST/LEGACYTXLOCKREQUEST/ * Revert "verified via InstantSend" back to "verified via LLMQ based InstantSend" * Use cmd == nullptr instead of !cmd * Remove last parameter from AvailableCoins call This was for fUseInstantSend which is not present anymore since rebase
89 lines
2.8 KiB
Python
Executable File
89 lines
2.8 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2018 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.mininode import *
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
from time import *
|
|
|
|
'''
|
|
'''
|
|
|
|
class SporkTest(BitcoinTestFramework):
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.num_nodes = 3
|
|
self.setup_clean_chain = True
|
|
self.extra_args = [["-sporkkey=cP4EKFyJsHT39LDqgdcB43Y3YXjNyjb5Fuas1GQSeAtjnZWmZEQK"], [], []]
|
|
|
|
def setup_network(self):
|
|
disable_mocktime()
|
|
self.setup_nodes()
|
|
# connect only 2 first nodes at start
|
|
connect_nodes(self.nodes[0], 1)
|
|
|
|
def get_test_spork_state(self, node):
|
|
info = node.spork('active')
|
|
# use InstantSend spork for tests
|
|
return info['SPORK_2_INSTANTSEND_ENABLED']
|
|
|
|
def set_test_spork_state(self, node, state):
|
|
if state:
|
|
value = 0
|
|
else:
|
|
value = 4070908800
|
|
# use InstantSend spork for tests
|
|
node.spork('SPORK_2_INSTANTSEND_ENABLED', value)
|
|
|
|
def run_test(self):
|
|
# check test spork default state
|
|
assert(not self.get_test_spork_state(self.nodes[0]))
|
|
assert(not self.get_test_spork_state(self.nodes[1]))
|
|
assert(not self.get_test_spork_state(self.nodes[2]))
|
|
|
|
# check spork propagation for connected nodes
|
|
self.set_test_spork_state(self.nodes[0], True)
|
|
start = time()
|
|
sent = False
|
|
while True:
|
|
if self.get_test_spork_state(self.nodes[1]):
|
|
sent = True
|
|
break
|
|
if time() > start + 10:
|
|
break
|
|
sleep(0.1)
|
|
assert(sent)
|
|
|
|
# restart nodes to check spork persistence
|
|
self.stop_node(0)
|
|
self.stop_node(1)
|
|
self.nodes[0] = self.start_node(0, self.options.tmpdir)
|
|
self.nodes[1] = self.start_node(1, self.options.tmpdir)
|
|
assert(self.get_test_spork_state(self.nodes[0]))
|
|
assert(self.get_test_spork_state(self.nodes[1]))
|
|
|
|
# Force finish mnsync node as otherwise it will never send out headers to other peers
|
|
wait_to_sync(self.nodes[1], fast_mnsync=True)
|
|
|
|
# Generate one block to kick off masternode sync, which also starts sporks syncing for node2
|
|
self.nodes[1].generate(1)
|
|
|
|
# connect new node and check spork propagation after restoring from cache
|
|
connect_nodes(self.nodes[1], 2)
|
|
start = time()
|
|
sent = False
|
|
while True:
|
|
if self.get_test_spork_state(self.nodes[2]):
|
|
sent = True
|
|
break
|
|
if time() > start + 10:
|
|
break
|
|
sleep(0.1)
|
|
assert(sent)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
SporkTest().main()
|