mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
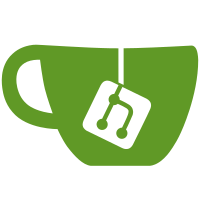
## Issue being fixed or feature implemented Many objects created and functions called by passing `const std::unique_ptr<Obj>& obj` instead directly passing `Obj& obj` In some cases it is indeed needed, but in most cases it is just extra complexity that is better to avoid. Motivation: - providing reference to object instead `unique_ptr` is giving warranty that there's no `nullptr` and no need to keep it in mind - value inside unique_ptr by reference can be changed externally and instead `nullptr` it can turn to real object later (or in opposite) - code is shorter but cleaner Based on that this refactoring is useful as it reduces mental load when reading or writing code. `std::unique` should be used ONLY for owning object, but not for passing it everywhere. ## What was done? Replaced most of usages `std::unique_ptr<Obj>& obj` to `Obj& obj`. Btw, in several cases implementation assumes that object can be nullptr and replacement to reference is not possible. Even using raw pointer is not possible, because the empty std::unique_ptr can be initialized later somewhere in code. For example, in `src/init.cpp` there's called `PeerManager::make` and pass unique_ptr to the `node.llmq_ctx` that would be initialized way later. That is out of scope this PR. List of cases, where reference to `std::unique_ptr` stayed as they are: - `std::unique_ptr<LLMQContext>& llmq_ctx` in `PeerManagerImpl`, `PeerManager` and `CDSNotificationInterface` - `std::unique_ptr<CDeterministicMNManager>& dmnman` in `CDSNotificationInterface` Also `CChainState` have 3 references to `unique_ptr` that can't be replaced too: - `std::unique_ptr<llmq::CChainLocksHandler>& m_clhandler;` - `std::unique_ptr<llmq::CInstantSendManager>& m_isman;` - `std::unique_ptr<llmq::CQuorumBlockProcessor>& m_quorum_block_processor;` ## How Has This Been Tested? Run unit/functional tests. ## Breaking Changes No breaking changes, all of these changes - are internal APIs for Dash Core developers only. ## Checklist: - [x] I have performed a self-review of my own code - [x] I have commented my code, particularly in hard-to-understand areas - [x] I have added or updated relevant unit/integration/functional/e2e tests - [x] I have made corresponding changes to the documentation - [x] I have assigned this pull request to a milestone --------- Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
51 lines
2.3 KiB
C++
51 lines
2.3 KiB
C++
// Copyright (c) 2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_DSNOTIFICATIONINTERFACE_H
|
|
#define BITCOIN_DSNOTIFICATIONINTERFACE_H
|
|
|
|
#include <validationinterface.h>
|
|
|
|
class CConnman;
|
|
class CDeterministicMNManager;
|
|
class CGovernanceManager;
|
|
class CMasternodeSync;
|
|
struct LLMQContext;
|
|
|
|
class CDSNotificationInterface : public CValidationInterface
|
|
{
|
|
public:
|
|
explicit CDSNotificationInterface(CConnman& _connman,
|
|
CMasternodeSync& _mn_sync, const std::unique_ptr<CDeterministicMNManager>& _dmnman,
|
|
CGovernanceManager& _govman, const std::unique_ptr<LLMQContext>& _llmq_ctx);
|
|
virtual ~CDSNotificationInterface() = default;
|
|
|
|
// a small helper to initialize current block height in sub-modules on startup
|
|
void InitializeCurrentBlockTip();
|
|
|
|
protected:
|
|
// CValidationInterface
|
|
void AcceptedBlockHeader(const CBlockIndex *pindexNew) override;
|
|
void NotifyHeaderTip(const CBlockIndex *pindexNew, bool fInitialDownload) override;
|
|
void SynchronousUpdatedBlockTip(const CBlockIndex *pindexNew, const CBlockIndex *pindexFork, bool fInitialDownload) override;
|
|
void UpdatedBlockTip(const CBlockIndex *pindexNew, const CBlockIndex *pindexFork, bool fInitialDownload) override;
|
|
void TransactionAddedToMempool(const CTransactionRef& tx, int64_t nAcceptTime) override;
|
|
void TransactionRemovedFromMempool(const CTransactionRef& ptx, MemPoolRemovalReason reason) override;
|
|
void BlockConnected(const std::shared_ptr<const CBlock>& pblock, const CBlockIndex* pindex) override;
|
|
void BlockDisconnected(const std::shared_ptr<const CBlock>& pblock, const CBlockIndex* pindexDisconnected) override;
|
|
void NotifyMasternodeListChanged(bool undo, const CDeterministicMNList& oldMNList, const CDeterministicMNListDiff& diff, CConnman& connman) override;
|
|
void NotifyChainLock(const CBlockIndex* pindex, const std::shared_ptr<const llmq::CChainLockSig>& clsig) override;
|
|
|
|
private:
|
|
CConnman& connman;
|
|
|
|
CMasternodeSync& m_mn_sync;
|
|
const std::unique_ptr<CDeterministicMNManager>& dmnman;
|
|
CGovernanceManager& govman;
|
|
|
|
const std::unique_ptr<LLMQContext>& llmq_ctx;
|
|
};
|
|
|
|
#endif // BITCOIN_DSNOTIFICATIONINTERFACE_H
|