mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
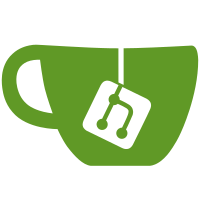
64c0800
Use logging in individual tests (John Newbery)38ad281
Use logging in test_framework/comptool.py (John Newbery)ff19073
Use logging in test_framework/blockstore.py (John Newbery)2a9c7c7
Use logging in test_framework/util.py (John Newbery)b0dec4a
Remove manual debug settings in qa tests. (John Newbery)af1363c
Always enable debug log and microsecond logging for test nodes. (John Newbery)6d0e325
Use logging in mininode.py (John Newbery)553a976
Add logging to p2p-segwit.py (John Newbery)0e6d23d
Add logging to test_framework.py (John Newbery) Tree-SHA512: 42ee2acbf444ec32d796f930f9f6e272da03c75e93d974a126d4ea9b2dbaa77cc57ab5e63ce3fd33d609049d884eb8d9f65272c08922d10f8db69d4a60ad05a3
82 lines
2.6 KiB
Python
Executable File
82 lines
2.6 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2017 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test the listsincelast RPC."""
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal
|
|
|
|
class ListSinceBlockTest (BitcoinTestFramework):
|
|
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 4
|
|
|
|
def run_test (self):
|
|
'''
|
|
`listsinceblock` did not behave correctly when handed a block that was
|
|
no longer in the main chain:
|
|
|
|
ab0
|
|
/ \
|
|
aa1 [tx0] bb1
|
|
| |
|
|
aa2 bb2
|
|
| |
|
|
aa3 bb3
|
|
|
|
|
bb4
|
|
|
|
Consider a client that has only seen block `aa3` above. It asks the node
|
|
to `listsinceblock aa3`. But at some point prior the main chain switched
|
|
to the bb chain.
|
|
|
|
Previously: listsinceblock would find height=4 for block aa3 and compare
|
|
this to height=5 for the tip of the chain (bb4). It would then return
|
|
results restricted to bb3-bb4.
|
|
|
|
Now: listsinceblock finds the fork at ab0 and returns results in the
|
|
range bb1-bb4.
|
|
|
|
This test only checks that [tx0] is present.
|
|
'''
|
|
|
|
assert_equal(self.is_network_split, False)
|
|
self.nodes[2].generate(101)
|
|
self.sync_all()
|
|
|
|
assert_equal(self.nodes[0].getbalance(), 0)
|
|
assert_equal(self.nodes[1].getbalance(), 0)
|
|
assert_equal(self.nodes[2].getbalance(), 500)
|
|
assert_equal(self.nodes[3].getbalance(), 0)
|
|
|
|
# Split network into two
|
|
self.split_network()
|
|
assert_equal(self.is_network_split, True)
|
|
|
|
# send to nodes[0] from nodes[2]
|
|
senttx = self.nodes[2].sendtoaddress(self.nodes[0].getnewaddress(), 1)
|
|
|
|
# generate on both sides
|
|
lastblockhash = self.nodes[1].generate(6)[5]
|
|
self.nodes[2].generate(7)
|
|
self.log.info('lastblockhash=%s' % (lastblockhash))
|
|
|
|
self.sync_all()
|
|
|
|
self.join_network()
|
|
|
|
# listsinceblock(lastblockhash) should now include tx, as seen from nodes[0]
|
|
lsbres = self.nodes[0].listsinceblock(lastblockhash)
|
|
found = False
|
|
for tx in lsbres['transactions']:
|
|
if tx['txid'] == senttx:
|
|
found = True
|
|
break
|
|
assert_equal(found, True)
|
|
|
|
if __name__ == '__main__':
|
|
ListSinceBlockTest().main()
|