mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
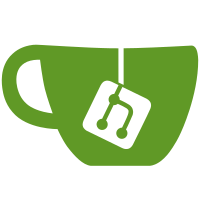
643aad17fa
Enable additional flake8 rules (practicalswift)f020aca297
Minor Python cleanups to make flake8 pass with the new rules enabled (practicalswift) Pull request description: Enabled rules: ``` * E242: tab after ',' * E266: too many leading '#' for block comment * E401: multiple imports on one line * E402: module level import not at top of file * E701: multiple statements on one line (colon) * E901: SyntaxError: invalid syntax * E902: TokenError: EOF in multi-line string * F821: undefined name 'Foo' * W293: blank line contains whitespace * W606: 'async' and 'await' are reserved keywords starting with Python 3.7 ``` Note to reviewers: * In general we don't allow whitespace cleanups to existing code, but in order to allow for enabling Travis checking for these rules a few smaller whitespace cleanups had to made as part of this PR. * Use [this `?w=1` link](https://github.com/bitcoin/bitcoin/pull/12987/files?w=1) to show a diff without whitespace changes. Before this commit: ``` $ flake8 -qq --statistics --ignore=B,C,E,F,I,N,W --select=E112,E113,E115,E116,E125,E131,E133,E223,E224,E242,E266,E271,E272,E273,E274,E275,E304,E306,E401,E402,E502,E701,E702,E703,E714,E721,E741,E742,E743,F401,E901,E902,F402,F404,F406,F407,F601,F602,F621,F622,F631,F701,F702,F703,F704,F705,F706,F707,F811,F812,F821,F822,F823,F831,F841,W292,W293,W504,W601,W602,W603,W604,W605,W606 . 5 E266 too many leading '#' for block comment 4 E401 multiple imports on one line 6 E402 module level import not at top of file 5 E701 multiple statements on one line (colon) 1 F812 list comprehension redefines 'n' from line 159 4 F821 undefined name 'ConnectionRefusedError' 28 W293 blank line contains whitespace ``` After this commit: ``` $ flake8 -qq --statistics --ignore=B,C,E,F,I,N,W --select=E112,E113,E115,E116,E125,E131,E133,E223,E224,E242,E266,E271,E272,E273,E274,E275,E304,E306,E401,E402,E502,E701,E702,E703,E714,E721,E741,E742,E743,F401,E901,E902,F402,F404,F406,F407,F601,F602,F621,F622,F631,F701,F702,F703,F704,F705,F706,F707,F811,F812,F821,F822,F823,F831,F841,W292,W293,W504,W601,W602,W603,W604,W605,W606 . $ ``` Tree-SHA512: fc7d5e752298a50d4248afc620ee2c173135b4ca008e48e02913ac968e5a24a5fd5396926047ec62f1d580d537434ccae01f249bb2f3338fa59dc630bf97ca7a Signed-off-by: pasta <pasta@dashboost.org>
45 lines
1.1 KiB
Python
45 lines
1.1 KiB
Python
#!/usr/bin/env python3
|
|
#
|
|
|
|
try:
|
|
import gdb
|
|
except ImportError as e:
|
|
raise ImportError("This script must be run in GDB: ", str(e))
|
|
import traceback
|
|
import sys
|
|
import os
|
|
import common_helpers
|
|
sys.path.append(os.getcwd())
|
|
|
|
|
|
class UsedSizeCommand (gdb.Command):
|
|
"""calc size of the memory used by the object"""
|
|
|
|
def __init__ (self):
|
|
super (UsedSizeCommand, self).__init__ ("usedsize", gdb.COMMAND_USER)
|
|
|
|
@classmethod
|
|
def assign_value(cls, obj_name, value):
|
|
gdb.execute("set " + obj_name + " = " + str(value))
|
|
|
|
@classmethod
|
|
def get_type(cls, obj_name):
|
|
return gdb.parse_and_eval(obj_name).type
|
|
|
|
def invoke(self, arg, from_tty):
|
|
try:
|
|
args = gdb.string_to_argv(arg)
|
|
obj = gdb.parse_and_eval(args[1])
|
|
obj_type = obj.type
|
|
print (args[1] + " is " + str(obj_type))
|
|
size = common_helpers.get_instance_size(obj)
|
|
UsedSizeCommand.assign_value(args[0], size)
|
|
print (size)
|
|
|
|
except Exception as e:
|
|
print(traceback.format_exc())
|
|
raise e
|
|
|
|
UsedSizeCommand()
|
|
|