mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
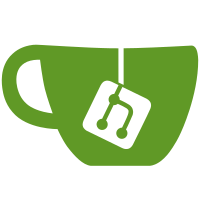
* merge 15638: Move CheckTransaction from lib_server to lib_consensus * merge 15638: Move policy settings to new src/policy/settings unit * merge 15638: Move rpc utility methods to rpc/util * merge 15638: Move rpc rawtransaction util functions to rpc/rawtransaction_util.cpp * merge 15638: Move several units into common libraries * merge 15638: Move wallet load functions to wallet/load unit * merge 15638: Document src subdirectories and different libraries * [build] Add several util units (cleanup) * build: resolve missing declarations by re-specifying headers
73 lines
2.4 KiB
C++
73 lines
2.4 KiB
C++
// Copyright (c) 2014-2020 The Dash Core developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <key_io.h>
|
|
#include <hash.h>
|
|
#include <util/validation.h> // For strMessageMagic
|
|
#include <messagesigner.h>
|
|
#include <tinyformat.h>
|
|
#include <util/strencodings.h>
|
|
|
|
bool CMessageSigner::GetKeysFromSecret(const std::string& strSecret, CKey& keyRet, CPubKey& pubkeyRet)
|
|
{
|
|
keyRet = DecodeSecret(strSecret);
|
|
if (!keyRet.IsValid()) {
|
|
return false;
|
|
}
|
|
pubkeyRet = keyRet.GetPubKey();
|
|
|
|
return true;
|
|
}
|
|
|
|
bool CMessageSigner::SignMessage(const std::string& strMessage, std::vector<unsigned char>& vchSigRet, const CKey& key)
|
|
{
|
|
CHashWriter ss(SER_GETHASH, 0);
|
|
ss << strMessageMagic;
|
|
ss << strMessage;
|
|
|
|
return CHashSigner::SignHash(ss.GetHash(), key, vchSigRet);
|
|
}
|
|
|
|
bool CMessageSigner::VerifyMessage(const CPubKey& pubkey, const std::vector<unsigned char>& vchSig, const std::string& strMessage, std::string& strErrorRet)
|
|
{
|
|
return VerifyMessage(pubkey.GetID(), vchSig, strMessage, strErrorRet);
|
|
}
|
|
|
|
bool CMessageSigner::VerifyMessage(const CKeyID& keyID, const std::vector<unsigned char>& vchSig, const std::string& strMessage, std::string& strErrorRet)
|
|
{
|
|
CHashWriter ss(SER_GETHASH, 0);
|
|
ss << strMessageMagic;
|
|
ss << strMessage;
|
|
|
|
return CHashSigner::VerifyHash(ss.GetHash(), keyID, vchSig, strErrorRet);
|
|
}
|
|
|
|
bool CHashSigner::SignHash(const uint256& hash, const CKey& key, std::vector<unsigned char>& vchSigRet)
|
|
{
|
|
return key.SignCompact(hash, vchSigRet);
|
|
}
|
|
|
|
bool CHashSigner::VerifyHash(const uint256& hash, const CPubKey& pubkey, const std::vector<unsigned char>& vchSig, std::string& strErrorRet)
|
|
{
|
|
return VerifyHash(hash, pubkey.GetID(), vchSig, strErrorRet);
|
|
}
|
|
|
|
bool CHashSigner::VerifyHash(const uint256& hash, const CKeyID& keyID, const std::vector<unsigned char>& vchSig, std::string& strErrorRet)
|
|
{
|
|
CPubKey pubkeyFromSig;
|
|
if(!pubkeyFromSig.RecoverCompact(hash, vchSig)) {
|
|
strErrorRet = "Error recovering public key.";
|
|
return false;
|
|
}
|
|
|
|
if(pubkeyFromSig.GetID() != keyID) {
|
|
strErrorRet = strprintf("Keys don't match: pubkey=%s, pubkeyFromSig=%s, hash=%s, vchSig=%s",
|
|
keyID.ToString(), pubkeyFromSig.GetID().ToString(), hash.ToString(),
|
|
EncodeBase64(vchSig));
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|