mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
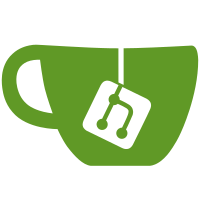
## Issue being fixed or feature implemented Some headers include other heavy headers, such as `logging.h`, `tinyformat.h`, `iostream`. These headers are heavy and increase compilation time on scale of whole project drastically because can be used in many other headers. ## What was done? Moved many heavy includes from headers to cpp files to optimize compilation time. In some places added forward declarations if it is reasonable. As side effect removed 2 circular dependencies: ``` "llmq/debug -> llmq/dkgsessionhandler -> llmq/debug" "llmq/debug -> llmq/dkgsessionhandler -> llmq/dkgsession -> llmq/debug" ``` ## How Has This Been Tested? Run build 2 times before refactoring and after refactoring: `make clean && sleep 10s; time make -j18` Before refactoring: ``` real 5m37,826s user 77m12,075s sys 6m20,547s real 5m32,626s user 76m51,143s sys 6m24,511s ``` After refactoring: ``` real 5m18,509s user 73m32,133s sys 6m21,590s real 5m14,466s user 73m20,942s sys 6m17,868s ``` ~5% of improvement for compilation time. That's not huge, but that's worth to get merged There're several more refactorings TODO but better to do them later by backports: - bitcoin/bitcoin#27636 - bitcoin/bitcoin#26286 - bitcoin/bitcoin#27238 - and maybe this one: bitcoin/bitcoin#28200 ## Breaking Changes N/A ## Checklist: - [x] I have performed a self-review of my own code - [ ] I have commented my code, particularly in hard-to-understand areas - [ ] I have added or updated relevant unit/integration/functional/e2e tests - [ ] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone
44 lines
1.6 KiB
C++
44 lines
1.6 KiB
C++
///////////////////////////////////////////////////////////////////////////////
|
|
//
|
|
// Copyright (c) 2023 The Dash Core developers
|
|
// Copyright (c) 2015 Microsoft Corporation. All rights reserved.
|
|
//
|
|
// This code is licensed under the MIT License (MIT).
|
|
//
|
|
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
// THE SOFTWARE.
|
|
//
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
|
|
#include <gsl/assert.h>
|
|
#include <logging.h>
|
|
|
|
#include <sstream>
|
|
namespace gsl
|
|
{
|
|
namespace details
|
|
{
|
|
[[noreturn]] void terminate(nostd::source_location loc) noexcept
|
|
{
|
|
#if defined(GSL_MSVC_USE_STL_NOEXCEPTION_WORKAROUND)
|
|
(*gsl::details::get_terminate_handler())();
|
|
#else
|
|
std::ostringstream s;
|
|
s << "ERROR: error detected null not_null detected at " << loc.file_name() << ":" << loc.line() << ":"
|
|
<< loc.column() << ":" << loc.function_name()
|
|
<< std::endl;
|
|
std::cerr << s.str() << std::flush;
|
|
LogPrintf("%s", s.str()); /* Continued */
|
|
std::terminate();
|
|
#endif // defined(GSL_MSVC_USE_STL_NOEXCEPTION_WORKAROUND)
|
|
}
|
|
|
|
} // namespace details
|
|
} // namespace gsl
|
|
|