mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
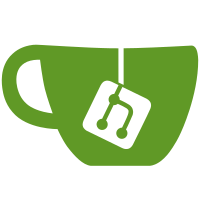
* Merge 9a3a984bb Merge #14272: init: Remove deprecated args from hidden args fa910e4301 init: Remove deprecated args from hidden args (MarcoFalke) Pull request description: The args have been deprecated since 0.17 (maybe longer) and since we reject unknown args, there is no need to add deprecated args to the list of hidden args and then hand-craft an error message if a user provides them. Tree-SHA512: 3a3191439ab0d7969fb72801d097bd86998524f84b3819380224f746cbe4b0f57beec1ad34744424f6587038035b0ddf418ad13171a8d9c3b97b4f3b7b3222a3 * Address review comment Modified hidden_args in SetupServerArgs() Removed usehd from list of unsupported/deprecated args Co-authored-by: MarcoFalke <falke.marco@gmail.com>
53 lines
2.0 KiB
Python
53 lines
2.0 KiB
Python
#!/usr/bin/env python3
|
|
# Copyright (c) 2015 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
'''
|
|
This checks if all command line args are documented.
|
|
Return value is 0 to indicate no error.
|
|
|
|
Author: @MarcoFalke
|
|
'''
|
|
|
|
from subprocess import check_output
|
|
import re
|
|
import sys
|
|
|
|
FOLDER_GREP = 'src'
|
|
FOLDER_TEST = 'src/test/'
|
|
REGEX_ARG = '(?:ForceSet|SoftSet|Get|Is)(?:Bool)?Args?(?:Set)?\("(-[^"]+)"'
|
|
REGEX_DOC = 'AddArg\("(-[^"=]+?)(?:=|")'
|
|
CMD_ROOT_DIR = '`git rev-parse --show-toplevel`/{}'.format(FOLDER_GREP)
|
|
CMD_GREP_ARGS = r"git grep --perl-regexp '{}' -- {} ':(exclude){}'".format(REGEX_ARG, CMD_ROOT_DIR, FOLDER_TEST)
|
|
CMD_GREP_DOCS = r"git grep --perl-regexp '{}' {}".format(REGEX_DOC, CMD_ROOT_DIR)
|
|
# list unsupported, deprecated and duplicate args as they need no documentation
|
|
SET_DOC_OPTIONAL = set(['-h', '-help', '-dbcrashratio', '-forcecompactdb'])
|
|
|
|
|
|
def main():
|
|
if sys.version_info >= (3, 6):
|
|
used = check_output(CMD_GREP_ARGS, shell=True, universal_newlines=True, encoding='utf8')
|
|
docd = check_output(CMD_GREP_DOCS, shell=True, universal_newlines=True, encoding='utf8')
|
|
else:
|
|
used = check_output(CMD_GREP_ARGS, shell=True).decode('utf8').strip()
|
|
docd = check_output(CMD_GREP_DOCS, shell=True).decode('utf8').strip()
|
|
|
|
args_used = set(re.findall(re.compile(REGEX_ARG), used))
|
|
args_docd = set(re.findall(re.compile(REGEX_DOC), docd)).union(SET_DOC_OPTIONAL)
|
|
args_need_doc = args_used.difference(args_docd)
|
|
args_unknown = args_docd.difference(args_used)
|
|
|
|
print("Args used : {}".format(len(args_used)))
|
|
print("Args documented : {}".format(len(args_docd)))
|
|
print("Args undocumented: {}".format(len(args_need_doc)))
|
|
print(args_need_doc)
|
|
print("Args unknown : {}".format(len(args_unknown)))
|
|
print(args_unknown)
|
|
|
|
sys.exit(len(args_need_doc))
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|