mirror of
https://github.com/dashpay/dash.git
synced 2024-12-28 13:32:47 +01:00
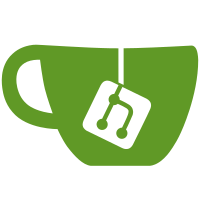
1f668b6
Expose if CScheduler is being serviced, assert its not in EmptyQueue (Matt Corallo)3192975
Flush CValidationInterface callbacks prior to destruction (Matt Corallo)08096bb
Support more than one CScheduler thread for serial clients (Matt Corallo)2fbf2db
Add default arg to CScheduler to schedule() a callback now (Matt Corallo)cda1429
Give CMainSignals a reference to the global scheduler (Matt Corallo)3a19fed
Make ValidationInterface signals-type-agnostic (Matt Corallo)ff6a834
Use TestingSetup to DRY qt rpcnestedtests (Matt Corallo) Tree-SHA512: fab91e34e30b080ed4d0a6d8c1214910e383c45440676e37be61d0bde6ae98d61e8903d22b846e95ba4e73a6ce788798350266feba246d8a2ab357e8523e4ac5
133 lines
4.2 KiB
C++
133 lines
4.2 KiB
C++
// Copyright (c) 2015 The Bitcoin Core developers
|
|
// Copyright (c) 2014-2019 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_TEST_TEST_DASH_H
|
|
#define BITCOIN_TEST_TEST_DASH_H
|
|
|
|
#include "chainparamsbase.h"
|
|
#include "fs.h"
|
|
#include "key.h"
|
|
#include "pubkey.h"
|
|
#include "random.h"
|
|
#include "scheduler.h"
|
|
#include "txdb.h"
|
|
#include "txmempool.h"
|
|
|
|
#include <boost/thread.hpp>
|
|
|
|
extern uint256 insecure_rand_seed;
|
|
extern FastRandomContext insecure_rand_ctx;
|
|
|
|
static inline void SeedInsecureRand(bool fDeterministic = false)
|
|
{
|
|
if (fDeterministic) {
|
|
insecure_rand_seed = uint256();
|
|
} else {
|
|
insecure_rand_seed = GetRandHash();
|
|
}
|
|
insecure_rand_ctx = FastRandomContext(insecure_rand_seed);
|
|
}
|
|
|
|
static inline uint32_t InsecureRand32() { return insecure_rand_ctx.rand32(); }
|
|
static inline uint256 InsecureRand256() { return insecure_rand_ctx.rand256(); }
|
|
static inline uint64_t InsecureRandBits(int bits) { return insecure_rand_ctx.randbits(bits); }
|
|
static inline uint64_t InsecureRandRange(uint64_t range) { return insecure_rand_ctx.randrange(range); }
|
|
static inline bool InsecureRandBool() { return insecure_rand_ctx.randbool(); }
|
|
|
|
/** Basic testing setup.
|
|
* This just configures logging and chain parameters.
|
|
*/
|
|
struct BasicTestingSetup {
|
|
ECCVerifyHandle globalVerifyHandle;
|
|
|
|
BasicTestingSetup(const std::string& chainName = CBaseChainParams::MAIN);
|
|
~BasicTestingSetup();
|
|
};
|
|
|
|
/** Testing setup that configures a complete environment.
|
|
* Included are data directory, coins database, script check threads setup.
|
|
*/
|
|
class CConnman;
|
|
struct TestingSetup: public BasicTestingSetup {
|
|
CCoinsViewDB *pcoinsdbview;
|
|
fs::path pathTemp;
|
|
boost::thread_group threadGroup;
|
|
CConnman* connman;
|
|
CScheduler scheduler;
|
|
|
|
TestingSetup(const std::string& chainName = CBaseChainParams::MAIN);
|
|
~TestingSetup();
|
|
};
|
|
|
|
class CBlock;
|
|
struct CMutableTransaction;
|
|
class CScript;
|
|
|
|
struct TestChainSetup : public TestingSetup
|
|
{
|
|
TestChainSetup(int blockCount);
|
|
~TestChainSetup();
|
|
|
|
// Create a new block with just given transactions, coinbase paying to
|
|
// scriptPubKey, and try to add it to the current chain.
|
|
CBlock CreateAndProcessBlock(const std::vector<CMutableTransaction>& txns,
|
|
const CScript& scriptPubKey);
|
|
CBlock CreateAndProcessBlock(const std::vector<CMutableTransaction>& txns,
|
|
const CKey& scriptKey);
|
|
CBlock CreateBlock(const std::vector<CMutableTransaction>& txns,
|
|
const CScript& scriptPubKey);
|
|
CBlock CreateBlock(const std::vector<CMutableTransaction>& txns,
|
|
const CKey& scriptKey);
|
|
|
|
std::vector<CTransaction> coinbaseTxns; // For convenience, coinbase transactions
|
|
CKey coinbaseKey; // private/public key needed to spend coinbase transactions
|
|
};
|
|
|
|
//
|
|
// Testing fixture that pre-creates a
|
|
// 100-block REGTEST-mode block chain
|
|
//
|
|
struct TestChain100Setup : public TestChainSetup {
|
|
TestChain100Setup() : TestChainSetup(100) {}
|
|
};
|
|
|
|
struct TestChainDIP3Setup : public TestChainSetup
|
|
{
|
|
TestChainDIP3Setup() : TestChainSetup(431) {}
|
|
};
|
|
|
|
struct TestChainDIP3BeforeActivationSetup : public TestChainSetup
|
|
{
|
|
TestChainDIP3BeforeActivationSetup() : TestChainSetup(430) {}
|
|
};
|
|
|
|
class CTxMemPoolEntry;
|
|
|
|
struct TestMemPoolEntryHelper
|
|
{
|
|
// Default values
|
|
CAmount nFee;
|
|
int64_t nTime;
|
|
unsigned int nHeight;
|
|
bool spendsCoinbase;
|
|
unsigned int sigOpCount;
|
|
LockPoints lp;
|
|
|
|
TestMemPoolEntryHelper() :
|
|
nFee(0), nTime(0), nHeight(1),
|
|
spendsCoinbase(false), sigOpCount(4) { }
|
|
|
|
CTxMemPoolEntry FromTx(const CMutableTransaction &tx);
|
|
CTxMemPoolEntry FromTx(const CTransaction &tx);
|
|
|
|
// Change the default value
|
|
TestMemPoolEntryHelper &Fee(CAmount _fee) { nFee = _fee; return *this; }
|
|
TestMemPoolEntryHelper &Time(int64_t _time) { nTime = _time; return *this; }
|
|
TestMemPoolEntryHelper &Height(unsigned int _height) { nHeight = _height; return *this; }
|
|
TestMemPoolEntryHelper &SpendsCoinbase(bool _flag) { spendsCoinbase = _flag; return *this; }
|
|
TestMemPoolEntryHelper &SigOps(unsigned int _sigops) { sigOpCount = _sigops; return *this; }
|
|
};
|
|
#endif
|