mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
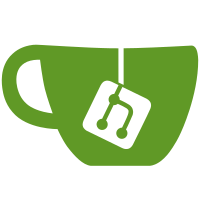
## Issue being fixed or feature implemented Current implementation relies either on asserts or sometimes checks then returning a special value; In the case of asserts (or no assert where we use the value without checks) it'd be better to make it explicit to function caller that the ptr must be not_null; otherwise gsl::not_null will call terminate. See https://github.com/microsoft/GSL/blob/main/docs/headers.md#user-content-H-pointers-not_null and https://isocpp.github.io/CppCoreGuidelines/CppCoreGuidelines#Rf-nullptr I'm interested in a conceptual review; specifically on if this is beneficial over just converting these ptrs to be a reference? ## What was done? *Partial* implementation on using gsl::not_null in dash code ## How Has This Been Tested? Building ## Breaking Changes None ## Checklist: _Go over all the following points, and put an `x` in all the boxes that apply._ - [x] I have performed a self-review of my own code - [ ] I have commented my code, particularly in hard-to-understand areas - [ ] I have added or updated relevant unit/integration/functional/e2e tests - [ ] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone _(for repository code-owners and collaborators only)_ --------- Signed-off-by: pasta <pasta@dashboost.org> Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
77 lines
2.7 KiB
C++
77 lines
2.7 KiB
C++
// Copyright (c) 2023 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
// Originally from https://github.com/paweldac/source_location
|
|
|
|
#ifndef BITCOIN_SOURCE_LOCATION_H
|
|
#define BITCOIN_SOURCE_LOCATION_H
|
|
|
|
#pragma once
|
|
|
|
#include <cstdint>
|
|
|
|
namespace nostd {
|
|
struct source_location {
|
|
public:
|
|
#if defined(__clang__) and (__clang_major__ >= 9)
|
|
static constexpr source_location current(const char* fileName = __builtin_FILE(),
|
|
const char* functionName = __builtin_FUNCTION(),
|
|
const uint_least32_t lineNumber = __builtin_LINE(),
|
|
const uint_least32_t columnOffset = __builtin_COLUMN()) noexcept
|
|
#elif defined(__GNUC__) and (__GNUC__ > 4 or (__GNUC__ == 4 and __GNUC_MINOR__ >= 8))
|
|
static constexpr source_location current(const char* fileName = __builtin_FILE(),
|
|
const char* functionName = __builtin_FUNCTION(),
|
|
const uint_least32_t lineNumber = __builtin_LINE(),
|
|
const uint_least32_t columnOffset = 0) noexcept
|
|
#else
|
|
static constexpr source_location current(const char* fileName = "unsupported",
|
|
const char* functionName = "unsupported",
|
|
const uint_least32_t lineNumber = 0,
|
|
const uint_least32_t columnOffset = 0) noexcept
|
|
#endif
|
|
{
|
|
return source_location(fileName, functionName, lineNumber, columnOffset);
|
|
}
|
|
|
|
source_location(const source_location&) = default;
|
|
source_location(source_location&&) = default;
|
|
|
|
constexpr const char* file_name() const noexcept
|
|
{
|
|
return fileName;
|
|
}
|
|
|
|
constexpr const char* function_name() const noexcept
|
|
{
|
|
return functionName;
|
|
}
|
|
|
|
constexpr uint_least32_t line() const noexcept
|
|
{
|
|
return lineNumber;
|
|
}
|
|
|
|
constexpr std::uint_least32_t column() const noexcept
|
|
{
|
|
return columnOffset;
|
|
}
|
|
|
|
private:
|
|
constexpr source_location(const char* fileName, const char* functionName, const uint_least32_t lineNumber,
|
|
const uint_least32_t columnOffset) noexcept
|
|
: fileName(fileName)
|
|
, functionName(functionName)
|
|
, lineNumber(lineNumber)
|
|
, columnOffset(columnOffset)
|
|
{
|
|
}
|
|
|
|
const char* fileName;
|
|
const char* functionName;
|
|
const std::uint_least32_t lineNumber;
|
|
const std::uint_least32_t columnOffset;
|
|
};
|
|
} // namespace nostd
|
|
|
|
#endif // BITCOIN_SOURCE_LOCATION_H
|