mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
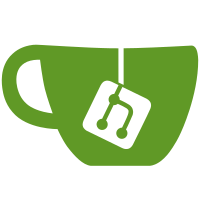
* Don't rely on UTXO set in CheckCanLock The UTXO set only works for TXs in the mempool and won't work when we try to retroactively lock unlocked TXs from blocks. This is safe as ProcessTx is only called when a TX was accepted into the mempool or connected in a block, which means that all input checks were good. * Rename RetryLockMempoolTxs to RetryLockTxs and let it retry connected TXs * Instead of manually calling ProcessTx, let SyncTransaction handle all cases SyncTransaction is called from AcceptToMemoryPool and when transactions got connected in a block. So this is the time we want to run TXs through ProcessTx. This also enables retroactive signing of TXs that were unknown before a new block appeared. * Test retroactive signing and safe TXs in LLMQ ChainLocks tests * Also test for retroactive signing of chained TXs * Honor lockedParentTx when looking for TXs to retry signing * Stop scanning for TXs to retry after a depth of 6 * Generate 6 block to avoid retroactive signing overloading Travis * Avoid retroactive signing * Don't rely on NewPoWValidBlock and use SyncTransaction to build blockTxs NewPoWValidBlock is not guaranteed to be called when blocks come in fast. When a block is accepted in AcceptBlock, NewPoWValidBlock is only called when the new block is a successor of the currently active tip. This is not the case when after the first block a second block is accepted immediately as the first block is not connected yet. This might be a bug actually in the handling of NewPoWValidBlock, so we might need to check/fix this later, but currently I prefer to not touch that part. Instead, we now use SyncTransaction to gather TXs for blockTxs. This works because SyncTransaction is called for all transactions in a freshly connected block in one go. The call also happens before UpdatedBlockTip is called, so it's fine with the existing logic. * Use tx.IsCoinBase() instead of checking index 0 Also check for empty vin.
94 lines
3.5 KiB
Python
Executable File
94 lines
3.5 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2018 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.mininode import *
|
|
from test_framework.test_framework import DashTestFramework
|
|
from test_framework.util import *
|
|
from time import *
|
|
|
|
'''
|
|
InstantSendTest -- test InstantSend functionality (prevent doublespend for unconfirmed transactions)
|
|
'''
|
|
|
|
class InstantSendTest(DashTestFramework):
|
|
def __init__(self):
|
|
super().__init__(9, 5, [], fast_dip3_enforcement=True)
|
|
# set sender, receiver, isolated nodes
|
|
self.isolated_idx = 1
|
|
self.receiver_idx = 2
|
|
self.sender_idx = 3
|
|
|
|
def run_test(self):
|
|
self.nodes[0].spork("SPORK_17_QUORUM_DKG_ENABLED", 0)
|
|
self.wait_for_sporks_same()
|
|
self.mine_quorum()
|
|
|
|
self.log.info("Test old InstantSend")
|
|
self.test_doublespend()
|
|
|
|
# Generate 6 block to avoid retroactive signing overloading Travis
|
|
self.nodes[0].generate(6)
|
|
sync_blocks(self.nodes)
|
|
|
|
self.nodes[0].spork("SPORK_20_INSTANTSEND_LLMQ_BASED", 0)
|
|
self.wait_for_sporks_same()
|
|
|
|
self.log.info("Test new InstantSend")
|
|
self.test_doublespend()
|
|
|
|
def test_doublespend(self, new_is = False):
|
|
sender = self.nodes[self.sender_idx]
|
|
receiver = self.nodes[self.receiver_idx]
|
|
isolated = self.nodes[self.isolated_idx]
|
|
# feed the sender with some balance
|
|
sender_addr = sender.getnewaddress()
|
|
self.nodes[0].sendtoaddress(sender_addr, 1)
|
|
# make sender funds mature for InstantSend
|
|
for i in range(0, 2):
|
|
set_mocktime(get_mocktime() + 1)
|
|
set_node_times(self.nodes, get_mocktime())
|
|
self.nodes[0].generate(1)
|
|
self.sync_all()
|
|
|
|
# create doublepending transaction, but don't relay it
|
|
dblspnd_tx = self.create_raw_tx(sender, isolated, 0.5, 1, 100)
|
|
# stop one node to isolate it from network
|
|
isolated.setnetworkactive(False)
|
|
# instantsend to receiver
|
|
receiver_addr = receiver.getnewaddress()
|
|
is_id = sender.instantsendtoaddress(receiver_addr, 0.9)
|
|
for node in self.nodes:
|
|
if node is not isolated:
|
|
self.wait_for_instantlock(is_id, node)
|
|
# send doublespend transaction to isolated node
|
|
isolated.sendrawtransaction(dblspnd_tx['hex'])
|
|
# generate block on isolated node with doublespend transaction
|
|
set_mocktime(get_mocktime() + 1)
|
|
set_node_times(self.nodes, get_mocktime())
|
|
isolated.generate(1)
|
|
wrong_block = isolated.getbestblockhash()
|
|
# connect isolated block to network
|
|
isolated.setnetworkactive(True)
|
|
for i in range(0, self.isolated_idx):
|
|
connect_nodes(self.nodes[i], self.isolated_idx)
|
|
# check doublespend block is rejected by other nodes
|
|
timeout = 10
|
|
for i in range(0, self.num_nodes):
|
|
if i == self.isolated_idx:
|
|
continue
|
|
res = self.nodes[i].waitforblock(wrong_block, timeout)
|
|
assert (res['hash'] != wrong_block)
|
|
# wait for long time only for first node
|
|
timeout = 1
|
|
# mine more blocks
|
|
# TODO: mine these blocks on an isolated node
|
|
set_mocktime(get_mocktime() + 1)
|
|
set_node_times(self.nodes, get_mocktime())
|
|
self.nodes[0].generate(2)
|
|
self.sync_all()
|
|
|
|
if __name__ == '__main__':
|
|
InstantSendTest().main()
|